Python | Pandas dataframe.between_time()
Last Updated :
16 Nov, 2018
Python is a great language for doing data analysis, primarily because of the fantastic ecosystem of data-centric python packages. Pandas is one of those packages and makes importing and analyzing data much easier.
Pandas dataframe.between_time()
is used to select values between particular times of the day (e.g. 9:00-9:30 AM). Unlike dataframe.at_time()
function, this function extracts values in a range of time. This function is only used with time-series data. The index of the Dataframe must be DatetimeIndex in order to be able to use this function.
Syntax: DataFrame.between_time(start_time, end_time, include_start=True, include_end=True)
Parameters:
start_time : datetime.time or string
end_time : datetime.time or string
include_start : boolean, default True
include_end : boolean, default True
Returns: values_between_time : type of caller
Note: between_time()
function raises exception when the index of the dataframe is not a DatetimeIndex
Example #1: Use between_time()
function to find the values between a given time interval.
import pandas as pd
ind = pd.date_range( '01/01/2000' , periods = 8 , freq = '30T' )
df = pd.DataFrame({ "A" :[ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 ],
"B" :[ 10 , 20 , 30 , 40 , 50 , 60 , 70 , 80 ]},
index = ind)
df
|
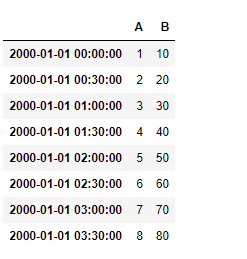
Now let’s query for time between “02:00” to “03:30”
df.between_time( '02:00' , '03:30' )
|
Output :
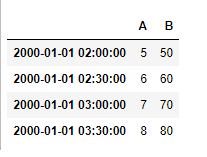
Example #2: Use between_time()
function to find the values between a given time interval while excluding the start and end times.
import pandas as pd
ind = pd.date_range( '01/01/2000' , periods = 8 , freq = '30T' )
df = pd.DataFrame({ "A" :[ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 ],
"B" :[ 10 , 20 , 30 , 40 , 50 , 60 , 70 , 80 ]},
index = ind)
df.between_time( '02:00' , '03:30' , include_start = False ,
include_end = False )
|
Output :
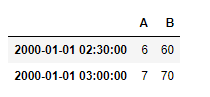
Notice the values corresponding to start time and end time is not included in the dataframe returned by the between_time()
function.
Share your thoughts in the comments
Please Login to comment...