Search Bar using HTML, CSS and JavaScript
Last Updated :
26 Dec, 2023
Every website needs a search bar through which a user can search the content of their concern on that page. A basic search bar can be made using HTML, CSS, and JavaScript only. Advanced searching algorithms look for many things like related content and then show the results. The one that we are going to make will look for substrings in a string.
Preview Image:
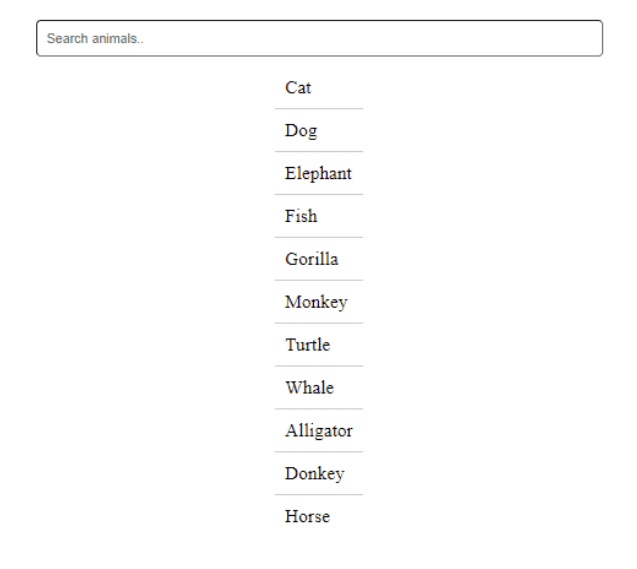
Prerequisites
Approach
- Create HTML with a search input and an ordered list of animals.
- Now assign the relevant IDs and classes to particular input boxes and list items.Â
- Apply initial styles for the container, search bar, and list items. Consider animations or transitions for visual appeal.
- Write a function (
search_animal()
) to handle input, loop through items, and toggle display based on content match.
Example: In this example, we will see the implementation of the above search bar with HTML and with an example.
Javascript
function search_animal() {
let input = document.getElementById( 'searchbar' ).value
input = input.toLowerCase();
let x = document.getElementsByClassName( 'animals' );
for (i = 0; i < x.length; i++) {
if (!x[i].innerHTML.toLowerCase().includes(input)) {
x[i].style.display = "none" ;
}
else {
x[i].style.display = "list-item" ;
}
}
}
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Search Bar Example</ title >
< link rel = "stylesheet"
type = "text/css" href = "./style.css" >
</ head >
< body >
< div class = "container" >
< input id = "searchbar"
onkeyup = "search_animal()"
type = "text" name = "search"
placeholder = "Search animals.." >
< ul id = 'list' >
< li class = "animals" >Cat</ li >
< li class = "animals" >Dog</ li >
< li class = "animals" >Elephant</ li >
< li class = "animals" >Fish</ li >
< li class = "animals" >Gorilla</ li >
< li class = "animals" >Monkey</ li >
< li class = "animals" >Turtle</ li >
< li class = "animals" >Whale</ li >
< li class = "animals" >Alligator</ li >
< li class = "animals" >Donkey</ li >
< li class = "animals" >Horse</ li >
</ ul >
</ div >
< script src = "./script.js" ></ script >
</ body >
</ html >
|
CSS
* {
margin : 0 ;
padding : 0 ;
box-sizing: border-box;
}
.container {
display : flex;
flex- direction : column;
align-items: center ;
margin : 20px ;
}
#searchbar {
margin : 10px ;
padding : 10px ;
border-radius: 5px ;
width : 50% ;
box-sizing: border-box;
}
#list {
list-style : none ;
padding : 0 ;
margin : 0 ;
}
.animals {
font-size : 1.2em ;
padding : 10px ;
border-bottom : 1px solid #ccc ;
animation: fadeIn 0.5 s ease-in-out;
}
.animals:last-child {
border-bottom : none ;
}
@keyframes fadeIn {
from {
opacity: 0 ;
transform: translateY( -10px );
}
to {
opacity: 1 ;
transform: translateY( 0 );
}
}
|
Output:
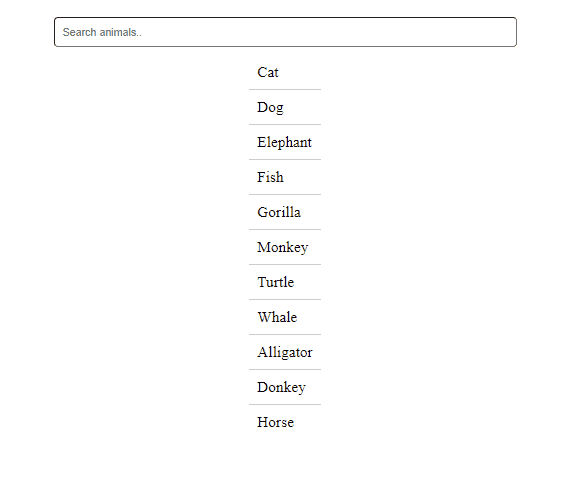
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples.
HTML is the foundation of webpages, is used for webpage development by structuring websites and web apps.You can learn HTML from the ground up by following this HTML Tutorial and HTML Examples.
CSS is the foundation of webpages, is used for webpage development by styling websites and web apps.You can learn CSS from the ground up by following this CSS Tutorial and CSS Examples.
Share your thoughts in the comments
Please Login to comment...