Python Django Handling Custom Error Page
Last Updated :
02 Oct, 2023
To handle error reporting in Django, you can utilize Django’s built-in form validation mechanisms and Django’s error handling capabilities. In this article, I’ll demonstrate how to implement error handling. If there are errors in the form submission, the user will be notified of the errors.
Required Modules
Python Django Handling Custom Error Page
To start the project use this command
django-admin startproject queryexpressionsproject
cd my_app
To start the app use this command
python manage.py startapp app
Now add this app to the ‘settings.py’
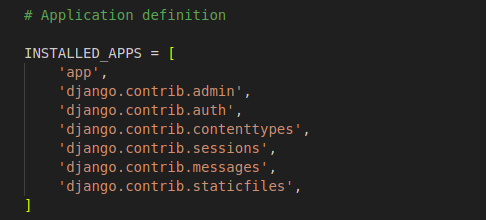
Setting up the Django Files
views.py: This code defines three view functions for a Django web application:
- home(request): Returns a “Hello, World!” response for the root URL.
- error_404(request, exception): Handles HTTP 404 errors (Not Found) and renders a 404 template.
- error_500(request): Handles HTTP 500 errors (Internal Server Error) and renders a 500 template (though there’s a potential typo in the template name).
Python3
from django.http import HttpResponse
from django.shortcuts import redirect, render
from django.db import models
from .models import Product
def home(request):
return HttpResponse( 'Hello, World!' )
def error_404(request, exception):
print (hh)
return render(request, 'myapp/error_404.html' , status = 404 )
def error_500(request):
return render(request, 'myapp/error_505.html' , status = 500 )
|
Setting Up GUI
404.html
Create custom error HTML templates in the templates directory of your app. In this case, let’s create error_404.html
HTML
<!DOCTYPE html>
< html >
< head >
< title >404 Error</ title >
</ head >
< body >
< h1 >Page Not Found (404)</ h1 >
< p >The requested page does not exist.</ p >
</ body >
</ html >
|
505.html
Create custom error HTML templates in the templates directory of your app. In this case, let’s create error_505.html
HTML
<!DOCTYPE html>
< html >
< head >
< title >500 Error</ title >
</ head >
< body >
< h1 >Server Error (500)</ h1 >
< p >Oops! Something went wrong on the server.</ p >
</ body >
</ html >
|
app/urls.py: This file is used to define the URLs inside the app.
Python3
from django.urls import path
from . import views
urlpatterns = [
path( 'hello/' , views.home, name = 'home' ),
]
|
urls.py: We set handler404 and handler500 to the custom error view functions we defined earlier (error_404 and error_500).
Now, when a 404 or 500 error occurs in your Django project, Django will use the custom error views and render the corresponding custom error pages you’ve defined.
Python3
from django.contrib import admin
from django.urls import path, include
handler404 = 'my_app.views.error_404'
handler500 = 'my_app.views.error_500'
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' my_app.urls')),
]
|
Things to Remember
If you’re seeing the default Django 404 page instead of your custom error_404.html template, it could be due to a few reasons. To ensure your custom 404 error page is displayed, please check the following:
- Template Location: Verify that your error_404.html template is placed in the correct directory structure within your app’s templates. The correct path should be products/templates/products/error_404.html. Ensure that the template file name and location are spelled correctly and match the app’s name.
- App Name: Confirm that the name of your app is indeed “products” as it appears in the template path and in the handler404 setting.
- Case Sensitivity: Ensure that the file and directory names in your file system are using the correct case sensitivity. On some systems, file and directory names are case-sensitive, so make sure they match exactly.
- Restart Server: After adding or modifying template files, sometimes you may need to restart your development server for changes to take effect. Stop the server (Ctrl+C) and then start it again with python manage.py runserver.
- Debug Mode: The message you provided suggests that you are running your project in Debug mode (DEBUG = True in your Django settings). In Debug mode, Django might still display its default error pages. Custom error pages are typically shown when DEBUG is set to False in your settings.
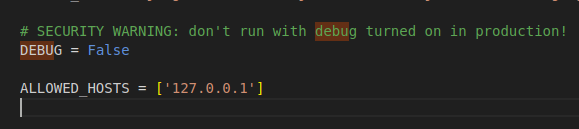
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
As you can see that if we write correct URLs we can see the hello world page.
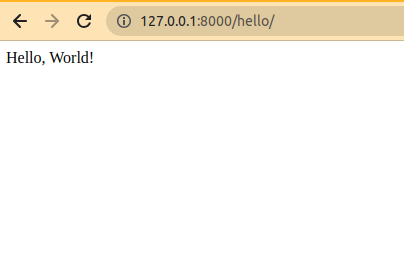
Now you can see that we written wrong url and error page occured.
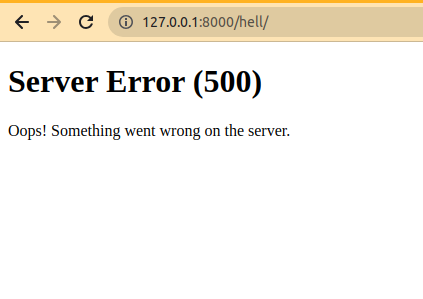
Share your thoughts in the comments
Please Login to comment...