Spring Boot – Internationalization
Last Updated :
17 Dec, 2023
Before understanding Spring Boot-Internationalization, we must know what internalization in general means. Internationalization is an action or process of making something international which means making things adaptable or available everywhere. In this article, we will be discussing Internationalization in Spring Boot.
What is Spring Boot-Internationalization?
Spring Boot-Internationalization is nothing but it’s a framework that aims to build spring applications and make them available for users around the world. Every user might be proficient in their languages. So, this framework makes your spring application available to everyone. Spring Boot-Internationalization has many features that assist all programmers in internationalizing their applications.
Examples of websites that use the Internationalization Concept:
- Amazon Application in French
- Amazon Application in English
Prerequisites of the Topic:
- Good understanding of Java Programming language as Spring Boot Uses Java libraries as well.
- Sound Knowledge in Spring Boot Framework.
- A basic understanding of terms like Internationalization, resource files, localization, and locale would be a plus.
Kew features of Spring Boot-Internationalization
- Resource files consist of localized textual data and other data, and this is used in Spring Boot.
- The Resource files are named according to the local code of language that it contain. Example- messages_en.properties for English and messages_fr.properties for French.
- To retrieve the localized textual data from the resource files there’s an interface called MessageSource.
- LocaleResolver is an interface that helps in identifying the user’s current language.
Whenever there are HTTP requests on the web the LocaleChangeInterceptor acts as an interceptor that changes the user’s locale according to the request parameters.
Note: Locale is nothing but the information of the users like their language, geographical region etc.
Why Spring Boot Internationalization?
- User-Friendly
- Global Reach
- Broader Market
- Different Users (from language,culture…etc) can use the application at one place.
A Comprehensive Approach for Internationalizing a Spring Boot Application:
- Creating Resource Files: As we already learnt that users might be from different geographical areas and have possess different languages. So, create individual files for every language. These files contains key-value format data which has their localized data.
- Configuration of Message Source: Now Configure the Message Source Bean so that exact resource file will be loaded properly.
- Configuration of Locale Resolver: Spring Boot has many Locale Resolvers like AcceptLanguageHeaderLocaleResolver and SessionLocalResolver. So, make sure you configure the locale Resolver to get the user’s locale easily.
- Retrieving the Localized Text: Retrieve the data from the Resource Files using the Message Source Interface. The Best Part of this is that we can retrieve the data from any part of our code.
- Testing: Final and Most Important thing is rigorous testing. Bugs are inevitable most of the times. So, make sure testing each and every part of your code. Especially in your Configuration files for selecting a language. Frequently developers forget to check the expected output Matches with the language specific data.
Example of Spring Boot – Internationalization:
Before Implementing code let’s see what all dependencies we need for Spring Boot – Internationalization:
1. Maven Dependencies
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
2. Main Class
Java
@SpringBootApplication
public class InternationalizationApplication {
public static void main(String[] args) {
SpringApplication.run(InternationalizationApplication. class , args);
}
}
|
3. Creating Resource Files
Make Sure you create the files for every individual language your application supports.
- For English – messages_en.properties file.
- For French – messages_fr.properties file.
Your File can contain following properties:
For English:
Welcome_message=Welcome to the application
login_label=Login
username_label=username
password_label=password
login_button =Login
For French:
Welcome_message=Bienvenue dans 1 'application
login_label=Connexion
username_label=Nom d'utilisateur
password_label=Mot de passe
login_button =Connexion
4.Configure the Message Source and LocaleChangeInterceptor in your application context file
XML
< bean id = "messageSource"
class = "org.springframework.context.support.ResourceBundleMessageSource" >
< property name = "basenames" value = "messages" />
</ bean >
< bean id = "localeChangeInterceptor"
class = "org.springframework.web.servlet.i18n.LocaleChangeInterceptor" >
< property name = "paramName" value = "locale" />
</ bean >
|
5. Configuring the Message Source
Now Configure the Message Source bean for loading your resource file. Note this step is very important because this bean loads your resource file which is based on the user’s locale. You can Implement all this in your configuration class. Refer the following code to know how to load your resource file.
Java
@Configuration
public class msgConfig
{
@Bean
public MessageSource msgSource()
{
ResourceBundleMessageSource msgSource = new ResourceBundleMessageSource();
msgSource.setBasenames( "messages" );
return msgSource;
}
}
|
The above configuration code will load your resource files which are declared as messages_en.properties, messages_fr.properties and whatever languages’ properties files you created.
6.Configuring the Locale Resolver
Configure the locale Resolver to get the user’s locale easily. This also can be implemented in your configuration class. As we seen that Spring Boot has many Locale Resolvers. Here we’ll implement AcceptLanguageHeaderLocaleResolver. This helps the locale based on the accept language HTTP header.
Java
@Configuration
public class LocaleConfiguration {
@Bean
public LocaleResolver localeRes(){
AcceptLanguageHeaderLocaleResolver localeResolver = new AcceptLanguageHeaderLocaleResolver();
localeResolver.setDefaultLocale(Locale.English);
return localeResolver;
}
}
|
The above configuration setup will make sure the default locale to english language if the accept language header doesn’t find the users locale.
7. Retrieving the Localized data/Text
Now we will implement the Message Source interface for retrieving the localized data from the resource files. We will retrieve the data from the controller class. Note that we can retrieve the localized text from any part of our code.
Java
@Controller
@RequestMapping ( "/SpringInternationalization" )
public class ControllerClass {
@Autowired
private MessageSource msgSource;
@GetMapping ( "/english" )
public String index()
{
Locale locale=LocaleContextHolder.getLocale();
Stirng msg=msgSource.getMessage( "Message" , null ,locale);
return "english" ;
}
}
|
The above controller makes sures that the data will be retrieved from the appropriate resource file which is according to the users’ locale. Here LocaleContextHolder is used for fetching the current locale and the getMessage() method is used to get the localized text/data for the key “Message”. It Basically handles the Http Request and generates the desired response.
Output:
The Output depends on the user’s locale whether the user is English or French.
1. If User’s Locale is English the Output would be:
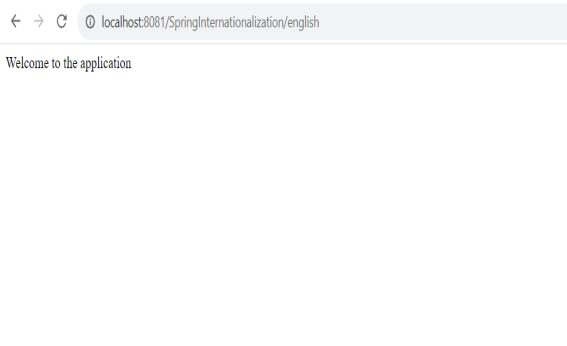
2. If User’s Locale is French the Output would be:
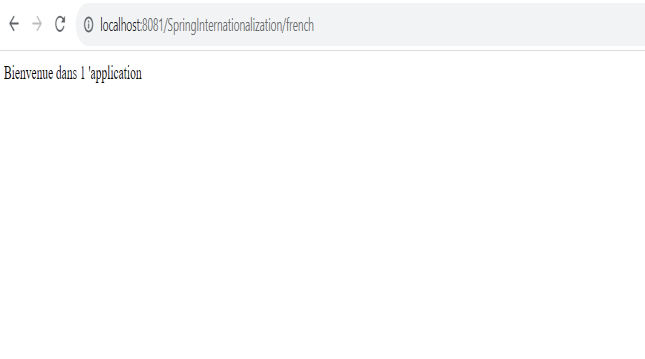
Note: The Message Resource Bean make sures that the appropriate file is loaded from the resource files depending upon the user’s locale. AcceptLanguageHeaderLocaleResolver bean is implemented for finding the users locale which according to the accept language HTTP header. So, if this header is not found the default language will be taken into consideration. Here we gave English as our default language.
Conclusion
The Spring Boot Internationalization is a tool which impacts on reaching your application in a Wider Audience Broader market. The kind of Global Reach it gives is something that developers can be proud of. The Important thing is that it simplifies the process of creating the multilingual applications. Where generally creating individual or separate application for each and every language would be a tedious job for the developers to develop an application. The Users of different languages/cultures/geographical areas can now access their application in a pretty much user-friendly manner.
Share your thoughts in the comments
Please Login to comment...