Solidity Global Variables
Last Updated :
08 Apr, 2023
Global variables in Solidity are predefined variables available in any function or contract. These variables reveal blockchain, contract, and transaction data. Some common global variables in Solidity:
Variable |
Type |
Description |
msg.sender |
address |
The address of the account that sent the current transaction. |
msg.value |
uint |
The amount of Ether sent with the current transaction. |
block.coinbase |
address |
The address of the miner who mined the current block. |
block.difficulty |
uint |
The difficulty of the current block. |
block.gaslimit |
uint |
The maximum amount of gas that can be used in the current block. |
block.number |
uint |
The number of the current block. |
block.timestamp |
uint |
The timestamp of the current block. |
now |
uint |
An alias for block.timestamp. |
tx.origin |
address |
The address of the account that originally created the transaction (i.e., the sender of the first transaction in the call chain). |
tx.gasprice |
uint |
The gas price (in Wei) of the current transaction. |
These variables are handy for getting blockchain and transaction information but use them carefully. Authentication and authorization should not utilize tx.origin since attackers may simply fake it. Use msg.sender instead.
- The contract cannot directly modify read-only global variables.
- They can make contract choices and execute logic. msg.sender can restrict contract functions to the owner.
- Global variables’ values vary based on context. In a transaction, msg.sender refers to the sender’s address, but outside of a transaction, it refers to the contract’s address (such as in a constructor).
Below is the Solidity program to implement global variables:
Solidity
pragma solidity ^0.8.0;
contract GlobalVariablesExample {
address public owner;
constructor()
{
owner = msg.sender;
}
function getOwner() public view returns (address)
{
return owner;
}
function isOwner(address _address) public view returns ( bool )
{
return _address == owner;
}
function sendEther(address payable _recipient) public payable
{
require(msg.sender == owner,
"Only the contract owner can send ether." );
_recipient.transfer(msg.value);
}
function getCurrentBlock() public view returns (uint,
uint, address)
{
return (block.number, block.timestamp, block.coinbase);
}
}
|
Explanation: This example defines the GlobalVariablesExample contract. The function Object() { [native code] } initializes the owner state variable to the contract’s deployment address using the msg.sender global variable.
The contract specifies global variable functions:
- GetOwner returns owner.
- IsOwner returns a boolean value if an address matches the owner.
- If msg.sender matches owner, the contract owner may transmit ether to a receiver using the sendEther method.
- The getCurrentBlock method uses global variables block.number, block.timestamp, and block.coinbase to retrieve block information.
- The sendEther method requires the contract owner to send ether. If the need statement is false, the transaction will be reversed with the error notice.
Global variables may be used in Solidity contracts to make choices and execute logic.
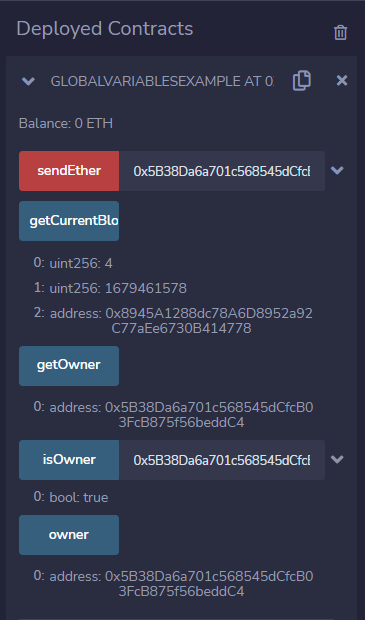
Â
Share your thoughts in the comments
Please Login to comment...