React Suite Popover PropsWhisper methods
Last Updated :
26 Sep, 2023
React Suite is a React Library used to create React components, with intuitive designs and user-friendly experiences. It is designed for both middle platform and back-end products, for server-side rendering. React Suite supports all major web browsers including Chrome, Mozilla Firefox, Safari, and Microsoft Edge.
Popover component is used to display more content when a mouse is clicked or hovered on a pop-up box.
PropsWhisper methods
These whisper methods include:
- open: opens an overlay(e.g. Popover) associated with the Whisper component.
- close: it closes an overlay that is associated with the Whisper component.
- updatePosition: it updates the overlay position within the Whisper component. It is useful when a user wants to manually update the position of the overlay, for example when the content of an overlay changes dynamically and you want to change its position.
Creating React Application And Installing Module:
Step 1: Creating a React App
Create a React Application by running the below command:
npm create vite@latest <projectname>
The above command creates a React-vite application. I prefer Vite over create-react-app because it has a faster runtime.
Step 2: Navigate to the project directory.
Move to the project folder by running the below command:
cd <projectname>
Step 3: Install required modules
Run the below command to create a node_modules directory that will hold all the required libraries and packages with which the project depends on:
npm install
Step 4: Open your project on the editor
After setting up your project using the terminal or command prompt, you now can open your project on your editor. Let’s use VS Code. Run the below command on the terminal/command prompt:
code .
This will open your project on VS code.
Project Structure:
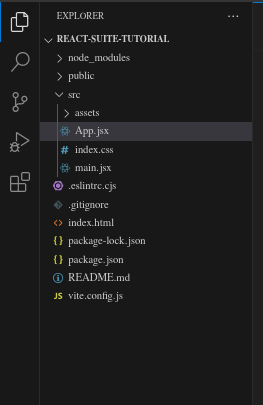
Project Structure when opened in Vs code
Step 5: Running our project
Run the project by using the below command on your terminal or command prompt. This should be on your root directory.
npm run dev
The project is now visible on http://localhost:5173
Example 1: Open App.jsx, delete the template code inside and add the following code:
Javascript
import "rsuite/dist/rsuite.min.css" ;
import { useRef } from "react" ;
import { Button, Popover, Whisper } from "rsuite" ;
export default function App() {
const whisperRef = useRef( null );
const handleclick = () => {
whisperRef.current.open();
setTimeout(() => {
whisperRef.current.close();
}, 3000);
setTimeout(() => {
whisperRef.current.open();
}, 5000);
};
const style = {
display: "flex" ,
flexDirection: "column" ,
alignItems: "center" ,
justifyContent: "center" ,
minHeight: "100vh" ,
justifyItems: "center" ,
};
return (
<div style={style}>
<h1 style={{ color: "green" , font: "bold" }}>
Geeks For Geeks
</h1>
<h3 style={{ font: "bold" }}>
React Suite Popover PropsWhisper methods
</h3>
<Whisper
ref={whisperRef}
trigger= "click"
placement= "bottom"
speaker={
<Popover title= "Title" >
<h5>
This is a sample Popover to show
how methods are used.
</h5>
<p>
You can add the rest of the
content below
</p>
</Popover>
}
>
<Button
appearance= "subtle"
style={{
backgroundColor: "#ff4820" ,
color: "white" ,
}}
onClick={handleclick}
>
Click Here
</Button>
</Whisper>
<br />
<hr />
</div>
);
}
|
Explanation:
- The above code creates a Whisper component which in it contains a Popover overlay.
- The handleClick function uses the open() and close() methods to open and close the overlay after 3 seconds and 5 seconds respectively.
Below is the resultant output:
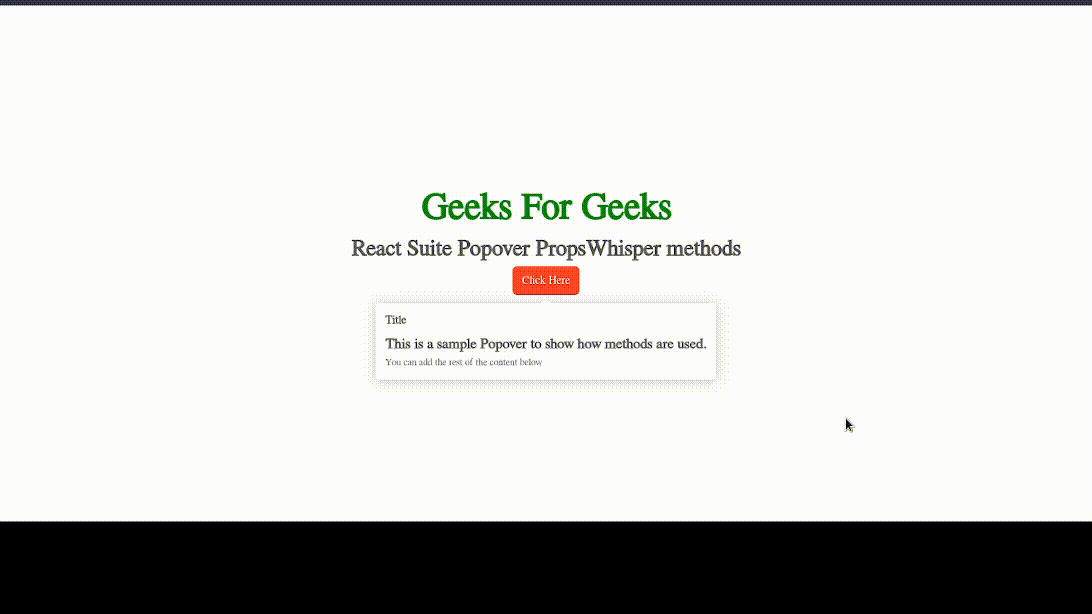
Output
Example 2. Using close() method in a Dropdown overlay
In this example, we will implement close() whisper method on a Dropdown overlay.
Javascript
import { Button, Whisper, Dropdown, Popover } from "rsuite" ;
import "rsuite/dist/rsuite.min.css" ;
import { useRef } from "react" ;
const App = () => {
const whisperRef = useRef( null );
const handleclick = () => {
setTimeout(() => {
whisperRef.current.close();
}, 4000);
};
const styles = {
display: "flex" ,
flexDirection: "column" ,
margin: "auto" ,
alignItems: "center" ,
paddingTop: "100px" ,
minHeight: "100vh" ,
};
return (
<div style={styles}>
<h1 style={{ color: "green" }}>
GeeksforGeeks
</h1>
<h4>React Suite Popover Component</h4> <br />
<Whisper
ref={whisperRef}
trigger= "click"
placement= "bottom"
speaker={
<Popover title= "What is your Best Stack?" >
<Dropdown.Menu>
<Dropdown.Item>
JavaScript
</Dropdown.Item>
<Dropdown.Item>
Python
</Dropdown.Item>
<Dropdown.Item>
Java
</Dropdown.Item>
<Dropdown.Item>
Go
</Dropdown.Item>
</Dropdown.Menu>
</Popover>
}
>
<Button
appearance= "subtle"
style={{
backgroundColor: "#ff4820" ,
color: "white" ,
}}
onClick={handleclick}
>
Click
</Button>
</Whisper>
</div>
);
};
export default App;
|
Output:
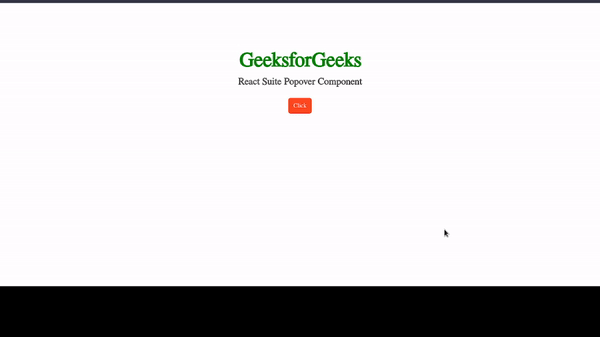
output
Example 3: Using updatePosition method.
Javascript
import { Button, Popover, Whisper } from "rsuite" ;
import "rsuite/dist/rsuite.min.css" ;
import { useRef } from "react" ;
const App = () => {
const whisperRef = useRef( null );
const handleClick = () => {
whisperRef.current.open();
whisperRef.current.updatePosition();
};
const styles = {
display: "flex" ,
flexDirection: "column" ,
margin: "auto" ,
alignItems: "center" ,
paddingTop: "100px" ,
minHeight: "100vh" ,
};
return (
<div style={styles}>
<h1 style={{ color: "green" }}>
Geeks For Geeks
</h1>
<h4>UpdatePosition</h4>
<Whisper
ref={whisperRef}
trigger= "hover"
placement= "bottom"
speaker={
<Popover title= "Sample" >
<h3>Sample Popover</h3>
<p>Rest of the content </p>
</Popover>
}
>
<Button
appearance= "subtle"
onClick={handleClick}
style={{
backgroundColor: "#ff4820" ,
color: "white" ,
}}
>
See Popover
</Button>
</Whisper>
</div>
);
};
export default App;
|
Output:
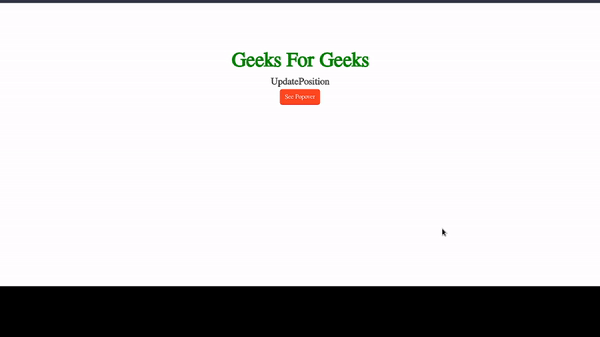
output
Reference: https://rsuitejs.com/components/whisper/#whisper-methods
Share your thoughts in the comments
Please Login to comment...