Perl | log() Function
Last Updated :
25 Jun, 2019
log() function in Perl returns the natural logarithm of value passed to it. Returns $_ if called without passing a value. log() function can be used to find the log of any base by using the formula:
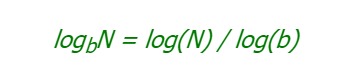
Syntax: log(value)
Parameter:
value: Number of which log is to be calculated
Returns:
Floating point number in scalar context
Example 1:
print "log10(2): " , log10(2), "\n" ;
print "log10(7): " , log10(7), "\n" ;
print "log10(9): " , log10(9), "\n" ;
sub log10
{
my $n = shift ;
return log ( $n ) / log (10);
}
|
Output:
log10(2): 0.301029995663981
log10(7): 0.845098040014257
log10(9): 0.954242509439325
Example 2:
print "log3(2): " , log3(2), "\n" ;
print "log5(7): " , log5(7), "\n" ;
print "log2(9): " , log2(9), "\n" ;
sub log3
{
my $n = shift ;
return log ( $n ) / log (3);
}
sub log5
{
my $n = shift ;
return log ( $n ) / log (5);
}
sub log2
{
my $n = shift ;
return log ( $n ) / log (2);
}
|
Output:
log3(2): 0.630929753571457
log5(7): 1.20906195512217
log2(9): 3.16992500144231
Share your thoughts in the comments
Please Login to comment...