Python Program to Calculate the Area of a Trapezoid
Last Updated :
13 Feb, 2024
A Trapezoid is a convex quadrilateral with at least one pair of parallel sides. The parallel sides are called the bases of the trapezoid and the other two sides which are not parallel are referred to as the legs. There can also be two pairs of bases. In this article, we will see how to find the area of the Trapezoid in Python.
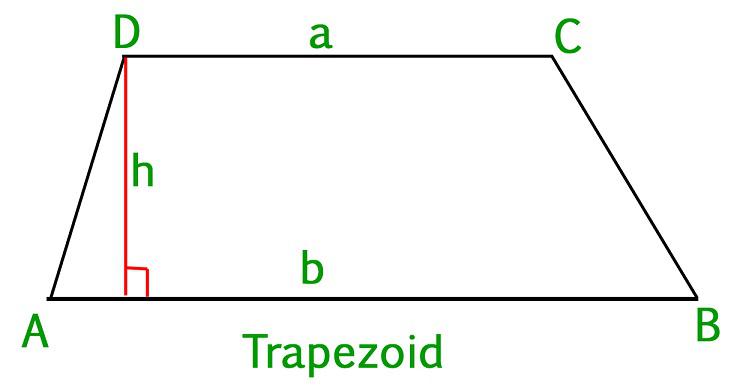
In the above figure CD || AB, so they form the bases and the other two sides i.e., AD and BC form the legs.Â
The area of a trapezoid can be found by using this simple formula :Â
Area = (a+b)/2 * h
Program To Find Area of Trapezoid in Python
Below are some of the ways by which we can find area of Trapezoid in Python:
- Using Formula directly in function
- Using a Class
- Using a Lambda Function
Area Of Trapezoid Using Formula Directly in Function
In this method, we define a function that takes takes three parameters: base1, base2, and height and calculates the area by using the formula (base1 + base2) / 2 * height and returns the result.
Python3
def area_trapezoid(base1, base2, height):
return (base1 + base2) / 2 * height
base1 = 5
base2 = 9
height = 4
result = area_trapezoid(base1, base2, height)
print (f "Area of Trapezoid: {result}" )
|
Output
Area of Trapezoid: 28.0
Area Of Trapezoid Using a Class
In this method, a class is defined to represent a Trapezoid. The class has an __init__ method to initialize base1, base2, and height attributes of the trapezoid object, and a method to calculates the area using the stored values.
Python3
class Trapezoid:
def __init__( self , base1, base2, height):
self .base1 = base1
self .base2 = base2
self .height = height
def calculate_area( self ):
return ( self .base1 + self .base2) / 2 * self .height
trapezoid_instance = Trapezoid( 5 , 9 , 4 )
result = trapezoid_instance.calculate_area()
print (f "Area of Trapezoid: {result}" )
|
Output
Area of Trapezoid: 28.0
Area Of Trapezoid Using a Lambda Function
In this approach, we use lambda() function, to calculate the area the formula (base1 + base2) / 2 * height. Lambda functions are concise, anonymous functions that can be handy for short-lived operations.
Python3
def area_trapezoid_lambda(base1, base2, height): return (
base1 + base2) / 2 * height
base1 = 5
base2 = 9
height = 4
result = area_trapezoid_lambda(base1, base2, height)
print (f "Area of Trapezoid: {result}" )
|
Output
Area of Trapezoid: 28.0
Conclusion
In this article we studied different methods to calculate the area of a trapezoid in Python, each employing distinct approaches and programming paradigms. The choice of method depends on factors such as code readability, project requirements, and the desired level of abstraction. Whether opting for simplicity, object-oriented design, functional programming, or a combination, Python offers the flexibility to choose the method that best suits the specific needs of the application.
Share your thoughts in the comments
Please Login to comment...