Python Filenotfounderror Winerror 3
Last Updated :
27 Feb, 2024
Python, being a versatile and widely used programming language, encounters various error messages during development. One common error that developers may encounter is the “FileNotFoundError WinError 3.” This error indicates that Python is unable to find the specified file, and the underlying operating system error code is 3, which corresponds to the ‘The system cannot find the path specified’ message on Windows.
What is “Python Filenotfounderror Winerror 3”?
When you try to open, read, or manipulate a file in your Python script, the interpreter may raise a FileNotFoundError if it cannot locate the specified file at the provided path. The “WinError 3” specifically points to an issue related to the file path or the file itself.
Syntax :
Error "Python Filenotfounderror Winerror 3"
Why does FileNotFoundError WinError 3 Occur?
Below, are the reasons of occurring “Python Filenotfounderror Winerror 3” in Python.
- Incorrect File Path
- Insufficient Permissions
- No Such File or Directory Exists
Incorrect File Path
In this example, erroneous file path Python raises the FileNotFoundError if the file path given in the script is invalid or does not point to an existing file or directory.
Python3
try :
file_path = 'C:\\incorrect\\path\\file.txt'
with open (file_path, 'r' ) as file :
content = file .read()
print ( "File content:" , content)
except FileNotFoundError as e:
print ( "File not found:" , e)
except Exception as e:
print ( "An error occurred:" , e)
|
Output
File not found: [Errno 2] No such file or directory: 'C:\\incorrect\\path\\file.txt'
Insufficient Permissions
The file path’s given file or directory may not be accessible to the Python script due to insufficient permissions.
Python3
try :
file_path = 'C:\\restricted\\file.txt'
with open (file_path, 'r' ) as file :
content = file .read()
print ( "File content:" , content)
except FileNotFoundError as e:
print ( "File not found:" , e)
except Exception as e:
print ( "An error occurred:" , e)
|
Output
File not found: [Errno 2] No such file or directory: 'C:\\restricted\\file.txt'
No Such File or Directory Exists
On the system, the requested file or directory might not exist.
Python3
try :
file_path = 'non_existent_file.txt'
with open (file_path, 'r' ) as file :
content = file .read()
print ( "File content:" , content)
except FileNotFoundError as e:
print ( "File not found:" , e)
except Exception as e:
print ( "An error occurred:" , e)
|
Output
File not found: [Errno 2] No such file or directory: 'non_existent_file.txt'
For the “FileNotFoundError WinError 3” error to be successfully troubleshooted and resolved, it is essential to comprehend these fundamental causes.
Fix Filenotfounderror Winerror 3 in Python
below, are the approaches to solve “Python Filenotfounderror Winerror 3”.
Add File Path Correctly
The code attempts to read the content of a file path located at the specified path. If the file is not found, it catches a FileNotFoundError
and prints an error message indicating the issue. To resolve the error, ensure that the file path is correctly specified, making sure it matches the actual location of the file in the system. For any other exception, a general error message is printed.
Python3
try :
file_path = '/content/sample_data/README.md'
with open (file_path, 'r' ) as file :
content = file .read()
print ( "File content:" , content)
except FileNotFoundError as e:
print ( "File not found:" , e)
except Exception as e:
print ( "An error occurred:" , e)
|
Output:
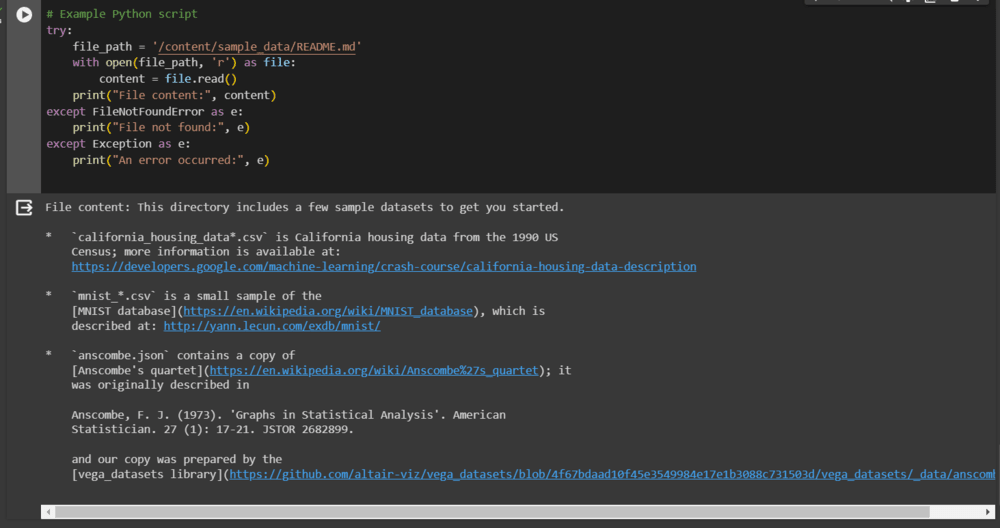
Conclusion:
In conclusion, resolving the “Python FileNotFoundError WinError 3” involves thorough verification of the file path, ensuring correct separators, using absolute paths, and confirming the file’s existence. By addressing these considerations and incorporating appropriate solutions, developers can prevent this error and facilitate seamless file operations in Python.
Share your thoughts in the comments
Please Login to comment...