Printing Lists as Tabular Data in Python
Last Updated :
25 Dec, 2022
During the presentation of data, the question arises as to why the data has to be presented in the form of a table. Tabular data refers to the data that is stored in the form of rows and columns i.e., in the form of a table. It is often preferred to store data in tabular form as data appears more organized and systematic. We are going to illustrate a few of them in this article.
Tabulation of data facilitates the comparison and analysis of data easily as compared to the presentation of raw data. Thus, we prefer to tabulate data instead of printing them as lists. For this purpose, we use the in-built modules offered by Python. There are many modules available in Python to print data in tabular form.
Example
Objects are stored in lists in horizontal format thus, we need some methods to present them in tabular format or dropdown form.
Input: ['Fruit', 'Price/Kg'], ['Apple', 25], ['Banana', 20]]
Output:
+--------+----------+
| Fruit | Price/Kg |
+========+==========+
| Apple | 25 |
+--------+----------+
| Banana | 20 |
+--------+----------+
Printing Lists as Tabular Data using Pandas library
The most common way of printing lists in tabular form is by using the Pandas library in Python. Pandas is an important Python library used for data analysis and data manipulation. It stores data in the form of data frames which can be presented in tabular form. You can install Pandas by using the following command in the CMD console.
pip install pandas
Example 1:
Data can be arranged in the form of a list where a 2D array-like structure consisting of rows and columns is formed to present data in tabular format using pd.Dataframe. Look at the example given below to get a better understanding.
Python3
import pandas as pd
lst1 = [ 'Geeks' , 'For' , 'Geeks' , 'is' ,
'the' , 'best' ]
df = pd.DataFrame(lst1)
print (df)
|
Output:
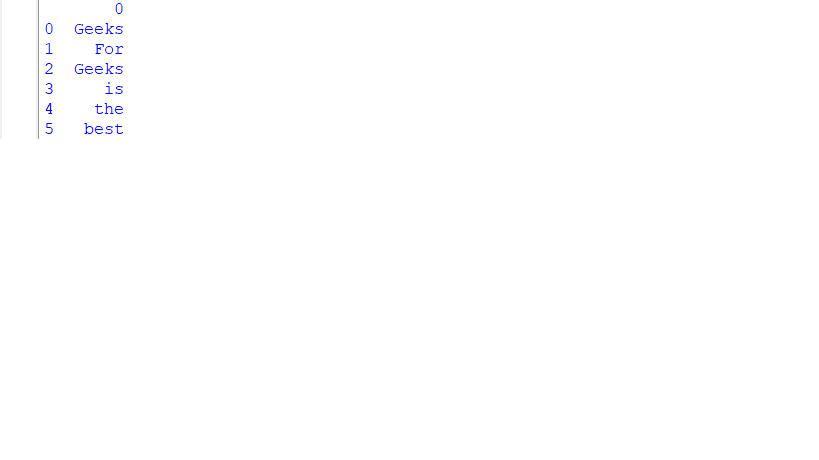
Output
Example 2:
We can see from the examples that using data frames, we can present data in columnar form using columns=[‘Tabular form’] as a parameter in pd.Dataframe.
Python3
import pandas as pd
my_list = [ 10 , 40.7 , 'apple' , 'b' ]
df = pd.DataFrame(my_list, columns = [ 'Tabular form' ])
print (df)
|
Output:
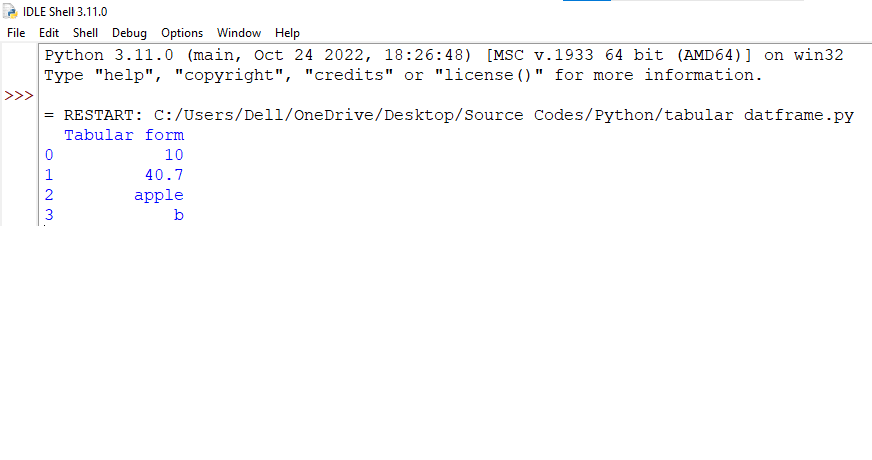
Output
Printing Lists as Tabular Data using Tabulate Module
Tabulate module is an open-source python package/module which is used to print tabular data in nicely formatted tables that make the data appear beautiful. It is user-friendly and has many formatting functions. You can install the module using the following command in the CMD console.
pip install tabulate
Example
It is quite an easy method as we simply have to input the data in the list format for the number of rows we want and provide a title/heading to the columns. Here, We are using Name and Age as headers of the table
Python3
from tabulate import tabulate
print (tabulate([[ 'Aditi' , 19 ], [ 'Anmol' , 16 ],
[ 'Bhavya' , 19 ], [ 'Ananya' , 19 ],
[ 'Rajeev' , 50 ], [ 'Parul' , 45 ]],
headers = [ 'Name' , 'Age' ]))
|
Output:
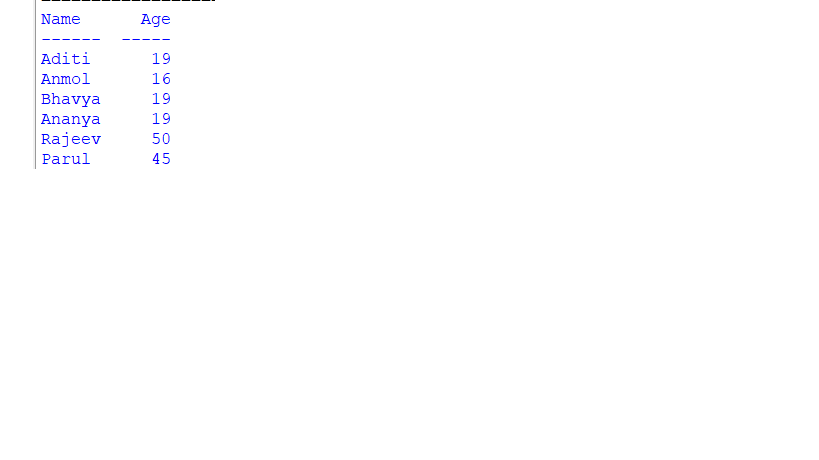
Output
Printing Lists as Tabular Data using Texttable Module
It is a Python Texttable module, which helps us to print tables on the terminal. It is one of the basic Python modules for reading and writing text tables in ASCII code. It can support both dynamic and fixed-size tables. Data is stored in a tabular format where each cell is differentiated from the other by a table border. You can install the module using the following command in the CMD console.
pip install texttable
Example
As we can see from the output shown, the items are arranged in the form of tables which makes them look structured and easier to read. Texttable enables a cell-like structure in which each data is stored and is similar to CSV files.
Python3
from texttable import Texttable
t = Texttable()
t.add_rows([[ 'Fruit' , 'Price/Kg' ], [ 'Apple' , 25 ], [ 'Banana' , 20 ]])
print (t.draw())
|
Output:
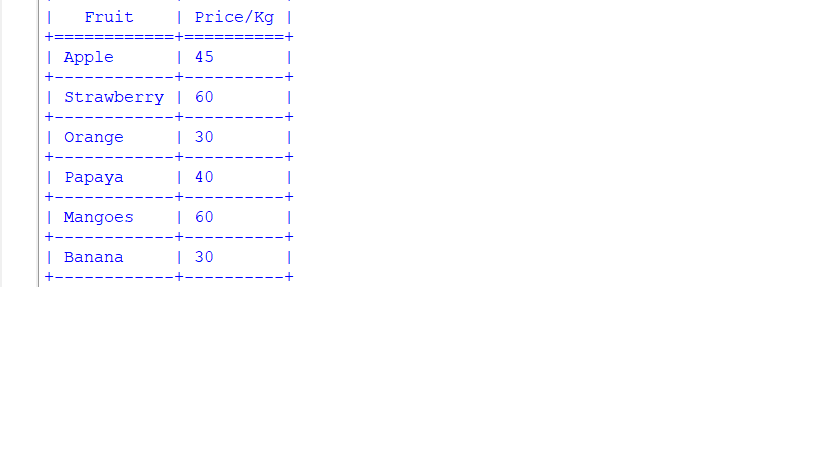
Output
Printing Lists as Tabular Data using Prettytable Module
The Prettytable is another Python module used for the tabular representation of data. They are ASCII tables and quite easy to use. The Prettytable library consists of the PrettyTable class, which is used to create relational tables. You can install the module using the following command in the CMD console.
pip install prettytable
Example
Here, the data is presented in an organized manner. Thus, you can print lists as tabular data by using PrettyTable methods, all the modules listed above can help in visualizing raw data in tabular form.
Python3
from prettytable import PrettyTable
t = PrettyTable([ 'Subject Code' , 'Subject' , 'Marks' ])
t.add_row([ 'K01' , 'Maths' , 95 ])
t.add_row([ 'K04' , 'Physics' , 89 ])
t.add_row([ 'K03' , 'Chemistry' , 92 ])
t.add_row([ 'K08' , 'English' , 91 ])
t.add_row([ 'K29' , 'Computer Science' , 99 ])
t.add_row([ 'K06' , 'Social Science' , 86 ])
print (t)
|
Output:
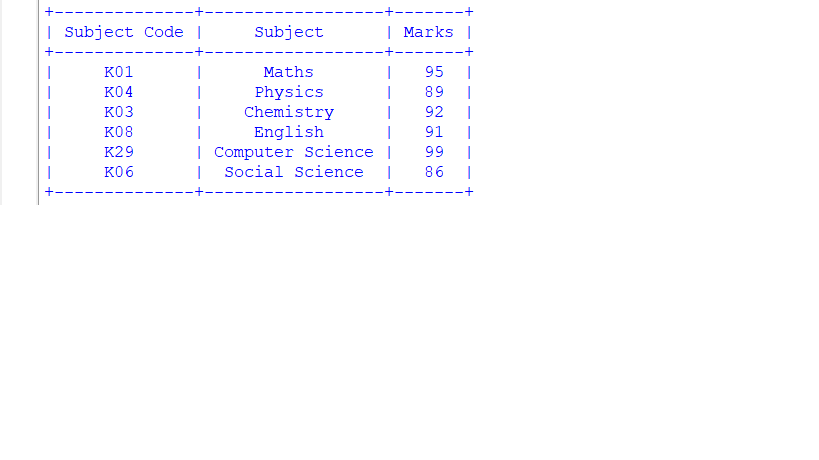
Output
Printing Lists as Tabular Data using terminaltables Module:
The terminaltables library is a Python library that allows you to create tables with ASCII and Unicode borders. It is a simple and easy-to-use library that provides various formatting options, such as text alignment, padding, and borders.
To use the terminaltables library, you need to first install it using pip install terminaltables. Then, you can import the AsciiTable class and create an instance of it by passing your data as an argument. The data should be in the form of a list of lists, where each inner list represents a row in the table.
Once you have created an instance of the AsciiTable class, you can use the table attribute to print the table. The table attribute returns the formatted table as a string, which can be printed to the terminal or saved to a file.
Here is an example of how to use the terminaltables library to print a table:
Python3
from terminaltables import AsciiTable
data = [[ 'Fruit' , 'Price/Kg' ], [ 'Apple' , 25 ], [ 'Banana' , 20 ]]
table = AsciiTable(data)
print (table.table)
|
This will output the following table:
+--------+----------+
| Fruit | Price/Kg |
+--------+----------+
| Apple | 25 |
+--------+----------+
| Banana | 20 |
+--------+----------+
The AsciiTable class has several formatting options that you can use to customize the appearance of the table. For example, you can use the title attribute to set a title for the table, the justify attribute to specify the text alignment (left, right, or center), and the inner_row_border attribute to enable or disable the inner row border.
Here is an example of how to use some of the formatting options:
Python3
from terminaltables import AsciiTable
data = [[ 'Fruit' , 'Price/Kg' ], [ 'Apple' , 25 ], [ 'Banana' , 20 ]]
table = AsciiTable(data, title = 'Fruit Prices' )
table.justify = 'center'
table.inner_row_border = True
print (table.table)
|
This will output the following table:
+------------+
| Fruit Prices |
+============+
| Fruit | Price/Kg |
+------------+----------+
| Apple | 25 |
+------------+----------+
| Banana | 20 |
+------------+----------+
Share your thoughts in the comments
Please Login to comment...