Replacing Pandas or Numpy Nan with a None to use with MysqlDB
Last Updated :
02 Oct, 2023
The widely used relational database management system is known as MysqlDB. The MysqlDB doesn’t understand and accept the value of ‘Nan’, thus there is a need to convert the ‘Nan’ value coming from Pandas or Numpy to ‘None’. In this article, we will see how we can replace Pandas or Numpy ‘Nan’ with a ‘None’.
Replacing NaN with None in Pandas
Example 1: The data frame, for which ‘Nan’ is to be replaced with ‘None’, is as follows:
The provided code uses the Pandas library to replace ‘NaN’ values in a DataFrame df with ‘None’. It does so by using the replace() method with a dictionary mapping where keys (in this case, ‘np.nan’) are replaced by their corresponding values (in this case, ‘None’). The resulting DataFrame ‘replaced_df’ contains ‘None’ in place of ‘NaN’ values.
Python3
import pandas as pd
import numpy as np
df = pd.DataFrame({ 'A' : [ 1 , np.nan, 3 ], 'B' : [np.nan, 5 , 6 ], 'C' : [ 7 , 8 , np.nan]})
replaced_df = df.replace({np.nan: None })
print (replaced_df)
|
Output:
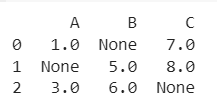
Example 2: The data frame, for which ‘Nan’ is to be replaced with ‘None’, is as follows:
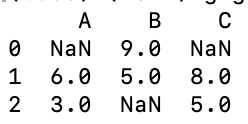
The provided code uses the Pandas library to replace ‘NaN’ values in a DataFrame df with ‘None’. It does so by using the replace() method with a dictionary mapping where keys (in this case, ‘np.nan’) are replaced by their corresponding values (in this case, ‘None’). The resulting DataFrame ‘replaced_df’ contains ‘None’ in place of ‘NaN’ values.
Python3
import pandas as pd
import numpy as np
df = pd.DataFrame({ 'A' : [np.nan, 6 , 3 ], 'B' : [ 9 , 5 , np.nan], 'C' : [np.nan, 8 , 5 ]})
print (df)
replaced_df = df.replace({np.nan: None })
print (replaced_df)
|
Output
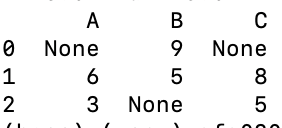
Replacing NaN with None in NumPy
Example 1: The data frame, for which ‘Nan’ is to be replaced with ‘None’ , is as follows:
[ 1. nan 3. 6. 7.]
It creates a NumPy array named temp, replaces the np.nan values with None using np.where, and then prints the modified temp array.
Python3
import numpy as np
temp = np.array([ 1 , np.nan, 3 , 6 , 7 ])
print (arr)
temp = np.where(np.isnan(temp), None , temp)
print (temp)
|
Output:
[1.0 None 3.0 6.0 7.0]
Example 2: The data frame, for which ‘Nan’ is to be replaced with ‘None’ , is as follows:
[ 4. 5. nan nan 7.]
It creates a NumPy array named temp, replaces the np.nan values with None using np.where, and then prints the modified temp array.
Python
import numpy as np
temp = np.array([ 1 , np.nan, 3 , 6 , 7 ])
print (arr)
temp = np.where(np.isnan(temp), None , temp)
print (temp)
|
Output
[4.0 5.0 None None 7.0]
Share your thoughts in the comments
Please Login to comment...