Program to Convert Hexadecimal Number to Binary
Last Updated :
29 Apr, 2023
Given a Hexadecimal number as an input, the task is to convert that number to a Binary number.
Examples:
Input: Hexadecimal = 1AC5
Output: Binary = 0001101011000101
Explanation:
Equivalent binary value of 1: 0001
Equivalent binary value of A: 1010
Equivalent binary value of C: 1100
Equivalent binary value of 5: 0101
Input: Hexadecimal = 5D1F
Output: Binary = 0101110100011111
Approach: A hexadecimal number is a positional numeral system with a radix, or base, of 16 and uses sixteen distinct symbols.
A binary number is a number expressed in the base-2 binary numeral system, which uses only two symbols: which are 0 (zero) and 1 (one).
To convert HexaDecimal number to Binary, the binary equivalent of each digit of the HexaDecimal number is evaluated and combined at the end to get the equivalent binary number.
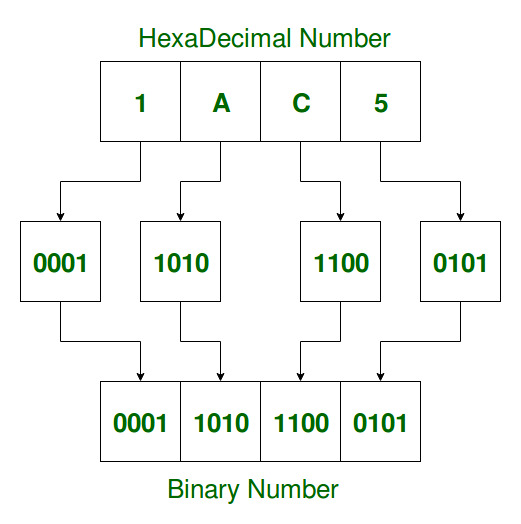
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void HexToBin(string hexdec)
{
size_t i = (hexdec[1] == 'x' || hexdec[1] == 'X' )? 2 : 0;
while (hexdec[i]) {
switch (hexdec[i]) {
case '0' :
cout << "0000" ;
break ;
case '1' :
cout << "0001" ;
break ;
case '2' :
cout << "0010" ;
break ;
case '3' :
cout << "0011" ;
break ;
case '4' :
cout << "0100" ;
break ;
case '5' :
cout << "0101" ;
break ;
case '6' :
cout << "0110" ;
break ;
case '7' :
cout << "0111" ;
break ;
case '8' :
cout << "1000" ;
break ;
case '9' :
cout << "1001" ;
break ;
case 'A' :
case 'a' :
cout << "1010" ;
break ;
case 'B' :
case 'b' :
cout << "1011" ;
break ;
case 'C' :
case 'c' :
cout << "1100" ;
break ;
case 'D' :
case 'd' :
cout << "1101" ;
break ;
case 'E' :
case 'e' :
cout << "1110" ;
break ;
case 'F' :
case 'f' :
cout << "1111" ;
break ;
case '.' :
cout << "." ;
break ;
default :
cout << "\nInvalid hexadecimal digit "
<< hexdec[i];
}
i++;
}
}
int main()
{
char hexdec[100] = "1AC5" ;
cout << "\nEquivalent Binary value is : " ;
HexToBin(hexdec);
return 0;
}
|
C
#include <stdio.h>
void HexToBin( char * hexdec)
{
size_t i = (hexdec[1] == 'x' || hexdec[1] == 'X' )? 2 : 0;
while (hexdec[i]) {
switch (hexdec[i]) {
case '0' :
printf ( "0000" );
break ;
case '1' :
printf ( "0001" );
break ;
case '2' :
printf ( "0010" );
break ;
case '3' :
printf ( "0011" );
break ;
case '4' :
printf ( "0100" );
break ;
case '5' :
printf ( "0101" );
break ;
case '6' :
printf ( "0110" );
break ;
case '7' :
printf ( "0111" );
break ;
case '8' :
printf ( "1000" );
break ;
case '9' :
printf ( "1001" );
break ;
case 'A' :
case 'a' :
printf ( "1010" );
break ;
case 'B' :
case 'b' :
printf ( "1011" );
break ;
case 'C' :
case 'c' :
printf ( "1100" );
break ;
case 'D' :
case 'd' :
printf ( "1101" );
break ;
case 'E' :
case 'e' :
printf ( "1110" );
break ;
case 'F' :
case 'f' :
printf ( "1111" );
break ;
case '.' :
printf ( "." );
default :
printf ( "\nInvalid hexadecimal digit %c" ,
hexdec[i]);
}
i++;
}
}
int main()
{
char hexdec[100] = "1AC5" ;
printf ( "\nEquivalent Binary value is : " );
HexToBin(hexdec);
}
|
Java
public class Improve {
static void HexToBin( char hexdec[])
{
int i = 0 ;
while (hexdec[i] != '\u0000' ) {
switch (hexdec[i]) {
case '0' :
System.out.print( "0000" );
break ;
case '1' :
System.out.print( "0001" );
break ;
case '2' :
System.out.print( "0010" );
break ;
case '3' :
System.out.print( "0011" );
break ;
case '4' :
System.out.print( "0100" );
break ;
case '5' :
System.out.print( "0101" );
break ;
case '6' :
System.out.print( "0110" );
break ;
case '7' :
System.out.print( "0111" );
break ;
case '8' :
System.out.print( "1000" );
break ;
case '9' :
System.out.print( "1001" );
break ;
case 'A' :
case 'a' :
System.out.print( "1010" );
break ;
case 'B' :
case 'b' :
System.out.print( "1011" );
break ;
case 'C' :
case 'c' :
System.out.print( "1100" );
break ;
case 'D' :
case 'd' :
System.out.print( "1101" );
break ;
case 'E' :
case 'e' :
System.out.print( "1110" );
break ;
case 'F' :
case 'f' :
System.out.print( "1111" );
case '.' :
System.out.print( "." );
break ;
default :
System.out.print( "\nInvalid hexadecimal digit " + hexdec[i]);
}
i++;
}
}
public static void main(String args[])
{
String s = "1AC5" ;
char hexdec[] = new char [ 100 ] ;
hexdec = s.toCharArray() ;
System.out.print( "\nEquivalent Binary value is : " );
try {
HexToBin(hexdec);
}
catch (ArrayIndexOutOfBoundsException e){
System.out.print( "" );
}
}
}
|
Python3
def HexToBin(hexdec):
for i in hexdec:
if i = = '0' :
print ( '0000' , end = '')
elif i = = '1' :
print ( '0001' , end = '')
elif i = = '2' :
print ( '0010' , end = '')
elif i = = '3' :
print ( '0011' , end = '')
elif i = = '4' :
print ( '0100' , end = '')
elif i = = '5' :
print ( '0101' , end = '')
elif i = = '6' :
print ( '0110' , end = '')
elif i = = '7' :
print ( '0111' , end = '')
elif i = = '8' :
print ( '1000' , end = '')
elif i = = '9' :
print ( '1001' , end = '')
elif i = = 'A' or i = = 'a' :
print ( '1010' , end = '')
elif i = = 'B' or i = = 'b' :
print ( '1011' , end = '')
elif i = = 'C' or i = = 'c' :
print ( '1100' , end = '')
elif i = = 'D' or i = = 'd' :
print ( '1101' , end = '')
elif i = = 'E' or i = = 'e' :
print ( '1110' , end = '')
elif i = = 'F' or i = = 'f' :
print ( '1111' , end = '')
elif i = = '.' :
print ( '.' , end = '')
else :
print ( "\nInvalid hexadecimal digit " +
str (hexdec[i]), end = '')
if __name__ = = "__main__" :
hexdec = "1AC5" ;
print ( "Equivalent Binary value is : " ,
end = '')
HexToBin(hexdec)
|
C#
class GFG
{
static void HexToBin( char [] hexdec)
{
int i = 0;
while (hexdec[i] != '\u0000' )
{
switch (hexdec[i])
{
case '0' :
System.Console.Write( "0000" );
break ;
case '1' :
System.Console.Write( "0001" );
break ;
case '2' :
System.Console.Write( "0010" );
break ;
case '3' :
System.Console.Write( "0011" );
break ;
case '4' :
System.Console.Write( "0100" );
break ;
case '5' :
System.Console.Write( "0101" );
break ;
case '6' :
System.Console.Write( "0110" );
break ;
case '7' :
System.Console.Write( "0111" );
break ;
case '8' :
System.Console.Write( "1000" );
break ;
case '9' :
System.Console.Write( "1001" );
break ;
case 'A' :
case 'a' :
System.Console.Write( "1010" );
break ;
case 'B' :
case 'b' :
System.Console.Write( "1011" );
break ;
case 'C' :
case 'c' :
System.Console.Write( "1100" );
break ;
case 'D' :
case 'd' :
System.Console.Write( "1101" );
break ;
case 'E' :
case 'e' :
System.Console.Write( "1110" );
break ;
case 'F' :
case 'f' :
System.Console.Write( "1111" );
break ;
case '.' :
System.Console.Write( '.' );
break ;
default :
System.Console.Write( "\nInvalid hexadecimal digit " +
hexdec[i]);
break ;
}
i++;
}
}
static void Main()
{
string s = "1AC5" ;
char [] hexdec = new char [100];
hexdec = s.ToCharArray();
System.Console.Write( "Equivalent Binary value is : " );
try
{
HexToBin(hexdec);
}
catch (System.IndexOutOfRangeException)
{
System.Console.Write( "" );
}
}
}
|
PHP
<?php
function HexToBin( $hexdec )
{
$i = 0;
while ( $hexdec [ $i ])
{
switch ( $hexdec [ $i ])
{
case '0' :
echo "0000" ;
break ;
case '1' :
echo "0001" ;
break ;
case '2' :
echo "0010" ;
break ;
case '3' :
echo "0011" ;
break ;
case '4' :
echo "0100" ;
break ;
case '5' :
echo "0101" ;
break ;
case '6' :
echo "0110" ;
break ;
case '7' :
echo "0111" ;
break ;
case '8' :
echo "1000" ;
break ;
case '9' :
echo "1001" ;
break ;
case 'A' :
case 'a' :
echo "1010" ;
break ;
case 'B' :
case 'b' :
echo "1011" ;
break ;
case 'C' :
case 'c' :
echo "1100" ;
break ;
case 'D' :
case 'd' :
echo "1101" ;
break ;
case 'E' :
case 'e' :
echo "1110" ;
break ;
case 'F' :
case 'f' :
echo "1111" ;
break ;
case '.' :
echo "." ;
break ;
default :
echo "\nInvalid hexadecimal digit " .
$hexdec [ $i ];
}
$i ++;
}
}
$hexdec = "1AC5" ;
echo "\nEquivalent Binary value is : " ;
HexToBin( $hexdec );
|
Javascript
<script>
function HexToBin(hexdec)
{
var i = 0;
while (hexdec[i]) {
switch (hexdec[i]) {
case '0' :
document.write( "0000" );
break ;
case '1' :
document.write( "0001" );
break ;
case '2' :
document.write( "0010" );
break ;
case '3' :
document.write( "0011" );
break ;
case '4' :
document.write( "0100" );
break ;
case '5' :
document.write( "0101" );
break ;
case '6' :
document.write( "0110" );
break ;
case '7' :
document.write( "0111" );
break ;
case '8' :
document.write( "1000" );
break ;
case '9' :
document.write( "1001" );
break ;
case 'A' :
case 'a' :
document.write( "1010" );
break ;
case 'B' :
case 'b' :
document.write( "1011" );
break ;
case 'C' :
case 'c' :
document.write( "1100" );
break ;
case 'D' :
case 'd' :
document.write( "1101" );
break ;
case 'E' :
case 'e' :
document.write( "1110" );
break ;
case 'F' :
case 'f' :
document.write( "1111" );
break ;
case '.' :
document.write( "." );
break ;
default :
document.write( "\nInvalid hexadecimal digit " + hexdec[i]);
}
i++;
}
}
var hexdec = "1AC5" ;
document.write( "\nEquivalent Binary value is : " );
HexToBin(hexdec);
</script>
|
Output:
Equivalent Binary value is : 0001101011000101
Time Complexity: O(n)
Auxiliary Space: O(1)
Alternate Approach :
C++
#include <bits/stdc++.h>
using namespace std;
string hexToBinary(string input)
{
unsigned int x = stoul(input, nullptr, 16) ;
string result = bitset<16>(x).to_string();
return result;
}
int main() {
string s = "1AC5" ;
string result = hexToBinary(s);
cout << result;
return 0;
}
|
Java
import java.io.*;
class HexToBinary {
public static void main(String[] args)
{
String s = "1AC5" ;
String result = hexToBinary(s);
System.out.println(result);
}
private static String hexToBinary(String input)
{
int decimalValue = Integer.parseInt(input, 16 );
String result
= Integer.toBinaryString(decimalValue);
return result;
}
}
|
Python
def to4DigitBin(value):
return '0' * ( 4 - len (value)) + value
def HexadecimalToBinary(inputHexadecimal):
resultBinary = ''
for eachElement in inputHexadecimal:
if (eachElement.isdigit()):
binaryOfSingleDigit = bin ( int (eachElement))[ 2 :]
resultBinary + = to4DigitBin(binaryOfSingleDigit)
elif (eachElement.isalpha() and ord (eachElement) < 71 ):
resultBinary + = to4DigitBin( bin ( ord (eachElement) - 55 )[ 2 :])
else :
resultBinary = 'Invalid hexadecimal digit: ' + eachElement
break
return 'Equivalent Binary value is: ' + resultBinary
inputHexadecimal = '1AC5'
print (HexadecimalToBinary(inputHexadecimal))
|
C#
using System;
class HexToBinary {
public static void Main( string [] args)
{
string s = "1AC5" ;
string result = hexToBinary(s);
Console.WriteLine(result);
}
private static string hexToBinary( string input)
{
int decimalValue = Convert.ToInt32(input, 16);
string result = Convert.ToString(decimalValue, 2);
return result;
}
}
|
Javascript
function hexToBinary(input)
{
var decimalValue = parseInt(input, 16);
var result = decimalValue.toString(2);
var len = 4 * (Math.floor(result.length / 4) + (result.length % 4 ? 1 : 0));
return result.padStart(len, '0' );
}
var s = "1AC5" ;
var result = hexToBinary(s);
console.log(result);
console.log( "0001101011000101" );
|
Output
Equivalent Binary value is: 0001101011000101
Time Complexity: O(1)
Auxiliary Space: O(1)
Method 3: Manual Conversion
1. The function first defines a dictionary called hex_to_bin_dict, which maps each hexadecimal digit to its binary representation. This is done using a series of key-value pairs in the format ‘hex_digit’: ‘binary_digit’.
2. The input hexadecimal number is converted to uppercase using the upper() method to avoid issues with lowercase letters.
3. The function then creates an empty string called bin_num, which will be used to store the binary representation of the input hexadecimal number.
4. For each digit in the input hexadecimal number, the corresponding binary digit is retrieved from the hex_to_bin_dict dictionary using square bracket notation, and added to the bin_num string. This process is repeated for each digit in the input hexadecimal number.
Finally, the function returns the binary representation of the input hexadecimal number as a string.
Python3
def hex_to_bin(hex_num):
hex_to_bin_dict = {
'0' : '0000' , '1' : '0001' , '2' : '0010' , '3' : '0011' ,
'4' : '0100' , '5' : '0101' , '6' : '0110' , '7' : '0111' ,
'8' : '1000' , '9' : '1001' , 'A' : '1010' , 'B' : '1011' ,
'C' : '1100' , 'D' : '1101' , 'E' : '1110' , 'F' : '1111'
}
hex_num = hex_num.upper()
bin_num = ''
for digit in hex_num:
bin_num + = hex_to_bin_dict[digit]
return bin_num
hex_num = "1AC5"
print (hex_to_bin(hex_num))
|
Java
public class HexToBin {
public static void main(String[] args) {
String hex_num = "1AC5" ;
String bin_num = hexToBin(hex_num);
System.out.println(bin_num);
}
public static String hexToBin(String hex_num) {
hex_num = hex_num.toUpperCase();
String hex_to_bin_dict[] = {
"0000" , "0001" , "0010" , "0011" ,
"0100" , "0101" , "0110" , "0111" ,
"1000" , "1001" , "1010" , "1011" ,
"1100" , "1101" , "1110" , "1111"
};
String bin_num = "" ;
for ( int i = 0 ; i < hex_num.length(); i++) {
bin_num += hex_to_bin_dict[Character.digit(hex_num.charAt(i), 16 )];
}
return bin_num;
}
}
|
C++
#include <iostream>
#include <string>
#include <unordered_map>
std::string hex_to_bin(std::string hex_num) {
std::unordered_map< char , std::string> hex_to_bin_dict {
{ '0' , "0000" }, { '1' , "0001" }, { '2' , "0010" }, { '3' , "0011" },
{ '4' , "0100" }, { '5' , "0101" }, { '6' , "0110" }, { '7' , "0111" },
{ '8' , "1000" }, { '9' , "1001" }, { 'A' , "1010" }, { 'B' , "1011" },
{ 'C' , "1100" }, { 'D' , "1101" }, { 'E' , "1110" }, { 'F' , "1111" }
};
std::string bin_num;
for ( char digit : hex_num) {
bin_num += hex_to_bin_dict[std:: toupper (digit)];
}
return bin_num;
}
int main() {
std::string hex_num = "1AC5" ;
std::cout << hex_to_bin(hex_num) << std::endl;
return 0;
}
|
C#
using System;
using System.Collections.Generic;
class Program {
static string HexToBin( string hexNum) {
Dictionary< char , string > hexToBinDict = new Dictionary< char , string >() {
{ '0' , "0000" }, { '1' , "0001" }, { '2' , "0010" }, { '3' , "0011" },
{ '4' , "0100" }, { '5' , "0101" }, { '6' , "0110" }, { '7' , "0111" },
{ '8' , "1000" }, { '9' , "1001" }, { 'A' , "1010" }, { 'B' , "1011" },
{ 'C' , "1100" }, { 'D' , "1101" }, { 'E' , "1110" }, { 'F' , "1111" }
};
string binNum = "" ;
foreach ( char digit in hexNum.ToUpper()) {
binNum += hexToBinDict[digit];
}
return binNum;
}
static void Main( string [] args) {
string hexNum = "1AC5" ;
Console.WriteLine(HexToBin(hexNum));
}
}
|
Javascript
function hexToBin(hexNum) {
const hexToBinDict = {
'0' : '0000' , '1' : '0001' , '2' : '0010' , '3' : '0011' ,
'4' : '0100' , '5' : '0101' , '6' : '0110' , '7' : '0111' ,
'8' : '1000' , '9' : '1001' , 'A' : '1010' , 'B' : '1011' ,
'C' : '1100' , 'D' : '1101' , 'E' : '1110' , 'F' : '1111'
};
hexNum = hexNum.toUpperCase();
let binNum = '' ;
for (let i = 0; i < hexNum.length; i++) {
const digit = hexNum[i];
binNum += hexToBinDict[digit];
}
return binNum;
}
const hexNum = "1AC5" ;
console.log(hexToBin(hexNum));
|
Time complexity: O(n)
Auxiliary space: O(n)
Share your thoughts in the comments
Please Login to comment...