Optparse module in Python
Last Updated :
30 Nov, 2022
Optparse module makes easy to write command-line tools. It allows argument parsing in the python program.
- optparse make it easy to handle the command-line argument.
- It comes default with python.
- It allows dynamic data input to change the output
Code: Creating an OptionParser object.
Python3
import optparse
parser = optparse.OptionParser()
|
Defining options:
It should be added one at a time using the add_option(). Each Option instance represents a set of synonymous command-line option string.
Way to create an Option instance are:
OptionParser.add_option(option)
OptionParser.add_option(*opt_str, attr=value, ...)
To define an option with only a short option string:
parser.add_option("-f", attr=value, ....)
And to define an option with only a long option string:
parser.add_option("--foo", attr=value, ....)
Standard Option Actions:
- “store”: store this option’s argument (default).
- “store_const”: store a constant value.
- “store_true”: store True.
- “store_false”: store False.
- “append”: append this option’s argument to a list.
- “append_const”: append a constant value to a list.
Standard Option Attributes:
- Option.action: (default: “store”)
- Option.type: (default: “string”)
- Option.dest: (default: derived from option strings)
- Option.default: The value to use for this option’s destination if the option is not seen on the command line.
Here’s an example of using optparse module in a simple script:
Python3
from optparse import OptionParser
parser = OptionParser()
parser.add_option( "-f" , "--file" ,
dest = "filename" ,
help = "write report to FILE" ,
metavar = "FILE" )
parser.add_option( "-q" , "--quiet" ,
action = "store_false" ,
dest = "verbose" , default = True ,
help = "don't print status messages to stdout" )
(options, args) = parser.parse_args()
|
With these few lines of code, users of your script can now do the “usual thing” on the command-line, for example:
<yourscript> --file=outfile -q
Lets, understand with an example:
Code: Writing python script for print table of n.
Python3
import optparse
def table(n, dest_cheak):
for i in range ( 1 , 11 ):
tab = i * n
if dest_cheak:
print (tab)
return tab
def Main():
parser = optparse.OptionParser()
parser.add_option( '-n' , dest = 'num' ,
type = 'int' ,
help = 'specify the n' 'th table number to output' )
parser.add_option( '-o' , dest = 'out' ,
type = 'string' ,
help = 'specify an output file (Optional)' )
parser.add_option( "-a" , "--all" ,
action = "store_true" ,
dest = "print" ,
default = False ,
help = "print all numbers up to N" )
(options, args) = parser.parse_args()
if (options.num = = None ):
print (parser.usage)
exit( 0 )
else :
number = options.num
result = table(number, options. print )
print ( "The " + str (number) + "th table is " + str (result))
if (options.out ! = None ):
f = open (options.out, "a" )
f.write( str (result) + '\n' )
if __name__ = = '__main__' :
Main()
|
Output:
python file_name.py -n 4
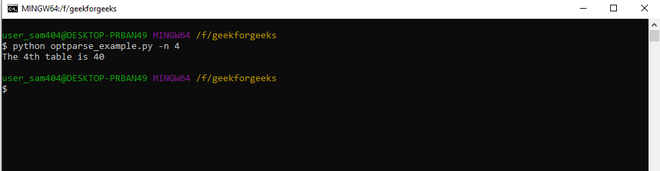
python file_name.py -n 4 -o
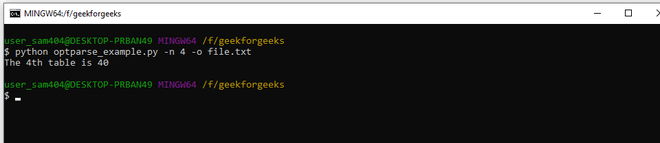
file.txt created
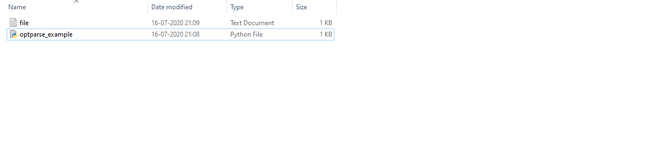
python file_name.py -n 4 -a
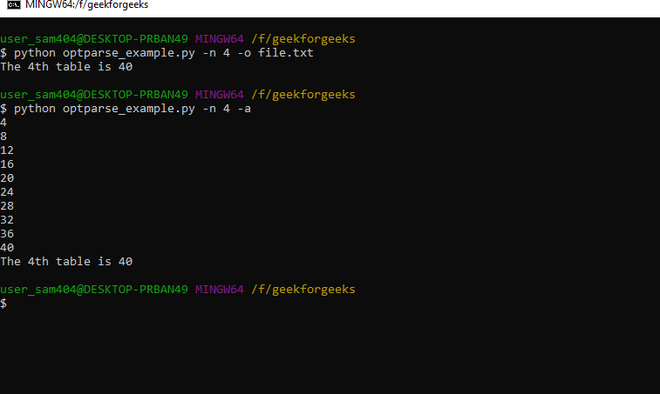
For knowing more about this module click here.
Share your thoughts in the comments
Please Login to comment...