Python MariaDB – Select Query using PyMySQL
Last Updated :
14 Oct, 2020
MariaDB is an open source Database Management System and its predecessor to MySQL. The pymysql client can be used to interact with MariaDB similar to that of MySQL using Python.
In this article we will look into the process of querying data from a table of the database using pymysql. To query data use the following syntax:
Syntax :
- In order to select particular attribute columns from a table, we write the attribute names.
SELECT attr1, attr2 FROM table_name
- In order to select all the attribute columns from a table, we use the asterisk ‘*’ symbol.
SELECT * FROM table_name
Example 1 :
Python3
import pymysql
Host = "localhost"
User = "user"
Password = ""
database = "GFG"
conn = pymysql.connect(host = Host, user = User, password = Password, database)
cur = conn.cursor()
query = f "SELECT * FROM PRODUCT"
cur.execute(query)
rows = cur.fetchall()
conn.close()
for row in rows :
print (row)
|
Output :

output of code
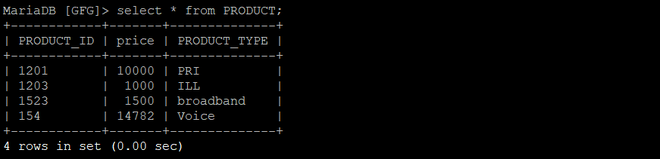
SQL output
The above program illustrates the connection with the MariaDB database ‘GFG‘ in which host-name is localhost, the username is ‘user’ and password is ‘your password’.
Example 2 :
Python3
import pymysql
conn = pymysql.connect( 'localhost' , 'user' , 'password' , 'database' )
cur = conn.cursor()
query = f "SELECT price,PRODUCT_TYPE FROM PRODUCT"
cur.execute(query)
rows = cur.fetchall()
conn.close()
for row in rows :
print (row)
|
Output :
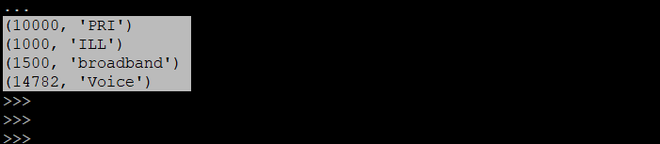
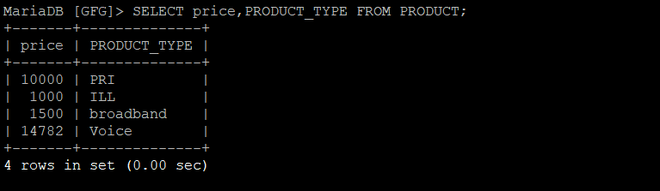
Share your thoughts in the comments
Please Login to comment...