For all the Linux distributions, the shell script is like a magic wand that automates the process, saves users time, and increases productivity. This shall scripting tutorial will introduce you to the 25 plus shall scripting examples.
But before we move on to the topic of shell scripting examples, let’s understand shell script and the actual use cases of shell scripting.
What is Shell Script?
Well, the shell is a CLI (command line interpreter), which runs in a text window where users can manage and execute shell commands. On the other hand, the process of writing a set of commands to be executed on a Linux system A file that includes such instructions is called a bash script.
Uses of Shell Scripts
Below are some common uses of Shell Script:
- Task Automation – It can be used to automate repetitive tasks like regular backups and software installation tasks.
- Customization – One can use shell scripts to design its command line environment and easily perform its task as per needs.
- File Management – The shell scripts can also be used to manage and manipulate files and directories, such as moving, copying, renaming, or deleting files.
Shell Script Examples in Linux
1) What does the shebang (#!) at the beginning of a shell script indicate?
The shebang (#!) at the beginning of a script indicates the interpreter that should be used to execute the script. It tells the system which shell or interpreter should interpret the script’s commands.
For example: Suppose we have a script named myscript.sh written in the Bash shell:

shebang
In this example:
- The #!/bin/bash at the beginning of the script indicates that the script should be interpreted using the Bash shell.
- The echo commands are used to print messages to the terminal.
2) How do you run a shell script from the command line?
To run a shell script from the command line, we need to follow these steps:
- Make sure the script file has executable permissions using the chmod command:
chmod +x myscript.sh
- Execute the script using its filename:
./myscript.sh
Here you have to replace “myscrtipt.sh” with yors script name.
3) Write a shell script that prints “GeeksforGeeks” to the terminal.
Create a script name `myscript.sh` (we are using `vim` editor, you can choose any editor)
vim myscript.sh
#!/bin/bash
# This script prints “GeeksforGeeks” to the terminal
echo “GeeksforGeeks”
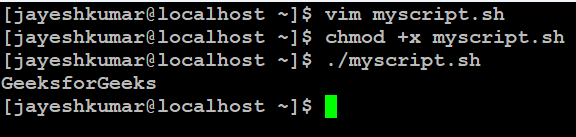
print name
We make our script executable by using `chmod +x` then execute with `./myscipt.sh` and get our desired output “GeeksforGeeks”.
4) Explain the purpose of the echo command in shell scripting.
The echo command is used to display text or variables on the terminal. It’s commonly used for printing messages, variable values, and generating program output.

echo command
In this example we have execute `echo` on terminal directely , as it works same inside shell script.
5) How can you assign a value to a variable in a shell script?
Variables are assigned values using the assignment operator =.
For example:
#!/bin/bash
# Assigning a value to a variable
name=”Jayesh”
age=21
echo $name $age
Explanation:
- The name variable is assigned the value “Jayesh”.
- The age variable is assigned the value 21.
- echo is used to print and `$name` `$age` is used to call the value stored in the variables.
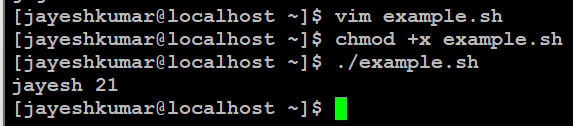
6) Write a shell script that takes a user’s name as input and greets them.
Create a script name `example.sh`.
#!/bin/bash
# Ask the user for their name
echo “What’s your name?”
read name
# Greet the user
echo “Hello, $name! Nice to meet you.”
Explanation:
- #!/bin/bash: This is the shebang line. It tells the system to use the Bash interpreter to execute the script.
- # Ask the user for their name: This is a comment. It provides context about the upcoming code. Comments are ignored by the interpreter.
- echo “What’s your name?”: The echo command is used to display the text in double quotes on the terminal.
- read name: The read command waits for the user to input text and stores it in the variable name.
- echo “Hello, $name! Nice to meet you.”: This line uses the echo command to print a greeting message that includes the value of the name variable, which was collected from the user’s input.
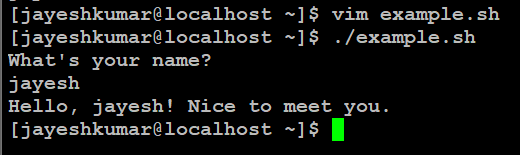
7) How do you add comments to a shell script?
Comments in shell scripting are used to provide explanations or context to the code. They are ignored by the interpreter and are only meant for humans reading the script. You can add comments using the # symbol.
#!/bin/bash
# This is a comment explaining the purpose of the script
echo “gfg”
8) Create a shell script that checks if a file exists in the current directory.
Here’s a script that checks if a file named “example.txt” exists in the current directory:
#!/bin/bash
file=”example.txt”
# Check if the file exists
if [ -e “$file” ]; then
echo “File exists: $file”
else
echo “File not found: $file”
fi
Explanation:
- #!/bin/bash: This is the shebang line that specifies the interpreter (/bin/bash) to be used for running the script.
- file=”example.txt”: This line defines the variable file and assigns the value “example.txt” to it. You can replace this with the name of the file you want to check for.
- if [ -e “$file” ]; then: This line starts an if statement. The condition [ -e “$file” ] checks if the file specified by the value of the file variable exists. The -e flag is used to check for file existence.
- echo “File exists: $file”: If the condition is true (i.e., the file exists), this line prints a message indicating that the file exists, along with the file’s name.
- else: If the condition is false (i.e., the file doesn’t exist), the script executes the code under the else branch.
- echo “File not found: $file”: This line prints an error message indicating that the specified file was not found, along with the file’s name.
- fi: This line marks the end of the if statement.
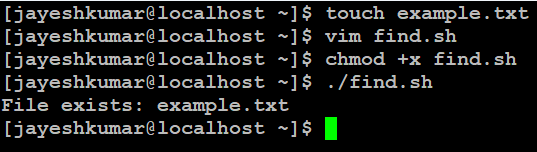
Finding file
9) What is the difference between single quotes (‘) and double quotes (“) in shell scripting?
Single quotes (‘) and double quotes (“) are used to enclose strings in shell scripting, but they have different behaviors:
- Single quotes: Everything between single quotes is treated as a literal string. Variable names and most special characters are not expanded.
- Double quotes: Variables and certain special characters within double quotes are expanded. The contents are subject to variable substitution and command substitution.
#!/bin/bash
abcd=”Hello”
echo ‘$abcd’ # Output: $abcd
echo “$abcd” # Output: Hello
10) How can you use command-line arguments in a shell script?
Command-line arguments are values provided to a script when it’s executed. They can be accessed within the script using special variables like $1, $2, etc., where $1 represents the first argument, $2 represents the second argument, and so on.
For Example: If our script name in `example.sh`
#!/bin/bash
echo “Script name: $0”
echo “First argument: $1”
echo “Second argument: $2”
If we run the script with `.example.sh hello_1 hello_2`, it will output:
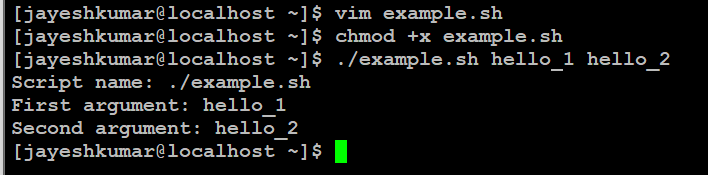
cli arguments
11) How do you use the for loop to iterate through a list of values?
Create a script name `example.sh`.
#!/bin/bash
fruits=(“apple” “banana” “cherry” “date”)
for fruit in “${fruits[@]}”; do
echo “Current fruit: $fruit”
done
Explanation:
`fruits=` line creates an array named fruits with four elements: “apple”, “banana”, “cherry”, and “date”.
- for fruit in “${fruits[@]}”; do: This line starts a for loop. Here’s what each part means:
- for fruit: This declares a loop variable called fruit. In each iteration of the loop, fruit will hold the value of the current element from the fruits array.
- “${fruits[@]}”: This is an array expansion that takes all the elements from the fruits array. The “${…}” syntax ensures that each element is treated as a separate item.
- do: This keyword marks the beginning of the loop body.
- echo “Current fruit: $fruit”: Inside the loop, this line uses the echo command to display the current value of the loop variable fruit. It prints a message like “Current fruit: apple” for each fruit in the array.
- done: This keyword marks the end of the loop body. It tells the script that the loop has finished.
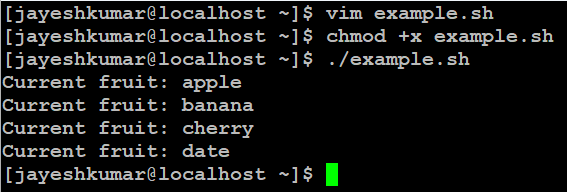
for loop
12) Write a shell script that calculates the sum of integers from 1 to N using a loop.
Create a script name `example.sh`.
#!/bin/bash
echo “Enter a number (N):”
read N
sum=0
for (( i=1; i<=$N; i++ )); do
sum=$((sum + i))
done
echo “Sum of integers from 1 to $N is: $sum”
Explanation:
The script starts by asking you to enter a number (N) using read. This number will determine how many times the loop runs.
- The variable sum is initialized to 0. This variable will keep track of the sum of integers.
- The for loop begins with for (( i=1; i<=$N; i++ )). This loop structure is used to repeat a set of actions a certain number of times, in this case, from 1 to the value of N.
- Inside the loop, these things happen:
- i=1 sets the loop variable i to 1 at the beginning of each iteration.
- The loop condition i<=$N checks if i is still less than or equal to the given number N.
- If the condition is true, the loop body executes.
- sum=$((sum + i)) calculates the new value of sum by adding the current value of i to it. This adds up the integers from 1 to the current i value.
- After each iteration, i++ increments the value of i by 1.
- The loop continues running until the condition i<=$N becomes false (when i becomes greater than N).
- Once the loop finishes, the script displays the sum of the integers from 1 to the entered number N.
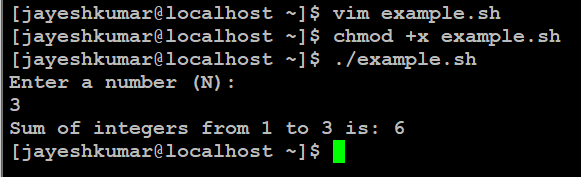
13) Create a script that searches for a specific word in a file and counts its occurrences.
Create a script name `word_count.sh`
#!/bin/bash
echo “Enter the word to search for:”
read target_word
echo “Enter the filename:”
read filename
count=$(grep -o -w “$target_word” “$filename” | wc -l)
echo “The word ‘$target_word’ appears $count times in ‘$filename’.”
Explanation:
- echo “Enter the word to search for:”: This line displays a message asking the user to enter a word they want to search for in a file.
- read target_word: This line reads the input provided by the user and stores it in a variable named target_word.
- echo “Enter the filename:”: This line displays a message asking the user to enter the name of the file they want to search in.
- read filename: This line reads the input provided by the user and stores it in a variable named filename.
- count=$(grep -o -w “$target_word” “$filename” | wc -l): This line does the main work of the script. Let’s break it down further:
- grep -o -w “$target_word” “$filename”: This part of the command searches for occurrences of the target_word in the specified filename. The options -o and -w ensure that only whole word matches are counted.
- |: This is a pipe, which takes the output of the previous command and sends it as input to the next command.
- wc -l: This part of the command uses the wc command to count the number of lines in the input. The option -l specifically counts the lines.
- The entire command calculates the count of occurrences of the target_word in the file and assigns that count to the variable coun
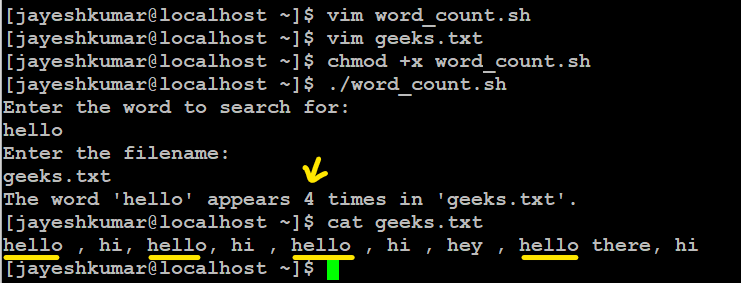
14) Explain the differences between standard output (stdout) and standard error (stderr).
The main difference between standard output (stdout) and standard error (stderr) is as follows:
- Standard Output (stdout): This is the default output stream where a command’s regular output goes. It’s displayed on the terminal by default. You can redirect it to a file using >.
- Standard Error (stderr): This is the output stream for error messages and warnings. It’s displayed on the terminal by default as well. You can redirect it to a file using 2>.
15) Explain the concept of conditional statements in shell scripting.
Conditional statements in shell scripting allow us to make decisions and control the flow of our script based on certain conditions. They enable our script to execute different sets of commands depending on whether a particular condition is true or false. The primary conditional statements in shell scripting are the if statement, the elif statement (optional), and the else statement (optional).
Here’s the basic structure of a conditional statement in shell scripting:
if [ condition ]; then
# Commands to execute if the condition is true
elif [ another_condition ]; then
# Commands to execute if another_condition is true (optional)
else
# Commands to execute if none of the conditions are true (optional)
fi
Explanation:
- [ condition ] = Command that evaluates the condition and returns a true (0) or false (non-zero) exit status.
- then = It is a keyword which indicates that the commands following it will be executed if the condition evaluates to true.
- elif = (short for “else if”) It is a section that allows us to specify additional conditions to check.
- else = it is a section that contains commands that will be executed if none of the conditions are true.
- fi = It is a keyword that marks the end of the conditional block.
16) How do you read lines from a file within a shell script?
To read lines from a file within a shell script, we can use various methods, but one common approach is to use a while loop in combination with the read command. Here’s how we can do it:
#!/bin/bash
file=”/home/jayeshkumar/jayesh.txt”
# Check if the file exists
if [ -e “$file” ]; then
while IFS= read -r line; do
echo “Line read: $line”
# Add your processing logic here
done < “$file”
else
echo “File not found: $file”
fi
Explanation:
- #!/bin/bash: This is the shebang line that specifies the interpreter (/bin/bash) to be used for running the script.
- file=”/home/jayeshkumar/jayesh.txt”: This line defines the variable file and assigns the full path to the file jayesh.txt in the /home/jayeshkumar directory. Change this path to match the actual path of the file you want to read.
- if [ -e “$file” ]; then: This line starts an if statement. It checks if the file specified by the variable $file exists. The -e flag checks for file existence.
- while IFS= read -r line; do: This line initiates a while loop that reads lines from the file.
- IFS=: The IFS (Internal Field Separator) is set to an empty value to preserve leading and trailing spaces.
- read -r line: This reads the current line from the file and stores it in the variable line.
- echo “Line read: $line”: This line prints the content of the line that was read from the file. The variable $line contains the current line’s content.
- # Add your processing logic here: This is a placeholder comment where you can add your own logic to process each line. For example, you might analyze the line, extract information, or perform specific actions based on the content.
- done < “$file”: This marks the end of the while loop. The < “$file” redirects the content of the file to be read by the loop.
- else: If the file does not exist (the condition in the if statement is false), the script executes the code under the else branch.
- echo “File not found: $file”: This line prints an error message indicating that the specified file was not found.
- fi: This line marks the end of the if statement.
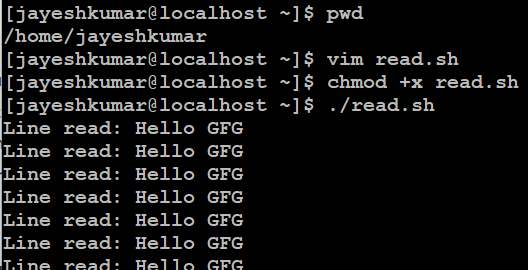
reading file
Here , we used `pwd` command to get the path of current directory.
17) Write a function in a shell script that calculates the factorial of a given number.
Here is the script that calculate the factorial of a given number.
#!/bin/bash
# Define a function to calculate factorial
calculate_factorial() {
num=$1
fact=1
for ((i=1; i<=num; i++)); do
fact=$((fact * i))
done
echo $fact
}
# Prompt the user to enter a number
echo “Enter a number: “
read input_num
# Call the calculate_factorial function with the input number
factorial_result=$(calculate_factorial $input_num)
# Display the factorial result
echo “Factorial of $input_num is: $factorial_result”
Explanation:
- The script starts with the shebang line #!/bin/bash to specify the interpreter.
- calculate_factorial() is defined as a function. It takes one argument, num, which is the number for which the factorial needs to be calculated.
- Inside the function, fact is initialized to 1. This variable will store the factorial result.
- The for loop iterates from 1 to the given number (num). In each iteration, it multiplies the current value of fact by the loop index i.
- After the loop completes, the fact variable contains the calculated factorial.
- The script prompts the user to enter a number using read.
- The calculate_factorial function is called with the user-provided number, and the result is stored in the variable factorial_result.
- Finally, the script displays the calculated factorial result.
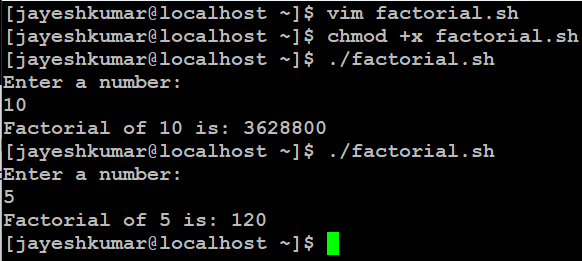
Factorial
18) How do you handle signals like Ctrl+C in a shell script?
In a shell script, you can handle signals like Ctrl+C (also known as SIGINT) using the trap command. Ctrl+C generates a SIGINT signal when the user presses it to interrupt the running script or program. By using the trap command, you can specify actions to be taken when a particular signal is received. Here’s how you handle signals like Ctrl+C in a shell script:
#!/bin/bash
cleanup() {
echo “Script interrupted. Performing cleanup…”
# Add your cleanup actions here
exit 1
}
# Set up a trap to call the cleanup function when Ctrl+C (SIGINT) is received
trap cleanup SIGINT
# Rest of your script
echo “Running…”
sleep 10
echo “Finished.”
Handling signals is important for making scripts robust and ensuring that they handle unexpected interruptions gracefully. You can customize the cleanup function to match your specific needs, like closing files, stopping processes, or logging information before the script exits.
Explanation:
- #!/bin/bash: This shebang line specifies the interpreter to be used for running the script.
- cleanup() { … }: This defines a function named cleanup. Inside this function, you can include any actions that need to be performed when the script is interrupted, such as closing files, releasing resources, or performing other cleanup tasks.
- trap cleanup SIGINT: The trap command is used to set up a signal handler. In this case, it specifies that when the SIGINT signal (Ctrl+C) is received, the cleanup function should be executed.
- echo “Running…”, sleep 10, echo “Finished.”: These are just sample commands to simulate the execution of a script.
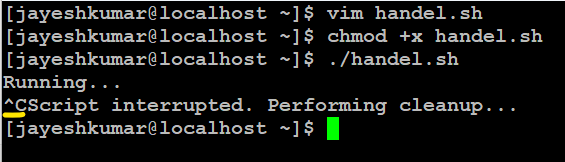
19) Create a script that checks for and removes duplicate lines in a text file.
Here is our linux scipt in which we will remove duplicate lines from a text file.
#!/bin/bash
input_file=”input.txt”
output_file=”output.txt”
sort “$input_file” | uniq > “$output_file”
echo “Duplicate lines removed successfully.”
Explanation:
- The script starts with a shebang (#!/bin/bash), which indicates that the script should be interpreted using the Bash shell.
- The input_file variable is set to the name of the input file containing duplicate lines (change this to your actual input file name).
- The output_file variable is set to the name of the output file where the duplicates will be removed (change this to your desired output file name).
- The script uses the sort command to sort the lines in the input file. Sorting the lines ensures that duplicate lines are grouped together.
- The sorted lines are then passed through the uniq command, which removes consecutive duplicate lines. The output of this process is redirected to the output file.
- After the duplicates are removed, the script prints a success message.
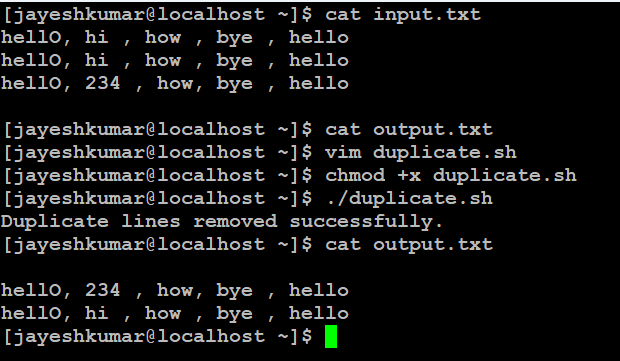
duplicate line removing
Here, we use `cat` to display the text inside the text file.
20) Write a script that generates a secure random password.
Here is our script to generate a secure random password.
#!/bin/bash
# Function to generate a random password
generate_password() {
tr -dc ‘A-Za-z0-9!@#$%^&*()_+{}[]’ < /dev/urandom | fold -w 12 | head -n 1
}
# Call the function and store the generated password
password=$(generate_password)
echo “Generated password: $password”
Note: User can accordingly change the length of there password, by replacing the number `12`.
Explanation:
- The script starts with a shebang (#!/bin/bash), indicating that it should be interpreted using the Bash shell.
- The generate_password function is defined to generate a random password. Here’s how it works:
- tr -dc ‘A-Za-z0-9!@#$%^&*()_+{}[]’ < /dev/urandom uses the tr command to delete (-d) characters not in the specified set of characters (A-Za-z0-9!@#$%^&*()_+{}[]) from the random data provided by /dev/urandom.
- fold -w 12 breaks the filtered random data into lines of width 12 characters each.
- head -n 1 selects the first line, effectively giving us a random sequence of characters of length 12.
- The password variable is assigned the result of calling the generate_password function.
- Finally, the generated password is displayed using echo.
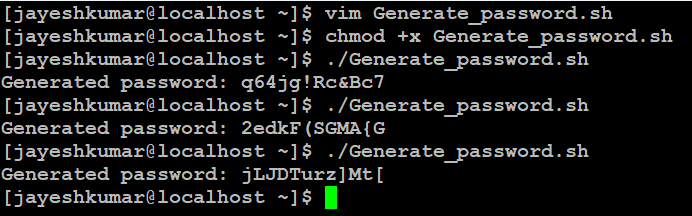
21) Write a shell script that calculates the total size of all files in a directory.
Here is a shell script to calculate the total size of all files in a directory.
#!/bin/bash
directory=”/path/to/your/directory”
total_size=$(du -csh “$directory” | grep total | awk ‘{print $1}’)
echo “Total size of files in $directory: $total_size”
Explanation:
- The script starts with the #!/bin/bash shebang, indicating that it should be interpreted using the Bash shell.
- The directory variable is set to the path of the directory for which you want to calculate the total file size. Replace “/path/to/your/directory” with the actual path.
- The du command is used to estimate file space usage. The options used are:
- -c: Produce a grand total.
- -s: Display only the total size of the specified directory.
- -h: Print sizes in a human-readable format (e.g., KB, MB, GB).
- The output of du is piped to grep total to filter out the line that contains the total size.
- awk ‘{print $1}’ is used to extract the first field (total size) from the line.
- The calculated total size is stored in the total_size variable.
- Finally, the script displays the total size using echo.
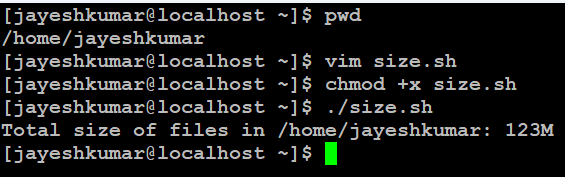
Total Size of Files
Here , we used `pwd` command to see the current directory path.
22) Explain the difference between if and elif statements in shell scripting.
Purpose |
Explain the difference between if and elif statements in shell scripting. |
Provides alternative conditions to check when the initial if condition is false. |
usage |
Used for the initial condition. |
Used after the initial if condition to check additional conditions. |
number of Blocks |
Can have only one if block. |
Can have multiple elif blocks, but only one else block (optional). |
Execution |
Executes the block of code associated with the if statement if the condition is true. If the condition is false, the else block (if present) is executed (optional). |
Checks each elif condition in order. If one elif condition is true, the corresponding block of code is executed, and the script exits the entire conditional block. If none of the elif conditions are true, the else block (if present) is executed. |
Nested Structures |
Can be nested within other if, elif, or else blocks. |
Cannot be nested within another elif block, but can be used inside an if or else block. |
Lets understand it by an example.
#!/bin/bash
number=5
if [ $number -gt 10 ]; then
echo “$number is greater than 10”
else
echo “$number is not greater than 10”
fi
echo “——–“
if [ $number -gt 10 ]; then
echo “$number is greater than 10”
elif [ $number -eq 10 ]; then
echo “$number is equal to 10”
else
echo “$number is less than 10”
fi
Explanation:
In this example, the first if block checks whether number is greater than 10. If not, it prints a message indicating that the number is not greater than 10. The second block with elif statements checks multiple conditions sequentially until one of them is true. In this case, since the value of number is 5, the output will be:
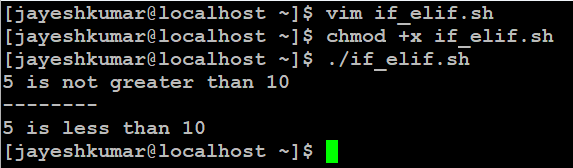
if_elif difference
23) How do you use a while loop to repeatedly execute commands?
A while loop is used in shell scripting to repeatedly execute a set of commands as long as a specified condition is true. The loop continues executing the commands until the condition becomes false.
Here’s the basic syntax of a while loop:
while [ condition ]; do
# Commands to be executed
done
Explanation:
- The `while` loop starts with the keyword `while` followed by a condition enclosed within square brackets `[ ]`.
- The loop’s body, which contains the commands to be executed, is enclosed within the `do` and `done` keywords.
- The loop first checks the condition. If the condition is true, the commands within the loop body are executed. After the loop body executes, the condition is checked again, and the process repeats until the condition becomes false.
Example: If we want to print numbers from 1 to 5
#!/bin/bash
counter=1
while [ $counter -le 5 ]; do
echo “Number: $counter”
counter=$((counter + 1))
done
Explanation:
- The counter variable is set to 1.
- The while loop checks whether the value of counter is less than or equal to 5. As long as this condition is true, the loop continues executing.
- Inside the loop, the current value of counter is printed using echo.
- The counter is incremented by 1 using the expression $((counter + 1)).
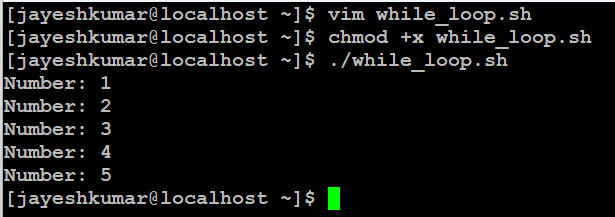
while loop
24) Create a shell script that finds and lists all empty files in a directory.
Shell script that you can use to find and list all empty files in a directory using the `find` and `stat` commands:
#!/bin/bash
directory=”$1″
if [ -z “$directory” ]; then
echo “Usage: $0 <directory>”
exit 1
fi
if [ ! -d “$directory” ]; then
echo “Error: ‘$directory’ is not a valid directory.”
exit 1
fi
echo “Empty files in $directory:”
find “$directory” -type f -empty
Explanation:
- `#!/bin/bash`: This is called a “shebang,” and it tells the operating system to use the Bash shell to interpret and execute the script.
- `directory=”$1″`: This line assigns the first command-line argument (denoted by $1) to the variable `directory`.
- `if [ -z “$directory” ]; then`: This line starts an if statement that checks whether the `directory` variable is empty (-z tests for an empty string).
- `echo “Usage: $0 <directory>”`: If the directory is empty, this line prints a usage message, where `$0` represents the script’s name.
- `exit 1`: This line exits the script with an exit code of `1`, indicating an error.
- `fi`: This line marks the end of the `if` statement.
- `if [ ! -d “$directory” ]; then`: This starts another if statement to check if the provided directory exists (`-d` tests for a directory).
- `echo “Error: ‘$directory’ is not a valid directory.”`: If the provided directory doesn’t exist, this line prints an error message.
- `exit 1`: Exits the script with an exit code of `1`.
- `fi`: Marks the end of the second `if` statement.
- `echo “Empty files in $directory:”`: If everything is valid so far, this line prints a message indicating that the script will list empty files in the specified directory.
- `find “$directory” -type f -empty`: This line uses the `find` command to search for empty files (`-empty`) of type regular files (`-type f`) in the specified directory. It then lists these empty files.
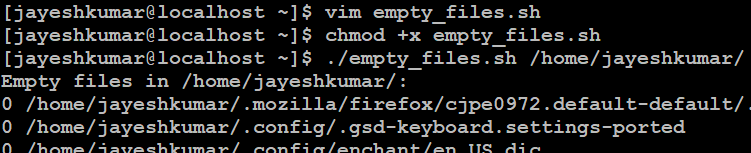
Finding empty files
Note: We need to provide a directory as an argument when running the script. Here we have used the path of current directory “home/jayeshkumar/”
25) What is the purpose of the read command in shell scripting?
The read command in shell scripting lets the script ask you for information. It’s like when a computer asks you a question and waits for your answer. This is useful for scripts that need you to type something or for when the script needs to work with information from files. The read command helps the script stop and wait for what you type, and then it can use that information to do more things in the script.
Syntax of read command:
read variable_name
Example: If we want to take name as an input from user to print it.
#!/bin/bash
echo “Please enter your name:”
read name
echo “Hello, $name!”
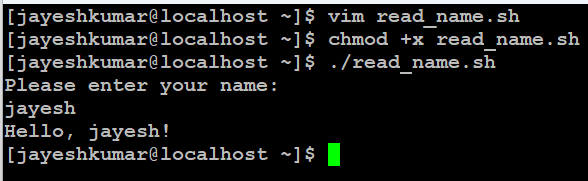
read nam
In summary, the read command is used to capture user input or data from files within shell scripts, making the scripts more interactive and versatile.
26) Write a shell script that converts all filenames in a directory to lowercase.
Here’s a shell script that converts all filenames in a directory to lowercase.
#!/bin/bash
directory=”$1″
if [ -z “$directory” ]; then
echo “Usage: $0 <directory>”
exit 1
fi
if [ ! -d “$directory” ]; then
echo “Error: ‘$directory’ is not a valid directory.”
exit 1
fi
cd “$directory” || exit 1
for file in *; do
if [ -f “$file” ]; then
newname=$(echo “$file” | tr ‘A-Z’ ‘a-z’)
[ “$file” != “$newname” ] && mv “$file” “$newname”
fi
done
Explanation:
- #!/bin/bash: This is the shebang, specifying that the script should be interpreted using the Bash shell.
- directory=”$1″: This line assigns the first command-line argument to the variable directory.
- if [ -z “$directory” ]; then: This line checks if the directory variable is empty (no argument provided when running the script).
- echo “Usage: $0 <directory>”: If the directory is empty, this line prints a usage message with the script’s name ($0).
- exit 1: This line exits the script with an exit code of 1, indicating an error occurred.
- fi: This marks the end of the first if statement.
- if [ ! -d “$directory” ]; then: This line checks if the specified directory does not exist (-d tests for a directory).
- echo “Error: ‘$directory’ is not a valid directory.”: If the specified directory doesn’t exist, this line prints an error message.
- exit 1: Exits the script with an exit code of 1.
- fi: Marks the end of the second if statement.
- cd “$directory” || exit 1: Changes the current working directory to the specified directory. If the directory change fails (e.g., non-existent directory), the script exits with an error code.
- for file in *; do: Ifor file in *; do:nitiates a loop that iterates over all items in the current directory (* matches all filenames).
- if [ -f “$file” ]; then: Checks if the current loop iteration item is a regular file (-f tests for a regular file).
- newname=$(echo “$file” | tr ‘A-Z’ ‘a-z’): Converts the current filename ($file) to lowercase using the tr command and stores the result in the newname variable.
- [ “$file” != “$newname” ] && mv “$file” “$newname”: Compares the original filename with the new lowercase filename. If they are different, it renames the file using the mv command.
- fi: Marks the end of the inner if statement.
- done: Marks the end of the loop.
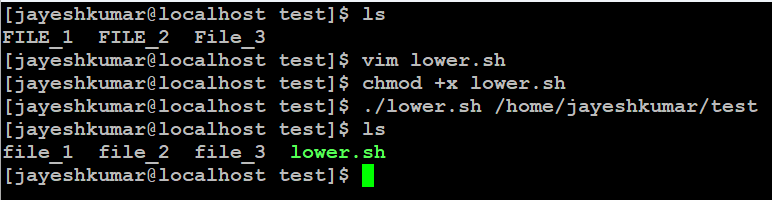
Note: We need to provide a directory as an argument when running the script. Here we have used the path of current directory “home/jayeshkumar/test”
27) How can you use arithmetic operations within a shell script?
Arithmetic operations can be performed within a shell script using various built-in methods. The shell provides mechanisms for simple arithmetic calculations using arithmetic expansion Like:
- Arithmetic Expansion ($((…)))
- Using expr Command
- Using let Command
Here is our Shell script explaning all three methods for arithmetic operations.
#!/bin/bash
num1=10
num2=5
#Arithmetic Expansion ($((…)))
result=$((num1 + num2))
echo “Sum: $result”
#Using expr Command
sum=$(expr $num1 + $num2)
echo “Sum: $sum”
#Using let Command
let “sum = num1 + num2”
echo “Sum: $sum”
Explanation:
- `#!/bin/bash`: This is the shebang, specifying that the script should be interpreted using the Bash shell.
- `num1=10` and `num2=5`: These lines assign the values 10 and 5 to the variables `num1` and `num2`, respectively.
- `#Arithmetic Expansion ($((…)))`: This is a comment indicating the start of the section that demonstrates arithmetic expansion.
- `result=$((num1 + num2))`: This line uses arithmetic expansion to calculate the sum of `num1` and `num2` and stores the result in the `result` variable.
- `echo “Sum: $result”`: This line prints the calculated sum using the value stored in the `result` variable.
- `#Using expr Command`: This is a comment indicating the start of the section that demonstrates using the `expr` command for arithmetic operations.
- `sum=$(expr $num1 + $num2)`: This line uses the `expr` command to calculate the sum of `num1` and `num2` and stores the result in the `sum` variable. Note that the `expr` command requires spaces around the operators.
- `echo “Sum: $sum”`: This line prints the calculated sum using the value stored in the `sum` variable.
- `#Using let Command`: This is a comment indicating the start of the section that demonstrates using the `let` command for arithmetic operations.
- `let “sum = num1 + num2″`: This line uses the `let` command to calculate the sum of `num1` and `num2` and assigns the result to the `sum` variable. The `let` command does not require spaces around the operators.
- `echo “Sum: $sum”`: This line prints the calculated sum using the value stored in the `sum` variable.
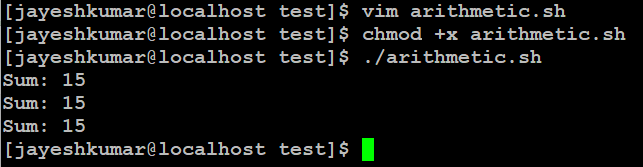
arithmetic
28) Create a script that checks if a network host is reachable.
Here’s a simple shell script that uses the ping command to check if a network host is reachable:
#!/bin/bash
host=”$1″
if [ -z “$host” ]; then
echo “Usage: $0 <hostname or IP>”
exit 1
fi
ping -c 4 “$host”
if [ $? -eq 0 ]; then
echo “$host is reachable.”
else
echo “$host is not reachable.”
fi
Explanation:
- It takes a hostname or IP address as an argument and checks if the argument is provided.
- If no argument is provided, it displays a usage message and exits.
- It uses the ping command with the -c 4 option to send four ICMP echo requests to the specified host.
- After the ping command runs, it checks the exit status ($?). If the exit status is 0, it means the host is reachable and the script prints a success message. Otherwise, it prints a failure message.
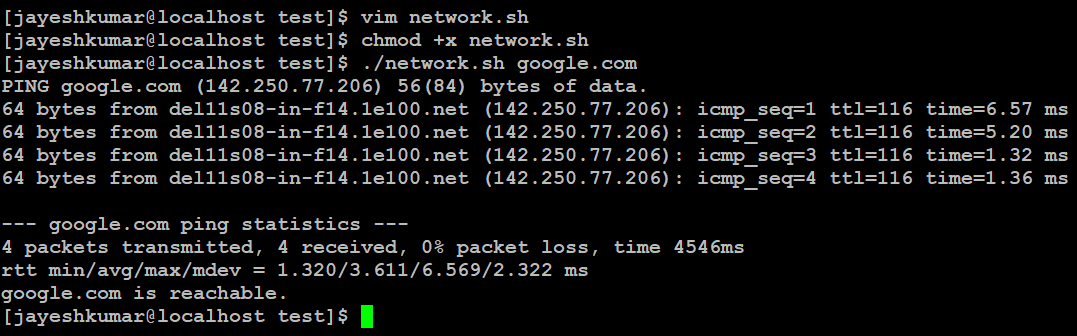
Note: We need to provide a hostname as an argument when running the script. Here we have used “google.com”
29) Write a Shell Script to Find the Greatest Element in an Array:
Here’s a shell script to find the greatest element in an array.
#!/bin/bash
# Declare an array
array=(3 56 24 89 67)
# Initialize a variable to store the maximum value, starting with the first element
max=${array[0]}
# Iterate through the array
for num in “${array[@]}”; do
# Compare each element with the current maximum
if ((num > max)); then
max=$num
fi
done
# Print the maximum value
echo “The maximum element in the array is: $max”
Explanation:
- `#!/bin/bash`: The shebang line specifies that the script should be interpreted using the Bash shell.
- `array=(3 56 24 89 67)`: The array is declared and initialized with values.
- `max=${array[0]}`: `max` is initialized with the first element of the array.
- `for num in “${array[@]}”; do`: A `for` loop is used to iterate through the elements of the array.
- `if ((num > max)); then`: An `if` statement checks if the current element `num` is greater than the current maximum `max`.
- `max=$num`: If`num` is greater than `max`, `max` is updated with the value of num.
- `done`: The `for` loop is closed.
- `echo “The maximum element in the array is: $max”`: Finally, the script prints the maximum value found in the array.
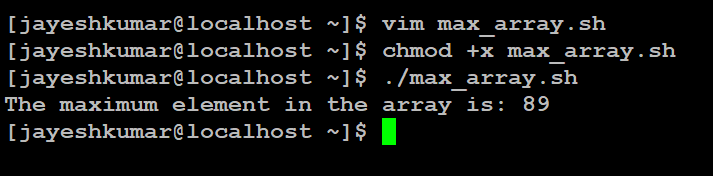
greatest number
30) Write a scripte to calculate the sum of Elements in an Array.
#!/bin/bash
# Declare an array
array=(1 65 22 19 94)
# Initialize a variable to store the sum
sum=0
# Iterate through the array and add each element to the sum
for num in “${array[@]}”; do
sum=$((sum + num))
done
# Print the sum
echo “The sum of elements in the array is: $sum”
Explanation:
`#!/bin/bash`: The shebang line specifies that the script should be interpreted using the Bash shell.
`array=(1 65 22 19 94)`: The array is declared and initialized with values.
`sum=0`: `sum` is initialized to zero to hold the sum of elements.
`for num in “${array[@]}”; do`: A `for` loop is used to iterate through the elements of the array.
`sum=$((sum + num))`: Inside the loop, each element `num` is added to the `sum` variable.
`done`: The `for` loop is closed.
`echo “The sum of elements in the array is: $sum”`: Finally, the script prints the sum of all elements in the array.
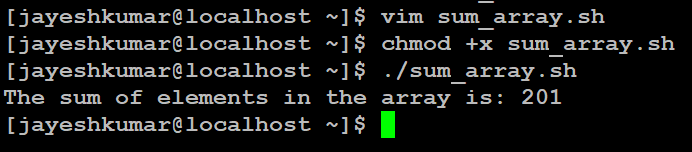
Sum of Elements
Know More About Shell Scripts
Conclusion
We all geeks know that shell script is very useful to increases the work productivity as well as it save time also. So, in this article we have covered 30 very useful and most conman shell scripts examples. We hope that this complete guide on shell scripting example help you to understand the all about the shell scripts.
Share your thoughts in the comments
Please Login to comment...