Python | Pandas Series.item()
Last Updated :
12 Feb, 2019
Pandas series is a One-dimensional ndarray with axis labels. The labels need not be unique but must be a hashable type. The object supports both integer- and label-based indexing and provides a host of methods for performing operations involving the index.
Pandas Series.item()
function return the first element of the underlying data of the given series object as a python scalar.
Note : This function can only convert an array of size 1 to a Python scalar
Syntax: Series.item()
Parameter : None
Returns : scalar
Example #1: Use Series.item()
function to return the first element of the given series object as a scalar.
import pandas as pd
sr = pd.Series([ 248 ])
index_ = [ 'Coca Cola' ]
sr.index = index_
print (sr)
|
Output :
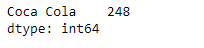
Now we will use Series.item()
function to return the first element of the given series object as a scalar.
result = sr.item()
print (result)
|
Output :

As we can see in the output, the Series.item()
function has successfully returned a scalar value.
Example #2 : Use Series.item()
function to iterate over all the elements in the given series object.
import pandas as pd
sr = pd.Series([ 11 ])
index_ = pd.date_range( '2010-10-09' , periods = 1 , freq = 'M' )
sr.index = index_
print (sr)
|
Output :
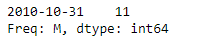
Now we will use Series.item()
function to return the first element of the given series object as a scalar.
result = sr.item()
print (result)
|
Output :
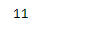
As we can see in the output, the Series.item()
function has successfully returned a scalar value.
Share your thoughts in the comments
Please Login to comment...