Python Game Development Libraries
Last Updated :
13 Mar, 2024
Python, with its simplicity and versatility, has become a favorite among developers for various applications, including game development. Thanks to its rich ecosystem of libraries and frameworks, creating games with Python has never been easier. In this article, we’ll delve into some of the top Python game development frameworks and provide an overview of tutorials available for each.
Pygame offers a robust and flexible framework for creating games in Python, making it an excellent choice for both beginners and experienced developers alike. Its rich feature set, cross-platform compatibility, and active community support make it a popular choice for game development projects of all sizes and complexities. Here are some of the key features of Pygame:
- Cross-platform compatibility
- Graphics rendering and manipulation
- Sound and music handling
- Input device management
- Event handling system
- Collision detection functionality
- Performance optimization features
- Active community support and documentation
pip install pygame
Example: Creating a Simple Moving Box using Pygame
Python3
import pygame
import sys
# Initialize Pygame
pygame.init()
# Set up the screen
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Pygame Sample")
# Set up colors
BLACK = (0, 0, 0)
RED = (255, 0, 0)
# Set up the initial position and speed of the square
square_size = 50
square_x = (screen_width - square_size) // 2
square_y = (screen_height - square_size) // 2
speed = 5
# Main game loop
running = True
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Key presses handling
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
square_x -= speed
if keys[pygame.K_RIGHT]:
square_x += speed
# Fill the screen with black color
screen.fill(BLACK)
# Draw the red square
pygame.draw.rect(screen, RED, (square_x, square_y, square_size, square_size))
# Update the display
pygame.display.flip()
# Cap the frame rate
pygame.time.Clock().tick(60)
# Quit Pygame
pygame.quit()
sys.exit()
Output
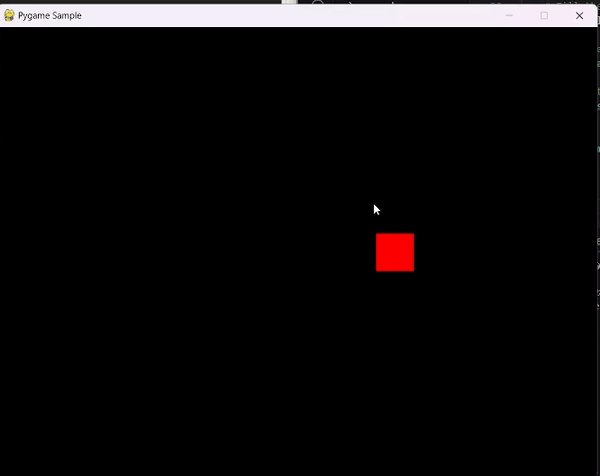
Pyglet
Pyglet is a lightweight, cross-platform library for creating games and multimedia applications in Python. It focuses on providing an easy-to-use interface for handling graphics, audio, and windowing. Here are some of the key features of Pyglet:
- Cross-platform: Pyglet works seamlessly across various operating systems, including Windows, macOS, and Linux.
- Graphics: It provides robust support for rendering graphics, including 2D and 3D graphics, with OpenGL integration.
- Multimedia: Pyglet supports playing audio and video files, making it suitable for multimedia applications and games.
- Windowing: The framework offers windowing support, allowing developers to create and manage windows for their applications.
- Input Handling: Pyglet provides easy-to-use input handling for keyboard, mouse, and joystick events, essential for game development.
pip install pyglet
Example: Creating a Window with “Hello World” with Pyglet
Python3
import pyglet
new_window = pyglet.window.Window()
label = pyglet.text.Label('Hello, World !',
font_name ='Cooper',
font_size = 16,
x = new_window.width//2,
y = new_window.height//2,
anchor_x ='center',
anchor_y ='center')
@new_window.event
def on_draw():
new_window.clear()
label.draw()
pyglet.app.run()
Output
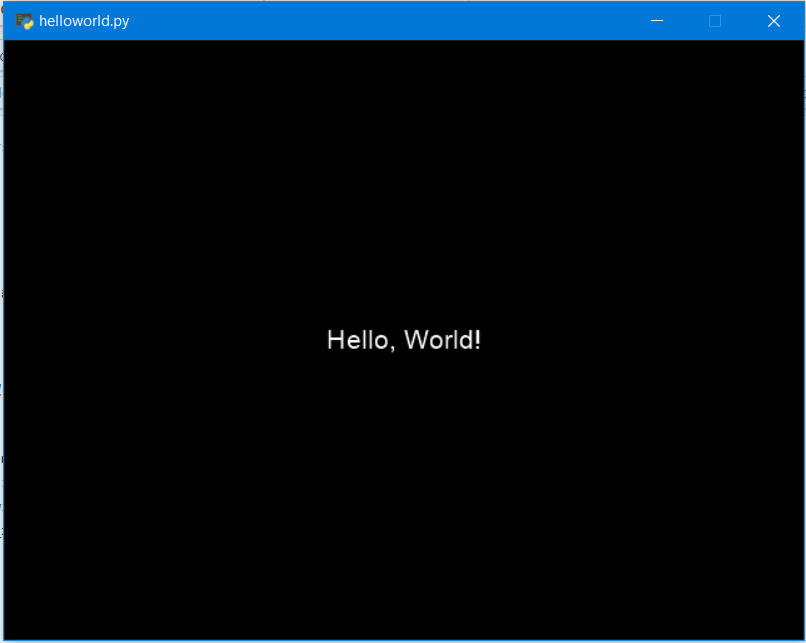
Kivy
Kivy is an open-source Python framework for developing multitouch applications. It’s particularly popular for creating applications with innovative user interfaces that run seamlessly across various platforms, including Windows, macOS, Linux, iOS, and Android. What sets Kivy apart is its emphasis on simplicity, flexibility, and ease of use, making it an attractive choice for both beginner and experienced developers. Here are some of the key features of Pyglet:
- Cross-platform compatibility (Windows, macOS, Linux, iOS, Android)
- Rich set of widgets for creating interactive user interfaces
- Support for multitouch gestures
- Powerful graphics and animation capabilities
- Rapid development with Python language
- Scalable for both simple and complex applications
pip install Kivy
Example: Creating a GUI to handle a Button Click Event using Kivy
Python3
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.label import Label
from kivy.uix.boxlayout import BoxLayout
class MyApp(App):
def build(self):
# Create a layout
layout = BoxLayout(orientation='vertical')
# Create a label
self.label = Label(text="Click the button!")
# Create a button
button = Button(text="Click Me!")
button.bind(on_press=self.on_button_click)
# Add the label and button to the layout
layout.add_widget(self.label)
layout.add_widget(button)
return layout
def on_button_click(self, instance):
self.label.text = "Button clicked!"
if __name__ == '__main__':
MyApp().run()
Output
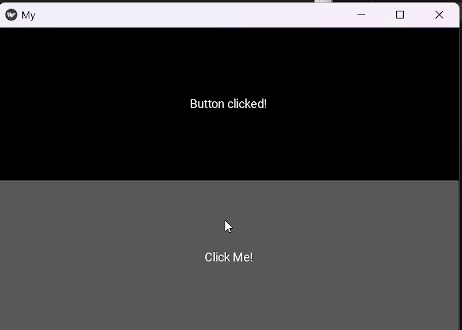
Panda3D
Panda3D is a powerful open-source framework for 3D game development in Python. It offers a range of features such as physics simulation, rendering, and audio support, making it suitable for creating immersive gaming experiences. Here are some of the key features of Panda3D:
- Cross-platform compatibility: Works on Windows, macOS, Linux, and more.
- Powerful rendering engine: Supports advanced rendering techniques like shaders and lighting effects.
- Physics simulation: Built-in physics engine for realistic object interactions.
- Animation support: Easily animate models and characters with keyframes or procedural animation.
- Audio integration: Provides tools for adding 3D sound effects and background music.
- Scene graph management: Hierarchical structure for organizing objects and optimizing rendering.
- Networking capabilities: Built-in networking support for multiplayer games.
Example: Creating a Window
Python3
from direct.showbase.ShowBase import ShowBase
from panda3d.core import PointLight, AmbientLight
from direct.task import Task
class MyApp(ShowBase):
def __init__(self):
ShowBase.__init__(self)
# Load the cube model
self.cube = self.loader.loadModel("models/box")
self.cube.reparentTo(self.render)
self.cube.setScale(0.5, 0.5, 0.5)
self.cube.setPos(0, 0, 0)
# Add a point light to the scene
self.point_light = PointLight('point_light')
self.point_light.setColor((1, 1, 1, 1))
self.point_light_node = self.render.attachNewNode(self.point_light)
self.point_light_node.setPos(5, -5, 7)
self.render.setLight(self.point_light_node)
# Add ambient light to the scene
self.ambient_light = AmbientLight('ambient_light')
self.ambient_light.setColor((0.2, 0.2, 0.2, 1))
self.ambient_light_node = self.render.attachNewNode(self.ambient_light)
self.render.setLight(self.ambient_light_node)
# Rotate the cube
self.taskMgr.add(self.rotateCube, "rotateCubeTask")
def rotateCube(self, task):
angle_degrees = task.time * 20.0
angle_radians = angle_degrees * (3.14159 / 180.0)
self.cube.setHpr(angle_degrees, angle_degrees, angle_degrees)
return Task.cont
app = MyApp()
app.run()
Output
Note: This is an empty program, it won’t do anything.
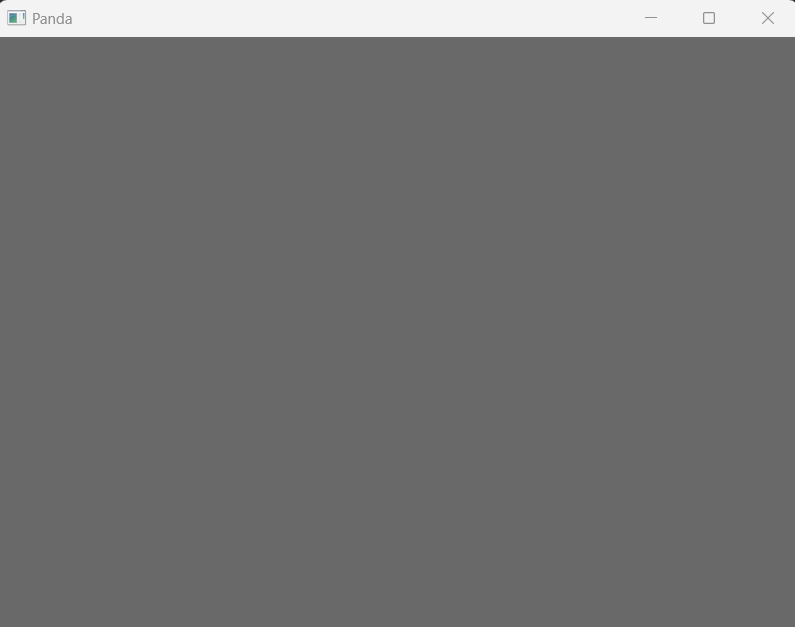
In conclusion, Python offers a wide range of frameworks and libraries for game development, catering to developers of all skill levels and preferences. Whether you’re interested in creating 2D or 3D games, there’s a Python framework out there to suit your needs.
Share your thoughts in the comments
Please Login to comment...