PHP | ImagickDraw color() Function
Last Updated :
07 Mar, 2024
The ImagickDraw::color() function is an inbuilt function in PHP which is used to draw color on the image using the current fill color, starting at the specified position, and using specified paint method.
Syntax:
bool ImagickDraw::color( float $x, float $y, int $paintMethod )
Parameters: This function accepts three parameters as mentioned above and described below:
- $x: It specifies the x-coordinate of the paint.
- $y: It specifies the y-coordinate of the paint.
- $paintMethod: It specifies an integer corresponding to one of PAINT constants.
List of PAINT constants are given below:
- imagick::PAINT_POINT (1)
- imagick::PAINT_REPLACE (2)
- imagick::PAINT_FLOODFILL (3)
- imagick::PAINT_FILLTOBORDER (4)
- imagick::PAINT_RESET (5)
Return Value: This function returns TRUE on success.
Exceptions: This function throws ImagickException on error.
Below given programs illustrate the ImagickDraw::color() function in PHP:
Program 1:
<?php
$imagick = new Imagick();
$imagick ->newImage(800, 250, 'white' );
$draw = new ImagickDraw();
$x = 0;
while ( $x < 900) {
$draw ->color( $x , 0, 1);
$draw ->color( $x , 30, 1);
$draw ->color( $x , 60, 1);
$draw ->color( $x , 90, 1);
$draw ->color( $x , 120, 1);
$draw ->color( $x , 150, 1);
$draw ->color( $x , 180, 1);
$draw ->color( $x , 210, 1);
$draw ->color( $x , 240, 1);
$x ++;
}
$imagick ->drawImage( $draw );
$imagick ->setImageFormat( "png" );
header( "Content-Type: image/png" );
echo $imagick ->getImageBlob();
?>
|
Output:
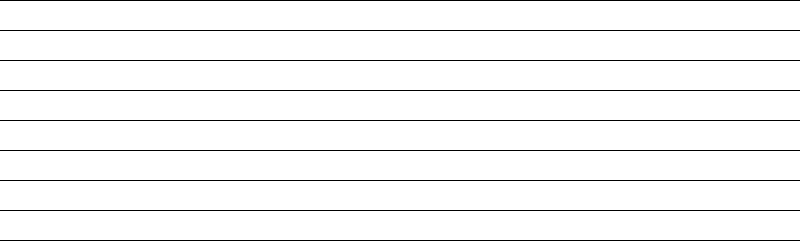
Program 2:
<?php
$imagick = new Imagick();
$imagick ->newImage(800, 250, 'white' );
$draw = new ImagickDraw();
$draw ->setFillColor( 'green' );
$draw ->color(1, 1, Imagick::PAINT_FLOODFILL);
$imagick ->drawImage( $draw );
$imagick ->setImageFormat( "png" );
header( "Content-Type: image/png" );
echo $imagick ->getImageBlob();
?>
|
Output:
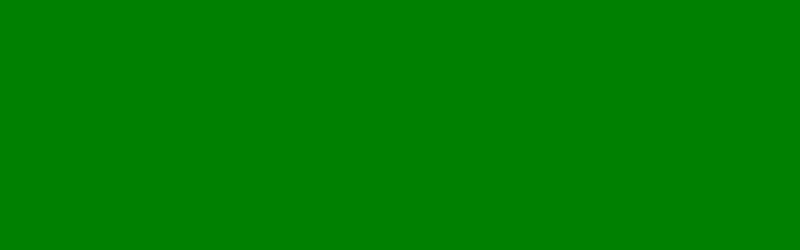
Share your thoughts in the comments
Please Login to comment...