Pandas DataFrame to_dict() Method | Convert DataFrame to Dictionary
Last Updated :
09 Feb, 2024
Python is a great language for doing data analysis because of the fantastic ecosystem of data-centric Python packages. Pandas is one of those packages and makes importing and analyzing data much easier.Â
Pandas .to_dict() method is used to convert a DataFrame into a dictionary of series or list-like data type depending on the orient parameter.
Example:
Python3
import pandas as pd
data = { 'Name' : [ 'Alice' , 'Bob' , 'Charlie' ], 'Age' : [ 25 , 30 , 28 ], 'City' : [ 'New York' , 'London' , 'Paris' ]}
df = pd.DataFrame(data)
series_dict = df.to_dict( 'series' )
print (series_dict)
|
Output:
{'Name': 0 Alice
1 Bob
2 Charlie
Name: Name, dtype: object, 'Age': 0 25
1 30
2 28
Name: Age, dtype: int64, 'City': 0 New York
1 London
2 Paris
Name: City, dtype: object}
Syntax
Syntax: DataFrame.to_dict(orient=’dict’, into=)Â
ParametersÂ
- orient: String value, (‘dict’, ‘list’, ‘series’, ‘split’, ‘records’, ‘index’) Defines which dtype to convert Columns(series into). For example, ‘list’ would return a dictionary of lists with Key=Column name and Value=List (Converted series).Â
- into: class, can pass an actual class or instance. For example in case of defaultdict instance of class can be passed. Default value of this parameter is dict.Â
Return type: Dataframe converted into Dictionary
More Examples
To download the data set used in the following example, click here.
In the following examples, the DataFrame used contains data of some NBA players. The image of the data frame before any operations is attached below.
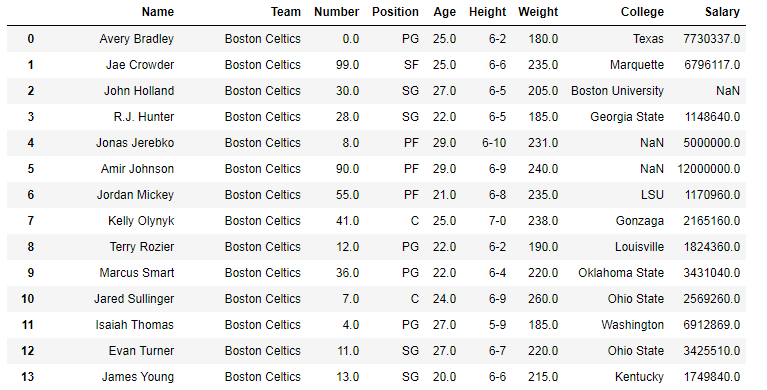
Example 1:
Default conversion of DataFrame into dictionary of Dictionaries. In this case, no parameter is passed to the to_dict() method of the Pandas library. Hence it will convert the DataFrame into a dictionary of dictionaries by default.
Python3
import pandas as pd
data.dropna(inplace = True )
data_dict = data.to_dict()
data_dict
|
Output:
As shown in the output image, the dictionary of dictionaries was returned by the to_dict() method. The key of the first dictionary is the column name and the column is stored with the index as a key of 2nd dictionary.
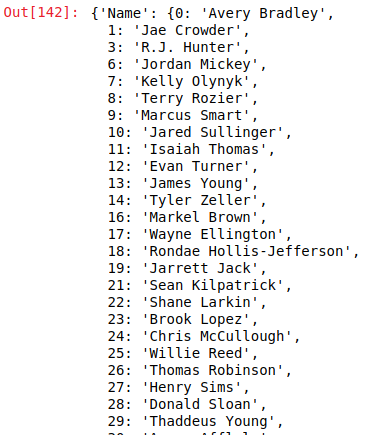
Example 2
Converting DataFrame to Dictionary of Series. The ‘series’ is passed to the orient parameter to convert the data frame into a Dictionary of Series.
Python3
import pandas as pd
data.dropna(inplace = True )
data_dict = data.to_dict( 'series' )
print ( type (data_dict[ 'Name' ]))
data_dict
|
Output:
As shown in the output image, Since the type of data_dict[‘Name’] was pandas.core.series.Series, to_dict() returned a dictionary of series.
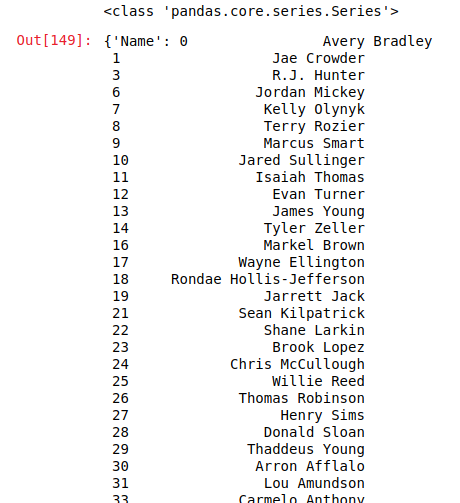
Share your thoughts in the comments
Please Login to comment...