Scrape a popup using python and selenium
Last Updated :
31 Jul, 2023
Web scraping is the process of extracting data from websites. It involves programmatically accessing web pages, parsing the HTML or XML content, and extracting the desired information. With the help of libraries like BeautifulSoup, Selenium, or Scrapy in Python, web scraping can be done efficiently. It enables automating data collection, scraping product details, scraping news articles, and more, making it a valuable technique for data extraction and analysis.
Selenium
Selenium plays a crucial role in web scraping by providing a powerful framework for automating web browsers. While other libraries like BeautifulSoup or Scrapy are commonly used for parsing HTML and extracting data from web pages, Selenium allows you to simulate human-like interactions with websites. It can navigate through pages, click buttons, fill out forms, and handle JavaScript-generated content. It is a popular open-source framework that allows you to automate web browsers. It provides a convenient API for interacting with web elements, navigating pages, submitting forms, and performing various other web-related tasks
Advantages of using selenium:
- Selenium supports multiple web browsers
- Selenium can be used to create robust and scalable test automation frameworks
- Selenium provides features for debugging and troubleshooting automation scripts
How Can I use Selenium in my projects
1. Install the Selenium library in the Python environment
Execute the following command to install the selenium package using pip
pip install selenium
- Selenium requires a WebDriver to interface with the chosen browser. WebDriver is a separate executable that needs to be installed and configured.
- For example, to use Chrome with Selenium, you need to download the Chrome WebDriver from the official Selenium website or the ChromeDriver downloads page.
2. Importing Selenium
To use Selenium in your Python script, import the webdriver
module
from selenium import webdriver
3. Setting up a WebDriver instance
Create an instance of the desired browser’s WebDriver class to interact with the browser
driver = webdriver.Chrome() # Chrome WebDriver
4. Interacting with web elements
Selenium provides methods to locate and interact with web elements on a page, such as finding elements by ID, class name, CSS selector, or XPath
Example methods:
element = driver.find_element(BY.ID, "element_id")
element.click()
element.send_keys("text")
5. Handling popups and alerts
- Selenium enables you to handle various types of popups and alerts that may appear during automated browsing, such as JavaScript alerts, modal dialogs, and browser notifications.
- You can switch to and interact with popup windows or accept/dismiss alerts using appropriate WebDriver methods.
- the
switch_to.frame()
method is used to switch the focus to an iframe (inline frame) within a web page. This allows you to interact with elements inside the iframe. Here’s how you can use switch_to.frame()
:
iframe = driver.find_element_by_id("iframe_id")
driver.switch_to.frame(iframe)
button = driver.find_element_by_xpath("//button[@class='button-class']")
button.click()
6. Close the browser
It’s essential to properly close the browser instance to release resources and terminate the WebDriver.
driver.quit()
Full code
Python
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
driver = webdriver.Chrome()
driver.maximize_window()
try :
time.sleep( 3 )
driver.find_element(By.XPATH, '//*[@id="userProfileId"]' ).click()
print ( "Clicked on Element!" )
time.sleep( 5 )
email = driver.find_element(By.XPATH, '//*[@id="luser"]' )
passwd = driver.find_element(By.XPATH, '//*[@id="password"]' )
time.sleep( 3 )
email.clear()
email.send_keys( 'classes@geeksforgeeks.org' )
time.sleep( 2 )
passwd.clear()
passwd.send_keys( 'geeksforgeeks' )
time.sleep( 3 )
print ( "Info changed successfully" )
except Exception as ex:
print (ex)
finally :
driver.quit()
|
Output:
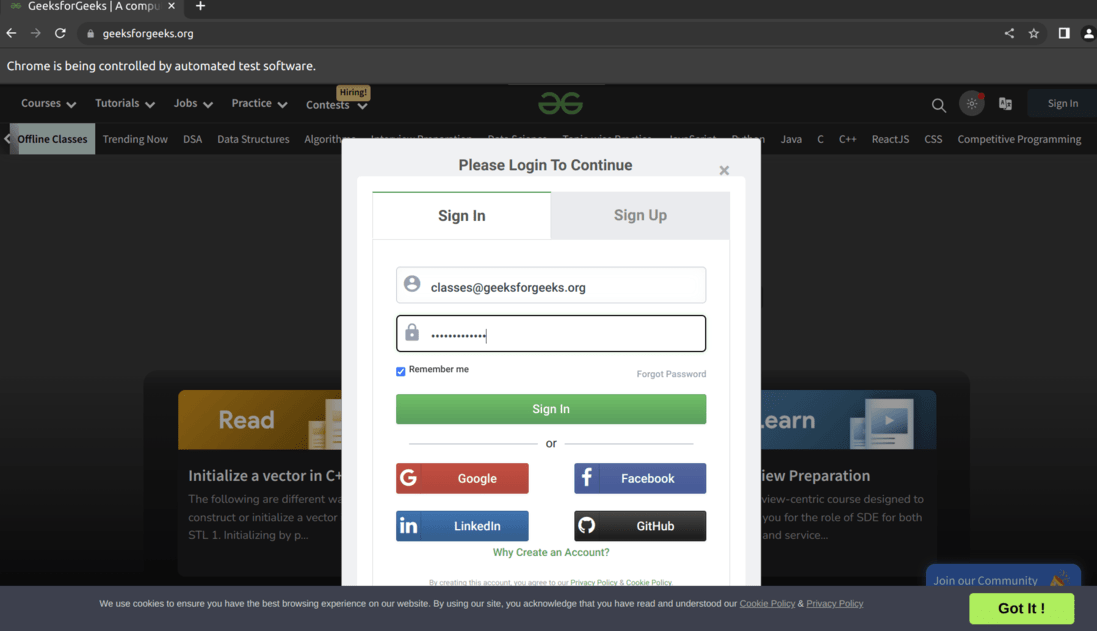
Conclusion:
By following the steps outlined in this article, you can integrate Selenium into your Python projects and leverage its capabilities to automate web interactions and perform efficient testing. Overall, Selenium with Python is a valuable tool for web automation and testing, enabling you to save time and effort while ensuring the quality and reliability of your web applications.
Share your thoughts in the comments
Please Login to comment...