PHP Program to Calculate Root Mean Square
Last Updated :
19 Feb, 2024
Root Mean Square (RMS) is a statistical measure of the magnitude of a varying quantity. It is commonly used in physics, engineering, and statistics to represent the effective value of a set of numbers. In this article, we will discuss how to calculate the RMS of a set of numbers in PHP using different approaches.
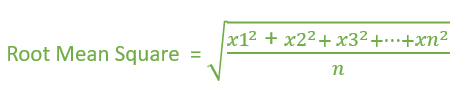
Calculate Root Mean Square using a Loop
This approach in the provided PHP program iterates through each number in the given array, squares each number, and then calculates the mean of the squares. Finally, it computes the square root of the mean of the squares to obtain the Root Mean Square (RMS) value.
Example: Implementation to calculate root mean square.
PHP
<?php
function calculateRMS( $numbers ) {
$sumOfSquares = 0;
$count = count ( $numbers );
foreach ( $numbers as $number ) {
$sumOfSquares += pow( $number , 2);
}
$meanOfSquares = $sumOfSquares / $count ;
$rms = sqrt( $meanOfSquares );
return $rms ;
}
$numbers = [2, 3, 4, 5];
$rms = calculateRMS( $numbers );
echo "Root Mean Square: " . $rms ;
?>
|
Output
Root Mean Square: 3.6742346141748
Explanation:
- The
calculateRMS
function takes an array of numbers as input.
- It calculates the sum of the squares of the numbers using a loop.
- It then calculates the mean of the squares by dividing the sum of squares by the count of numbers.
- Finally, it returns the square root of the mean of squares, which is the RMS.
Calculate Root Mean Square using Array Functions
PHP provides built-in array functions that can be used to calculate RMS in a more concise way.
Example: Implementation to calculate root mean square.
PHP
<?php
function calculateRMS( $numbers ) {
$square = function ( $n ) {
return pow( $n , 2);
};
$squaredNumbers = array_map ( $square , $numbers );
$meanOfSquares = array_sum ( $squaredNumbers ) / count ( $numbers );
$rms = sqrt( $meanOfSquares );
return $rms ;
}
$numbers = [2, 3, 4, 5];
$rms = calculateRMS( $numbers );
echo "Root Mean Square: " . $rms ;
?>
|
Output
Root Mean Square: 3.6742346141748
Explanation:
- The
calculateRMS
function uses the array_map( )
function to apply a function that squares each number in the array.
- It then uses the
array_sum(
)
function to calculate the sum of the squared numbers.
- The mean of squares is calculated by dividing the sum of squares by the count of numbers, and the RMS is the square root of the mean of squares.
Share your thoughts in the comments
Please Login to comment...