Responsive Web Design Using Media Queries
Last Updated :
16 Oct, 2023
Responsive Design is a web design approach that aims to adapt web page layout to any screen from maximum screen sizes like TVs, monitors, etc. to minimum screen sizes like mobiles, etc. with good usability. Media Queries is a CSS3 Feature that makes a website page adapt its layout to different screen sizes and media types. Media queries are CSS rules that apply specific styles based on the characteristics of the user’s device or viewport. These characteristics can include screen width, height, orientation, resolution, and more.
Syntax:
CSS
@media medieType and (condition) {
}
|
The @media rule is used to target media queries and then the media type which is optional depending on the device type and then the condition also called a breakpoint, if the condition is true then the CSS rules are applied to the web page from the parenthesis.
Media Types
Media Types describe the category of device type. Media type is optional and by default, it is considered as `all` type. Media Types are categorized into four types.
- all – for all device types.
- print – for printers
- screen — for smartphones, tablets, TVs and computer screens
- speech — for screen readers that “read” the page out loud
Why to use Media Queries?
Media Queries helps the developers to create a consistent and optimized user experience across all devices. Without responsive design, websites may appear unstable and broken on certain screens which results in poor user experience. With a better user experience, it will be easy to use the product.
Benefits of Responsive Design
- Improves User Experience(UX): Users can access content seamlessly and efficiently on any device. Whether they are on a desktop computer, tablet, or smartphone, the website adapts to provide an optimal and user-friendly experience. This leads to higher user satisfaction and engagement.
- Good SEO: When your website is responsive and provides a consistent experience across devices, it’s more likely to rank higher in search engine results. Responsive designs rank higher and increases traffic.
- Cost-Efficiency: Developing a single website for all platforms, reduces development and maintenance costs. Maintenance and updates are also simplified when you have one codebase to manage.
- Increases Engagements: Users are more likely to stay and interact with a well-designed responsive site. A well-designed responsive site enhances user engagement. When visitors have a positive experience and can easily navigate and interact with your content on their preferred devices, they are more likely to stay on your site.
Elements of a Responsive Design
- Media Queries: Media queries are the foundation of responsive design. They allow you to specify conditions based on characteristics like screen width, height, orientation, and resolution. Declared in CSS using @media followed by conditions. you can apply specific styles to different devices or screen sizes.
- Breakpoints: Specific screen widths where design changes occur. Breakpoints are specific screen widths (or occasionally heights) at which you make design adjustments. These adjustments can include changes in layout, typography, or the visibility of certain elements.
- Flexible Layouts: Use percentage-based or fluid grids and Avoid fixed width and height instead use min-width, max-width, min-height and max-height. CSS Flexbox and CSS Grid are powerful tools for creating flexible layouts.
- Images: Scaling images using CSS or using responsive image techniques. Use CSS to set max-width: 100% for images, preventing them from exceeding their container width.
- Font Sizes: Adjusting font sizes and better font hierarchy for readability on different screens. Font sizes play a vital role in readability and user experience. Consider using relative units like em or rem instead of fixed pixel values for font sizes.
How Does Responsive Design Work?
- Define Breakpoints: Identify key screen widths for design changes mostly mobile (320px — 480px), tablets (481px — 768px), laptops (769px — 1024px), and desktops(1025px — 1200px) these breakpoints are industry standard. But you can customize them based on your project’s requirements.
- Write Media Queries: Use CSS @media to apply styles at breakpoints. They are CSS rules that apply specific styles when certain conditions are met. These conditions are typically based on screen width using the min-width and max-width properties
- Adjust Layouts: To create responsive layouts, use CSS features like Flexbox and CSS Grid. These layout techniques provide a flexible and fluid structure that adapts to different screen sizes.
- Test on Various Devices: Ensure the design works well across different screens using dev tools. Pay attention to user interactions, load times, and accessibility to ensure a seamless experience across all devices.
- Refine as Needed: Continuously optimize and adjust for a seamless user experience. Responsive design is an ongoing process. After testing, gather feedback, and make necessary adjustments to improve the user experience further.
Example of the Main section of a Landing Page using HTML and CSS:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" />
< title >Responsive Design Example</ title >
< link rel = "stylesheet" href = "styles.css" />
</ head >
< body >
< nav >
< div class = "menu-toggle" >
< div class = "bar" ></ div >
< div class = "bar" ></ div >
< div class = "bar" ></ div >
</ div >
< ul class = "menu" >
< li >
</ li >
< li >
</ li >
</ ul >
</ nav >
< div class = "header-tip" >
???? New: Courses, Tutorials, Jobs, Contests and more →</ a >
</ div >
< main >
< section >
< h2 >Responsive Design Example Website!</ h2 >
< p >
Lorem ipsum dolor sit amet consectetur adipisicing elit. Saepe amet
beatae illo fuga cupiditate suscipit, animi quasi
</ p >
< button >Get started</ button >
</ section >
< section id = "img-section" >
< img
src =
alt = "Responsive Design Example"
loading = "lazy"
/>
</ section >
</ main >
< footer >
< div >© 2023 Your GFG Website</ div >
</ footer >
< script >
document.querySelector(".menu-toggle").addEventListener("click", function () {
const menu = document.querySelector(".menu");
menu.classList.toggle("active");
});
</ script >
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
margin : 0 ;
padding : 0 ;
height : 100 vh;
}
nav {
display : flex;
justify- content : center ;
align-items: center ;
background-color : #32cd32 ;
}
main {
display : flex;
justify- content : center ;
align-items: center ;
gap: 30px ;
height : 80 vh;
padding : 64px ;
}
button {
background-color : #32cd32 ;
border : none ;
padding : 12px 24px ;
border-radius: 4px ;
font-size : 16px ;
font-weight : 600 ;
cursor : pointer ;
width : 200px ;
color : white ;
}
section {
max-width : 50% ;
display : flex;
flex- direction : column;
justify- content : center ;
gap: 18px ;
}
img {
width : 500px ;
height : 100% ;
}
h 2 {
font-size : 64px ;
font-weight : 600 ;
margin-bottom : 16px ;
text-align : left ;
}
nav ul {
list-style-type : none ;
padding : 24px ;
margin : 0 ;
}
nav li {
display : inline ;
margin-right : 20px ;
padding : 24px ;
}
nav a {
color : #fff ;
text-decoration : none ;
color : black ;
padding : 2px ;
}
footer {
padding : 12px ;
text-align : center ;
font-size : 12px ;
color : black ;
}
.header-tip {
padding : 64px 0px 0px 64px ;
}
.latest {
text-decoration : none ;
color : black ;
border : 1px solid rgb ( 218 , 218 , 218 );
padding : 8px 12px ;
border-radius: 100px ;
font-size : x-small ;
top : 64px ;
left : 64px ;
}
.menu-toggle {
display : none ;
flex- direction : column;
cursor : pointer ;
padding : 10px ;
}
.menu-toggle .bar {
width : 25px ;
height : 3px ;
background-color : white ;
margin : 3px 0 ;
transition: 0.4 s;
}
.menu {
list-style-type : none ;
padding : 0 ;
margin : 0 ;
display : flex;
flex- direction : row;
}
.menu li {
display : inline ;
margin-right : 20px ;
}
.menu a {
color : #fff ;
text-decoration : none ;
}
@media ( max-width : 480px ) {
body {
font-size : 14px ;
background-color : black ;
color : #fff ;
}
main {
flex- direction : column;
padding : 24px ;
}
.header-tip {
padding : 24px 0px 0px 24px ;
}
.latest {
color : white ;
}
section {
width : 100% ;
padding : 0px ;
display : flex;
justify- content : center ;
align-items: center ;
}
h 2 {
font-size : 32px ;
margin-bottom : 12px ;
text-align : center ;
}
p {
text-align : center ;
}
.menu {
display : none ;
flex- direction : column;
background-color : #32cd32 ;
position : absolute ;
top : 60px ;
right : 0 ;
width : 100% ;
z-index : 1 ;
}
.menu.active {
display : flex;
}
.menu-toggle {
display : flex;
}
nav {
flex- direction : row-reverse;
justify- content : space-between;
background-color : #000 ;
}
#img-section {
display : none ;
}
button {
width : 150px ;
}
footer {
color : white ;
}
}
@media ( min-width : 481px ) and ( max-width : 768px ) {
main {
flex- direction : column;
padding : 40px ;
height : auto ;
}
section {
width : 100% ;
}
header-tip {
padding : 40px 0px 0px 40px ;
}
img {
width : 100% ;
height : 100% ;
}
h 2 {
font-size : 40px ;
margin-bottom : 14px ;
text-align : center ;
}
p {
text-align : center ;
}
footer {
color : white ;
}
button {
width : 100% ;
}
}
@media ( min-width : 769px ) and ( max-width : 1024px ) {
main {
padding : 64px ;
}
h 2 {
font-size : 44px ;
margin-bottom : 16px ;
}
img {
width : 384px ;
height : 100% ;
}
}
|
Output: Click here to see the live output
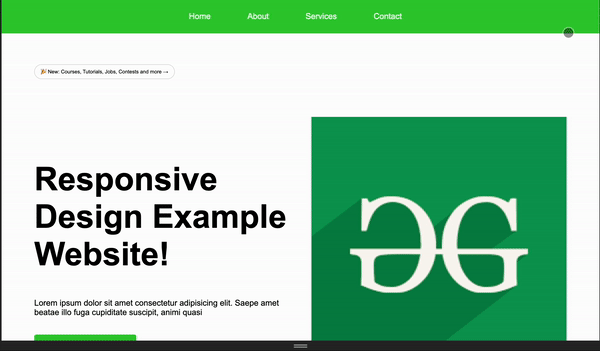
Responsive Design Using CSS Media Queries Example
What Practices to Avoid?
- Overloading with Media Queries: Choose breakpoints judiciously, focusing on the most critical ones for your design. Use a mobile-first approach (starting with mobile styles and adding complexity as needed) or a Desktop-first approach(starting with desktop styles and adding complexity as needed) to minimize the number of breakpoints required.
- Neglecting Mobile-First: Begin the design process with mobile devices in mind. Create a solid foundation for the mobile layout and progressively enhance it for larger screens using media queries. This approach ensures that your design remains functional and user-friendly on all devices.
- Ignoring Performance: Optimize your assets for the web. Compress images, use responsive image techniques, and minify CSS. Additionally, consider using lazy loading images and implementing performance best practices to ensure fast page loads.
- Testing: Test on various devices, browsers, and orientations as necessary. Use developer tools and online emulators to simulate different conditions
- Neglecting Content Priority: Identify the essential content and prioritize it for all screen sizes. Use media queries to reorganize content if necessary, but never sacrifice the clarity and accessibility of your core information.
Conclusion
CSS media queries are indispensable tool for creating responsive web designs. They allow websites to adapt gracefully to different devices, providing a consistent and user-friendly experience. By mastering media queries and following best practices, web developers can ensure their websites are ready for the diverse landscape of the modern digital world.
Share your thoughts in the comments
Please Login to comment...