Python | Writing to an excel file using openpyxl module
Last Updated :
03 May, 2018
Prerequisite : Reading an excel file using openpyxl
Openpyxl
is a Python library for reading and writing Excel (with extension xlsx/xlsm/xltx/xltm) files. The openpyxl module allows Python program to read and modify Excel files.
For example, user might have to go through thousands of rows and pick out few handful information to make small changes based on some criteria. Using Openpyxl module, these tasks can be done very efficiently and easily.
Let’s see how to create and write to an excel-sheet using Python.
Code #1 : Program to print a active sheet title name
import openpyxl
wb = openpyxl.Workbook()
sheet = wb.active
sheet_title = sheet.title
print ( "active sheet title: " + sheet_title)
|
Output :
active sheet title: Sheet
Code #2 : Program to change the Title name
import openpyxl
wb = openpyxl.Workbook()
sheet = wb.active
sheet.title = "sheet1"
print ( "sheet name is renamed as: " + sheet.title)
|
Output :
sheet name is renamed as: sheet1
Code #3 :Program to write to an Excel sheet
import openpyxl
wb = openpyxl.Workbook()
sheet = wb.active
c1 = sheet.cell(row = 1 , column = 1 )
c1.value = "ANKIT"
c2 = sheet.cell(row = 1 , column = 2 )
c2.value = "RAI"
c3 = sheet[ 'A2' ]
c3.value = "RAHUL"
c4 = sheet[ 'B2' ]
c4.value = "RAI"
wb.save( "C:\\Users\\user\\Desktop\\demo.xlsx" )
|
Output :
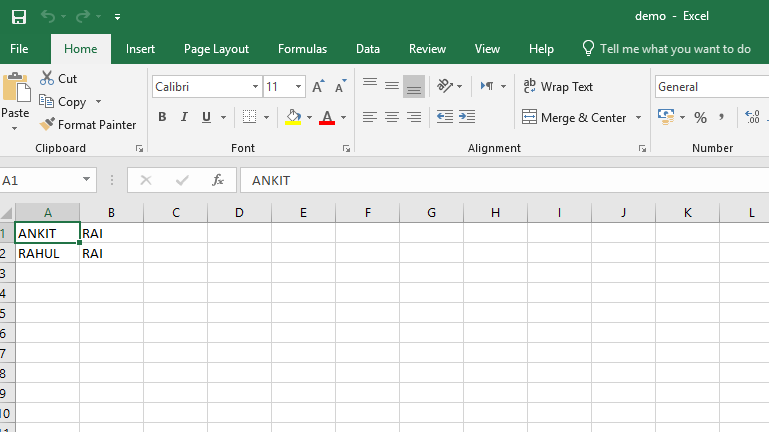
code #4 :Program to add Sheets in the Workbook
import openpyxl
wb = openpyxl.Workbook()
sheet = wb.active
wb.create_sheet(index = 1 , title = "demo sheet2" )
wb.save( "C:\\Users\\user\\Desktop\\demo.xlsx" )
|
Output :
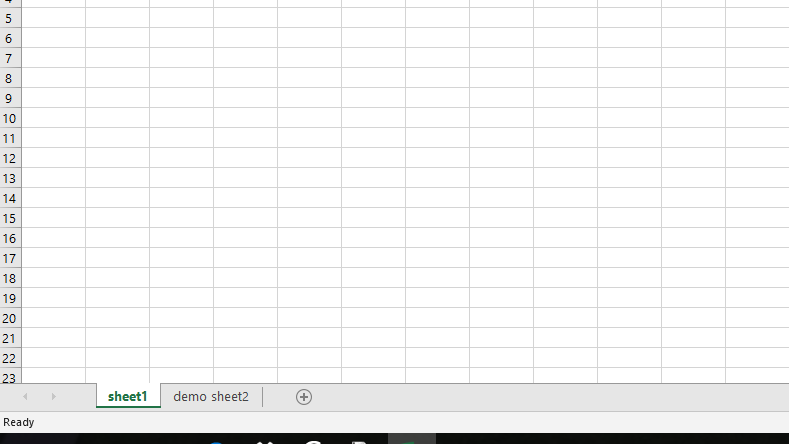
Share your thoughts in the comments
Please Login to comment...