Spring Boot is a powerful framework built on top of the Spring framework. Spring Boot allowed programmers to focus mainly on the business logic without worrying about the external configuration and setting up the environment resources like server setup.
Configuration of SpringBoot
Configuration is the feature of the framework that gives the programmer ease in configuring the application in minimal work and shifts the focus more to the application’s business logic.
In this article, we’ll look into spring boot configuration features and how they help achieve the Convention-over-Configuration (CoC) Principle.
Primary Terminologies
There are some well-known terms often faced while using Springboot as mentioned below:
1. Java Bean
A Java Bean is a terminology defined to refer to the Java objects whose creation, management, and removal when not required anymore (basically the full lifecycle) is managed by the Spring Container.
2. IoC Principle
IoC is an acronym for “Inversion Of Control“. IoC Principle suggests a structured approach for writing development code that is, shifting the creation & management control of Java Beans from the programmer to the framework. This approach allows the programmer to focus more on business logic than deal with the overall lifecycle of trivial Java Beans.
- IoC is achieved by the help of Spring IoC Container whose purpose is to initialize Java Objects and manage dependencies and other components of our Spring Boot Application.
3. Dependency Injection
Dependency Injection (DI) is a core feature of the Spring Boot framework that facilitates the creation & management of Java Beans much organized and easy to implement in the Spring Boot Application.
The Dependencies in the Spring Boot Application are injected through an autonomous procedure performed by the Spring IoC Container at the start of the Spring Boot Application.
4. Annotation
Annotations are the most basic components of the Spring framework that play a crucial role in configuring various aspects of our Spring Boot Application. From creation, management, and management of the lifecycle of certain objects offering a versatile functionality.
In simple words, annotations are a tag that can be put on top of a class, object, or method signifying a specific functionality for that component.
CoC Principle
CoC Principle – Convention Over Configuration is a software development principle that emphasizes boosting developer productivity by minimizing the amount of externalized configuration required to build an application.
This principle work by making certain assumption that can be re-configured by the developer if required. Spring Boot heavily embraces this approach by provisioning support for certain features following are some of them:
- Auto-Configuration
- Starter File
- Actuator
- Externalized Configuration
Throughout this article, we’ll learn about various technologies that power our Spring Boot application and embrace this principle.
1. Starter File
The starter file is the initial step in configuring a Spring Boot application. You can create your starter file at Spring Initializr specifying the dependencies the version of Java & Spring you want to work with, and the build tool you want to use (maven, gradle, or groove). Then you can download the started file in a zip file format and open it in your favorite IDE. It looks something like this :
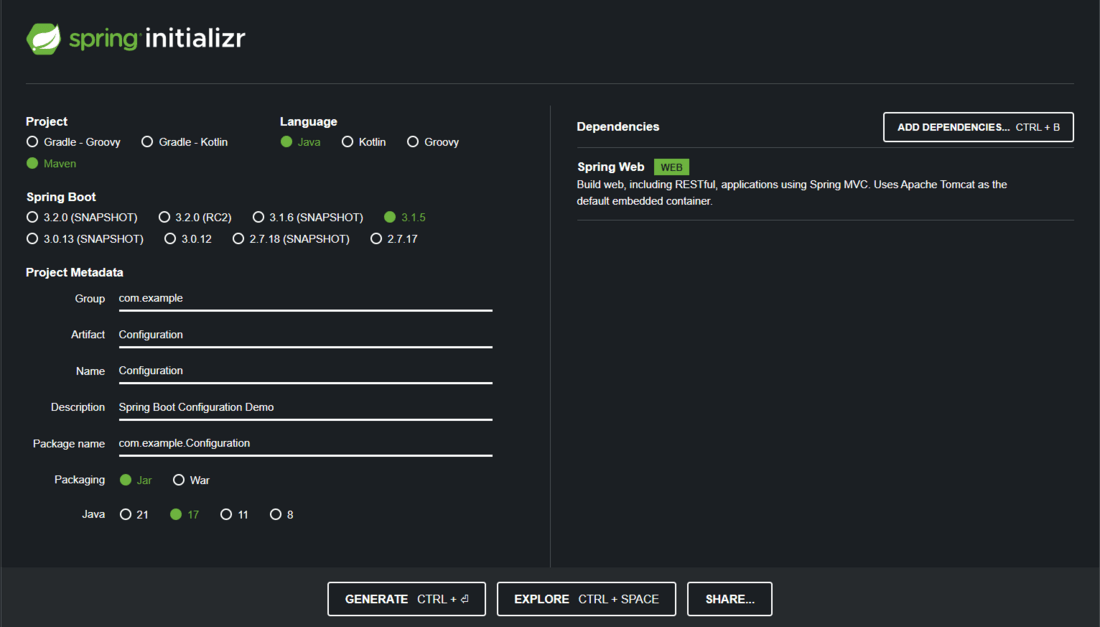
Starter File
2. In-Built Server Support
Spring Boot has in-built server support allowing us to embed web servers. This feature allows us to create a stand-alone application, an executable JAR File which is self-contained. By default, Spring Boot uses Tomcat as its default server.
However, if you want to change that you can define an external dependency for the server you want to use in your POM File.
Configuring the server
You can further configure the embedded server, and there are many ways to do this, we’ll see the example of using an external properties file :
# Set Server Port
server.port = 8080
# Set Server Context Path
server.servlet.context-path=/myapp
3. Several ways to configure Spring Boot Application
3.1 Externalized Configuration
Spring boot allows us to configure external database connection (datasource), specific instructions, server configuration, and settings in an external file – either a properties file or yaml file. Both have extensions as – .properties and .yaml
Auto configuration leverages this external file to bind some properties with the bean fields and accommodate them to work as per our specific use case.
application.properties
spring.application.name= ConfigurationApp
spring.datasource.username = root
spring.datasource.password = root
spring.datasource.url = jdbc:mysql://127.0.0.1:3306/ConfigDemoDB?autoreconnect=true
server.port=8080
# Custom properties
custom.property1=value1
custom.property2=value2
application.yaml
spring:
application:
name: ConfigurationApp
datasource:
username: root
password: root
url: jdbc:mysql://127.0.0.1:3306/ConfigDemoDB?autoreconnect=true
server:
port: 8080
# Custom properties
custom:
property1: value1
property2: value2
3.2 XML Based Configuration
We can configure our spring boot application using an XML File. XML File contains all the Java Bean definitions and the dependencies that are to be injected. Let’s understand by an example:-
Assume you have a Car & and Engine class as POJOs (Plain old Java objects) to carry data. From a real-world perspective, a car cannot function without an engine. So, here the engine is the dependency to be injected upon the creation of Car Bean. This dependency injection will be taken care of by the Spring IoC Container.
The XML based configuration would look something like this:-
XML
< bean id = "engine" class = "com.GeeksforGeeks.Engine" >
< property name = "type" value = "V-engine" />
</ bean >
< bean id = "car" class = "com.example.Car" >
< property name = "model" value = "luxury" />
< property name = "engine" ref = "engine" />
</ bean >
</ beans >
|
Explanation of above Code:
- <bean> This element is used to define the bean that will be managed by the Spring IoC Container
- id is the property assigned to the bean, and this bean will be referred to using this id in the configuration.
- The <property name> & <value> are key-value pairs that represent that this car has a model as – luxury
- <property name = “engine” ref = “engine”> defined the dependency that the engine bean has to be injected into the car bean.
Note: XML Configuration is consider legacy and rarely used nowadays.
3.3 Java Based Configuration
Java-based configuration is another modern way to configure our Spring Application, that utilises a specific Java class annotated with @Configuration indication that this class contains the configuration beans, and dependencies that are to be injected upon the launch of the Spring boot application.
Java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public Engine engine() {
Engine engine = new Engine();
engine.setType( "V-Engine" );
return engine;
}
@Bean
public Car car() {
Car car = new Car();
car.setModel( "luxury" );
car.setEngine(engine());
return car;
}
}
|
Explanation of above Code:
- The @Configuration annotation is annotated on top of a class which contains bean definition (basically methods that return an instance of a bean), and also the instruction on how to configure the beans and inject dependencies. This annotation lets the Spring framework know that the spring IoC container should process these beans during the initialization of Spring Context.
- @Bean is used to indicate that this is a Java Bean whose lifecycle will be managed by the Spring IoC Container.
- In the above code, created two beans – Car and Engine. Also injecting a dependency using a setEngine(engine()) Method manually.
This code can be optimised to further extents, using Annotation Based Configuration.
3.4 Annotation Based Configuration
Annotation Based Configuration is the most recent way to configure our spring boot application and it is preferred over other configuration types by most modern developers. It minimises the redundant code, improves readability, and promotes the CoC Principle.
1. Engine Class
Java
import org.springframework.stereotype.Component;
@Component
class Engine {
String type;
}
|
2. Car Class
Java
import org.springframework.stereotype.Component;
@Component
class Car {
String Model;
Engine engine;
@Autowired
public Car(Engine theEngine)
{
this .engine = theEngine;
}
}
|
Explanation of above Code:
- Here we’ve used the Spring Constructor injection to inject the Engine dependency in the Car Class. Spring Recommends using Constructor injection over other ways to inject dependencies as our first choice. You can learn more about setter injection and constructor injection here.
- @Component marks the class as eligible for spring component scanning and provides configuration as soon as the spring boot application launches.
- Other useful annotation in spring Annotation configuration includes : @Repository, @Service, etc.
4. Class Path Scanning
Spring boot employs a feature called classpath scanning (or spring context scanning) that scans through our current project’s directory structure systematically to initialize, inject dependencies and configure beans. This spring scanning offers the programmer a flexibility of only creating and annotating a bean without explicitly specifying to spring where to find it and how to look for it. It is a very productive feature that makes spring boot configuration way easier.
5. Actuator
Spring Boot Actuator is a subproject of Spring Boot, it provides useful insights and an overview of our application by defining a certain set of metrics such as monitoring, healthchecks, logging, and other application-related information. Endpoint examples include :
- localhost:8080/actuator/health
- localhost:8080/actuator/metrics
- localhost:8080/actuator/info
Note that every endpoint starts from the “actuator” keyword, signifying the same. We can include this sub-project into our application by including the following dependency into our POM File:-
XML
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-actuator</ artifactId >
</ dependency >
|
Advantages Spring Boot Configuration
- Flexibility: Spring Boot Configuration provides easily configurable components of the application to the developer.
- Rapid Development: Features like auto-configuation, and dependency management make it easier to develop the application in a fast and efficient manner, minimizing the explicit configuration.
- Large Community: Spring Boot has been powering large-scale enterprise level applications for so many years, hence it has rich community support and many errors, ambiguities & conflicts related solutions can be found easily on the internet.
Disadvantages Spring Boot Configuration
- Limited Flexibility in certain scenarios: While spring boot configuration embraces the CoC Principle approach, it may not amount to be useful in scenarios when we need a fine-grained control over our application.
- Versioning conflict: If one version of some dependency is not compatible with some other dependency, it might become quite confusing & complex to manage both.
- Opinionated Defaults: Spring boot default configuration may not align with every project’s goals. Developers must understand the requirements clearly and re-configure accordingly.
Conclusion
In conclusion, Spring Boot configuration techniques enhance developer productivity, employing easy configuration and mainly focus on the application’s business logic. Configuration can be done in many ways, there’s no black and whites. Its a matter of personal preferences and your project’s needs.
Spring also offers a very vast documentation support to understand everything in a concise manner.
- Starter file is the initial step of Spring Boot configuration that saves the developer from writing tedious XML configuration and doing initial setup.
- Spring Boot configuration provides developer with sensible defaults and automatic configurations that reducing the need to explicitly setup everything in place.
- Spring Boot configuration provides us with many annotations that allows the programmer to instruct the spring context configuration in small set of steps. Ex: @Configuration, @Autowired, @Bean etc.
Share your thoughts in the comments
Please Login to comment...