Skewed Binary Tree
Last Updated :
22 Jul, 2021
A skewed binary tree is a type of binary tree in which all the nodes have only either one child or no child.
Types of Skewed Binary trees
There are 2 special types of skewed tree:
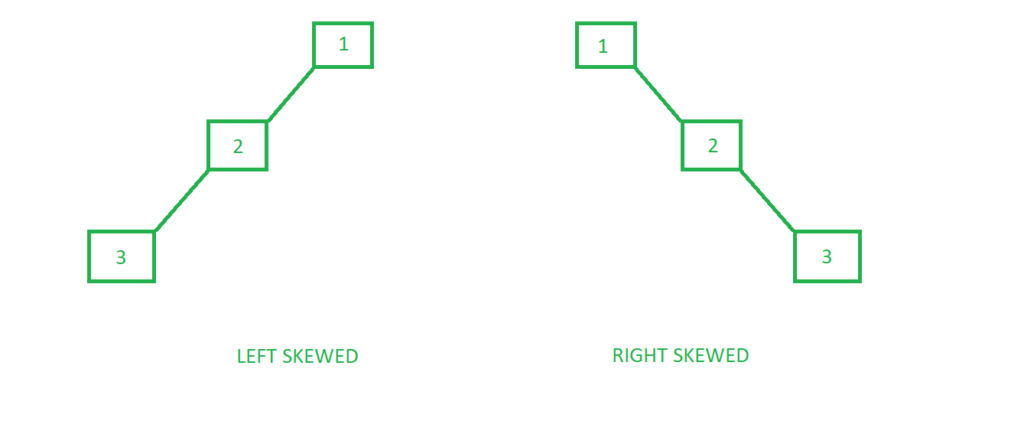
1. Left Skewed Binary Tree:
These are those skewed binary trees in which all the nodes are having a left child or no child at all. It is a left side dominated tree. All the right children remain as null.
Below is an example of a left-skewed tree:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int key;
struct Node *left, *right;
};
Node* newNode( int key)
{
Node* temp = new Node;
temp->key = key;
temp->left = temp->right = NULL;
return (temp);
}
int main()
{
Node* root = newNode(1);
root->left = newNode(2);
root->left->left = newNode(3);
return 0;
}
|
Java
import java.util.*;
class GFG
{
static class Node
{
int key;
Node left, right;
};
static Node newNode( int key)
{
Node temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
public static void main(String args[])
{
Node root = newNode( 1 );
root.left = newNode( 2 );
root.left.left = newNode( 3 );
}
}
|
Python3
class Node:
def __init__( self , key):
self .left = None
self .right = None
self .val = key
root = Node( 1 )
root.left = Node( 2 )
root.left.left = Node( 2 )
|
C#
using System;
class GFG
{
public class Node
{
public int key;
public Node left, right;
};
static Node newNode( int key)
{
Node temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
public static void Main()
{
Node root = newNode(1);
root.left = newNode(2);
root.left.left = newNode(3);
}
}
|
Javascript
<script>
class Node
{
constructor()
{
this .key=0;
this .left= this .right= null ;
}
}
function newNode(key)
{
let temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
let root = newNode(1);
root.left = newNode(2);
root.left.left = newNode(3);
</script>
|
2. Right Skewed Binary Tree:
These are those skewed binary trees in which all the nodes are having a right child or no child at all. It is a right side dominated tree. All the left children remain as null.
Below is an example of a right-skewed tree:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int key;
struct Node *left, *right;
};
Node* newNode( int key)
{
Node* temp = new Node;
temp->key = key;
temp->left = temp->right = NULL;
return (temp);
}
int main()
{
Node* root = newNode(1);
root->right = newNode(2);
root->right->right = newNode(3);
return 0;
}
|
Java
import java.util.*;
class GFG
{
static class Node
{
int key;
Node left, right;
};
static Node newNode( int key)
{
Node temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
public static void main(String args[])
{
Node root = newNode( 1 );
root.right = newNode( 2 );
root.right.right = newNode( 3 );
}
}
|
Python3
class Node:
def __init__( self , key):
self .left = None
self .right = None
self .val = key
root = Node( 1 )
root.right = Node( 2 )
root.right.right = Node( 3 )
|
C#
using System;
class GFG
{
public class Node
{
public int key;
public Node left, right;
};
static Node newNode( int key)
{
Node temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
public static void Main(String []args)
{
Node root = newNode(1);
root.right = newNode(2);
root.right.right = newNode(3);
}
}
|
Javascript
<script>
class Node
{
constructor()
{
this .key = 0;
this .left = this .right = null ;
}
}
function newNode(key)
{
let temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
let root = newNode(1);
root.right = newNode(2);
root.right.right = newNode(3);
</script>
|
Share your thoughts in the comments
Please Login to comment...