Python | Pandas Series.tshift()
Last Updated :
05 Feb, 2019
Pandas series is a One-dimensional ndarray with axis labels. The labels need not be unique but must be a hashable type. The object supports both integer- and label-based indexing and provides a host of methods for performing operations involving the index.
Pandas Series.tshift()
function is used to shift the time index, using the index’s frequency if available. If freq is not specified then, it tries to use the freq or inferred_freq attributes of the index. If neither of those attributes exist, a ValueError is thrown.
Syntax: Series.tshift(periods=1, freq=None, axis=0)
Parameter :
periods : Number of periods to move, can be positive or negative
freq : Increment to use from the tseries module or time rule (e.g. ‘EOM’)
axis : Corresponds to the axis that contains the Index
Returns : shifted : NDFrame
Example #1: Use Series.tshift()
function to shift the Datetime based index of the given series object by certain period.
import pandas as pd
sr = pd.Series([ 'New York' , 'Chicago' , 'Toronto' , 'Lisbon' , 'Rio' , 'Moscow' ])
didx = pd.DatetimeIndex(start = '2014-08-01 10:00' , freq = 'W' ,
periods = 6 , tz = 'Europe/Berlin' )
sr.index = didx
print (sr)
|
Output :
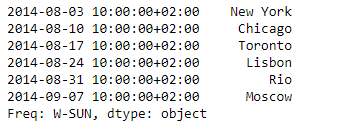
Now we will use Series.tshift()
function to shift the index by 2 periods on the already applied frequency of the series object.
Output :
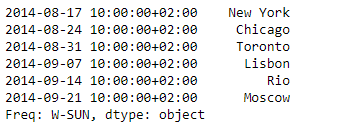
As we can see in the output, the Series.tshift()
function has successfully shifted the DateTime based index of the given series by 2 periods.
Example #2: Use Series.tshift()
function to increment the DateTime based index of the given series object by certain period and also apply ‘Daily’ frequency on it.
import pandas as pd
sr = pd.Series([ 'New York' , 'Chicago' , 'Toronto' , 'Lisbon' , 'Rio' , 'Moscow' ])
didx = pd.DatetimeIndex(start = '2014-08-01 10:00' , freq = 'W' ,
periods = 6 , tz = 'Europe/Berlin' )
sr.index = didx
print (sr)
|
Output :
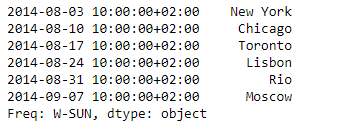
Now we will use Series.tshift()
function to increment the index by 4 periods on the already applied frequency of the series object.
sr.tshift(periods = 4 , freq = 'D' )
|
Output :
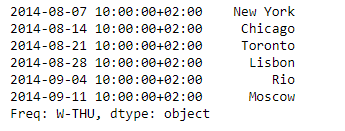
As we can see in the output, the Series.tshift()
function has successfully incremented the DateTime based index of the given series by 4 periods.
Share your thoughts in the comments
Please Login to comment...