React Native ProgressBarAndroid
Last Updated :
28 Jun, 2021
In this article, we are going to discuss how to create a Progress Bar for Android applications. We will be using the React Native component ProgressBarAndroid.
Syntax:
<View>
// Other Component
<ProgressBarAndroid/>
// Other Component
<View>
ProgressBarAndroid Props:
- animating: Boolean value which specifies whether to show the progress bar or not.
- color: It specifies the color of the progress bar.
- indeterminate: It is used to show indeterminate progress.
- progress: It specifies the progress value as a number.
- styleAttr: It specifies the style of the ProgressBar Android component. It can have Horizontal, Normal (default), Small, Large, Inverse, SmallInverse, and LargeInverse as value.
- testID: It is used to locate this view in end-to-end tests.
Now let’s start with the implementation:
-
Step 1: Open your terminal and install expo-cli by the following command.
npm install -g expo-cli
-
Step 2: Now create a project by the following command.
expo init ProgressBarAndroidDemo
-
Step 3: Now go into your project folder i.e. ProgressBarAndroidDemo using the following command.
cd ProgressBarAndroidDemo
Project Structure: It will look like the following.
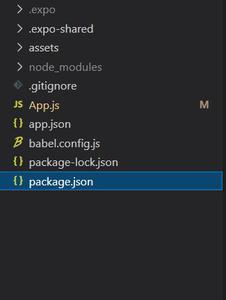
Example: Now let’s implement the ProgressBarAndroid. Here we have shown a simple loader animation, and after it ends, we have shown a text to the user.
App.js
import React, { useState } from 'react' ;
import {
StyleSheet, Text, View, ProgressBarAndroid,
TouchableWithoutFeedback
} from 'react-native' ;
export default function App() {
const [val, setVal] = useState( true );
return (
<View style={styles.container}>
<TouchableWithoutFeedback onPress={() => { setVal( false ); }}
style={{ flex: 1 }}>
<View style={{
flex: 1, justifyContent: 'center' ,
alignItems: 'center'
}}>
<ProgressBarAndroid animating={val} color= "blue"
styleAttr= 'LargeInverse' />
{!val && <Text>App has finished loading</Text>}
</View>
</TouchableWithoutFeedback>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff' ,
alignItems: 'center' ,
justifyContent: 'center' ,
},
});
|
Start the server by using the following command.
npm run android
Output: If your emulator did not open automatically then you need to do it manually. First, go to your android studio and run the emulator. Now start the server again.
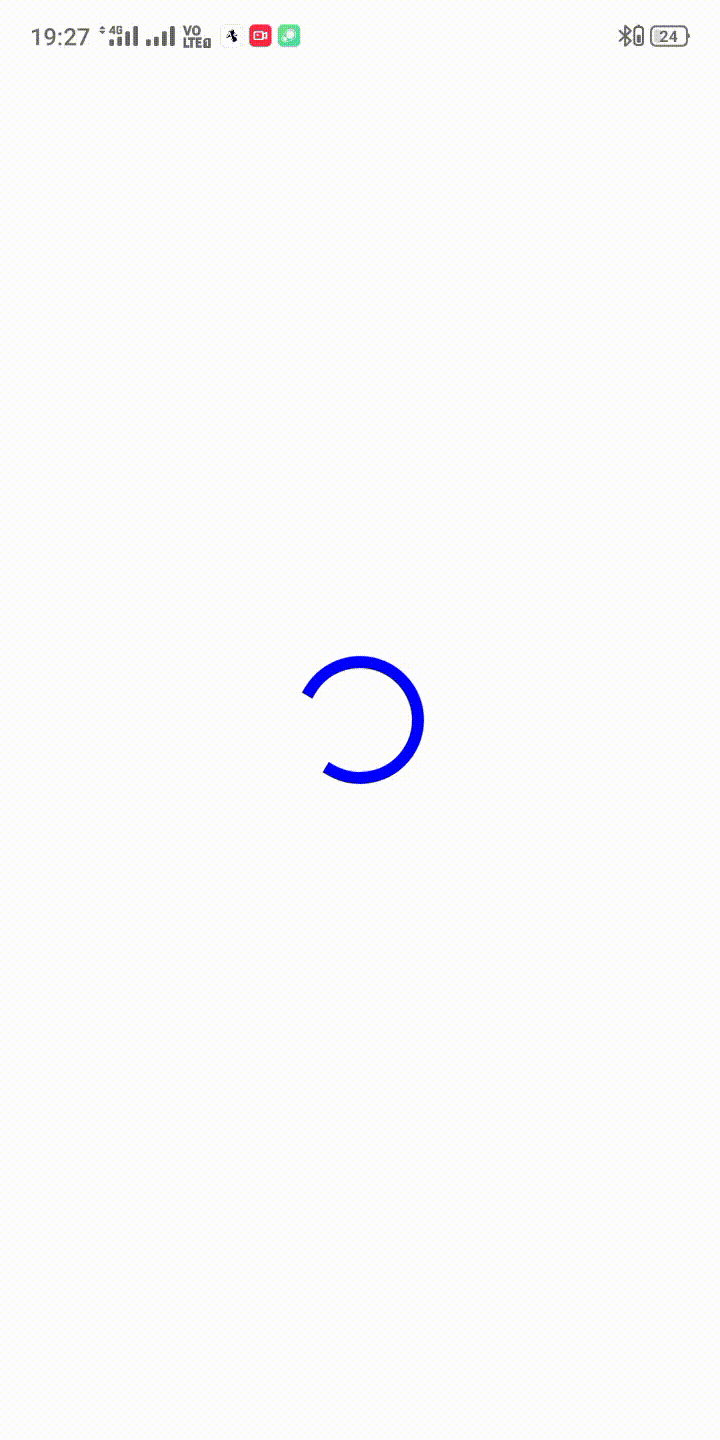
Reference: https://reactnative.dev/docs/progressbarandroid
Share your thoughts in the comments
Please Login to comment...