React Suite Drawer Component
Last Updated :
11 Apr, 2022
React Suite is a popular front-end library with a set of React components that are designed for the middle platform and back-end products. The Drawer component is a panel that slides in from the edge of the screen. We can use the following approach in ReactJS to use the React Suite Drawer Component.
Drawer Props:
- autoFocus: The Drawer is opened and is automatically focused on its own, and it is accessible to screen readers when this is set to true.
- backdrop: The Drawer will display the background when it is opened when this is set to true.
- backdropClassName: It is used to add an optional extra class name to .modal-backdrop.
- classPrefix: It is used to denote the prefix of the component CSS class.
- enforceFocus: The Drawer will prevent the focus from leaving when opened when this is set to true.
- full: It is used to enable the full screen.
- keyboard: It will close Drawer when the ESC key is pressed.
- onEnter: It is a callback function that is triggered before the Drawer transitions in.
- onEntered: It is a callback function that is triggered after the Drawer finishes transitioning in.
- onEntering: It is a callback function that is triggered as the Drawer begins to transition in.
- onExit: It is a callback function that is triggered right before the Drawer transitions out.
- onExited: It is a callback function that is triggered after the Drawer finishes transitioning out.
- onExiting: It is a callback function that is triggered as the Drawer begins to transition out.
- onHide: It is a callback function that is triggered when Drawer hides.
- onShow: It is a callback function that is triggered when the Drawer display.
- placement: It is used for the placement of the Drawer.
- show: It is used to show Drawer.
- size: It is used to set the Drawer size.
Creating React Application And Installing Module:
-
Step 1: Create a React application using the following command:
npx create-react-app foldername
-
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
-
Step 3: After creating the ReactJS application, Install the required module using the following command:
npm install rsuite
Project Structure: It will look like the following.
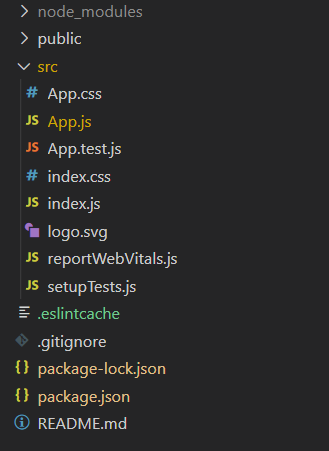
Project Structure
Example: Now write down the following code in the App.js file. Here, App is our default component where we have written our code.
App.js
import React from 'react'
import 'rsuite/dist/styles/rsuite-default.css' ;
import { Drawer, Button } from 'rsuite'
export default function App() {
const [isDrawerOpen, setIsDrawerOpen] = React.useState( false )
const closeDrawer = () => {
setIsDrawerOpen( false );
}
const toggleDrawer = () => {
setIsDrawerOpen( true );
}
return (
<div style={{
display: 'block' , width: 700, paddingLeft: 30
}}>
<h4>React Suite Drawer Component</h4>
<div>
<Button onClick={toggleDrawer}>
Open Drawer
</Button>
<Drawer
show={isDrawerOpen}
onHide={closeDrawer}
>
<Drawer.Header>
<Drawer.Title>Sample Title for Drawer</Drawer.Title>
</Drawer.Header>
<Drawer.Body>
Greetings from GeeksforGeeks!
</Drawer.Body>
<Drawer.Footer>
<Button onClick={closeDrawer} appearance= "primary" >
Confirm
</Button>
<Button onClick={closeDrawer} appearance= "subtle" >
Cancel
</Button>
</Drawer.Footer>
</Drawer>
</div>
</div>
);
}
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
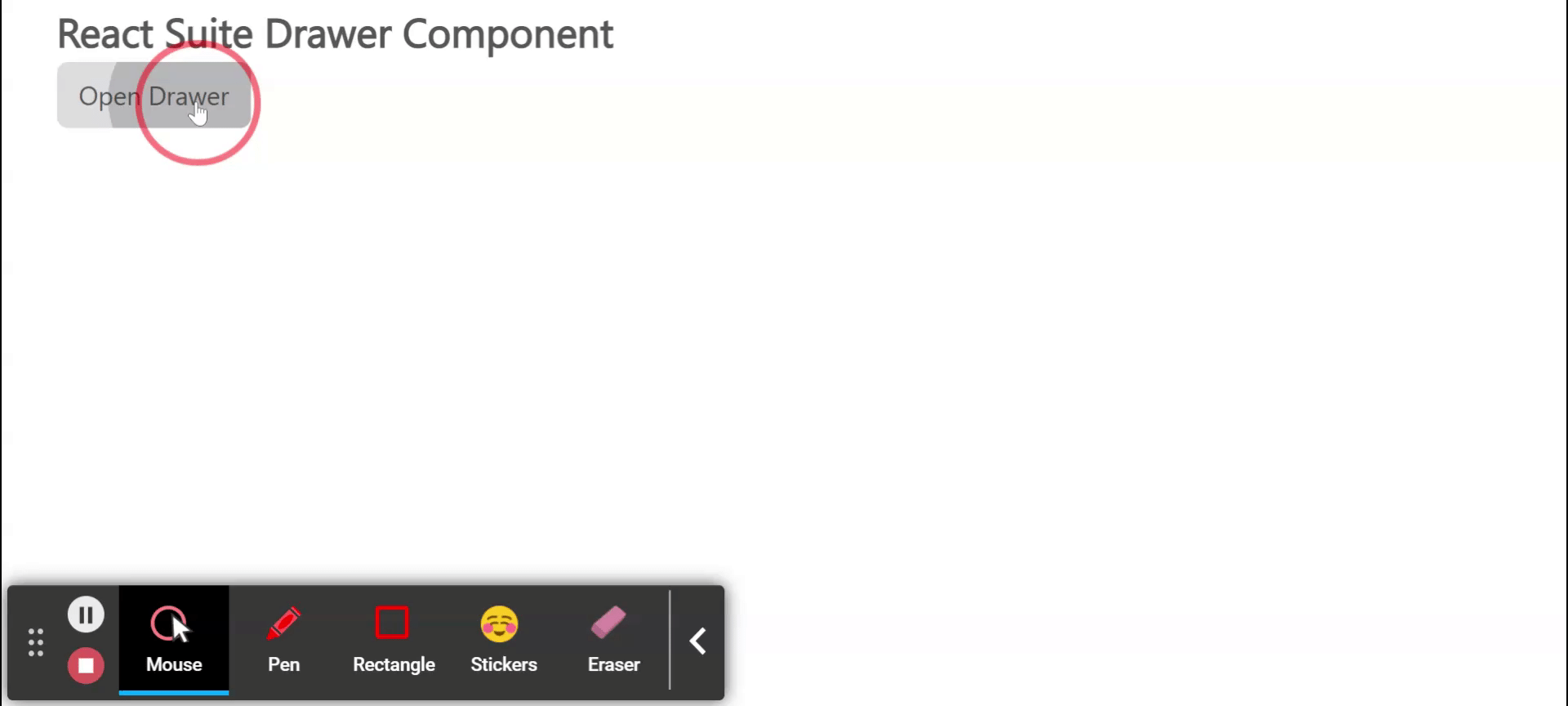
Reference: https://rsuitejs.com/components/drawer/
Share your thoughts in the comments
Please Login to comment...