How to Iterate Over Characters of a String in JavaScript ?
Last Updated :
28 Nov, 2023
In this article, we are going to learn about Iteration over characters of a string in JavaScript. Iterating over characters of a string in JavaScript involves sequentially accessing and processing each individual character within the string using loops or iteration methods for manipulation or analysis.
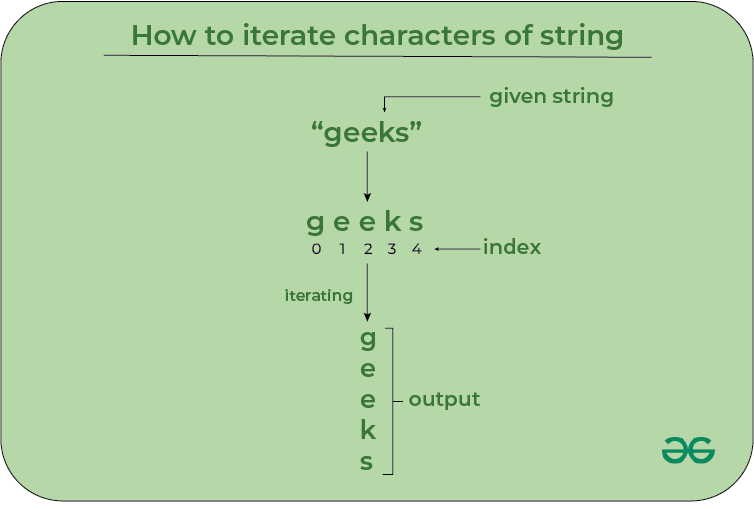
There are several methods that can be used to Iterate over characters of a string in JavaScript, which are listed below:
In this approach, we are using a for loop to iterate over a string’s characters by indexing each character based on the length of the string and accessing characters at each index.
Syntax:
for (statement 1 ; statement 2 ; statement 3){
code here...
};
Example: In this example, we are using the above-explained method.
Javascript
const str = "GeeksforGeeks" ;
for (let i = 0; i < str.length; i++) {
console.log(str[i]);
};
|
Output
G
e
e
k
s
f
o
r
G
e
e
k
s
In this approach, we Iterate over characters of a string using a for…of loop, which directly iterates through each character in the string without the need for indexing.
Syntax:
for ( variable of iterableObjectName) {
...
}
Example: In this example we are using the above-explained approach.
Javascript
const str = "Geeks" ;
for (const char of str) {
console.log(char);
};
|
In this approach, we are using forEach() method on an array created from the string to iterate through characters individually.
Syntax:
array.forEach(callback(element, index, arr), thisValue)
Example: In this example we are using forEach() method to itrate characters of our given string.
Javascript
const str = "Geeks" ;
Array.from(str).forEach(elem => {
console.log(elem);
});
|
In this approach, we Split the string into an array of characters using split(), then iterate through the array to process each character individually.
Syntax:
str.split('')
Example: In this example we are using above-explained approach.
Javascript
let str = "Geeks" ;
let result = str.split( '' );
result.forEach(char => {
console.log(char);
});
|
Share your thoughts in the comments
Please Login to comment...