Obtain List of Directories in R
Last Updated :
06 Jun, 2021
A directory or folder in the R programming language may contain other directories. It is possible to access the list of all the directories using base methods in R and return them as a list of folders.
The list.dirs() method in R language is used to retrieve a list of directories present within the path specified. The output returned is in the form of a character vector containing the names of the files contained in the specified directory path, or returns null if no directories were returned. If the specified path does not exist or is not a directory or is unreadable it is skipped from evaluation.
Syntax:
list.dirs(path = “.”, full.names = TRUE, recursive = TRUE)
Parameter:
path – The path of the main directory
full.names (Default : TRUE) – If set to FALSE, only the names of the subdirectories are displayed. Else, the entire paths are displayed.
Returns: List of files in lexicographic order
Directory in use:
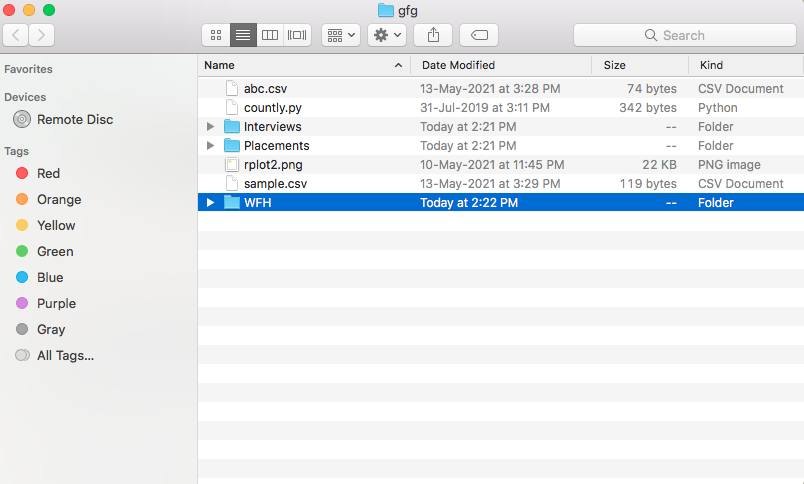
Input main directory
Getting the entire paths of directories in R
The full path returned when the attribute full.names = TRUE. If we set, recursive = TRUE, the directories are returned in hierarchical order, beginning with the main directory path and then followed by the sub-directories. However, if we specify recursive = FALSE, only the child directories are returned.
Example:
R
main_dir <- "/Users/mallikagupta/Desktop/gfg"
print ( "list of directories including the main directory" )
dir_list <- list.dirs (main_dir,recursive = TRUE )
print (dir_list)
print ( "list of directories excluding the main directory" )
dir_list <- list.dirs (main_dir,recursive = FALSE )
print (dir_list)
|
Output
“list of directories including the main directory”
[1] “/Users/mallikagupta/Desktop/gfg” “/Users/mallikagupta/Desktop/gfg/Interviews”
[3] “/Users/mallikagupta/Desktop/gfg/Placements” “/Users/mallikagupta/Desktop/gfg/WFH”
“list of directories excluding the main directory”
[1] “/Users/mallikagupta/Desktop/gfg/Interviews” “/Users/mallikagupta/Desktop/gfg/Placements”
[3] “/Users/mallikagupta/Desktop/gfg/WFH”
Another way, to exclude the main directory is to specify the [-1] index appended to the list.dirs() method which returns only subdirectories.
Example:
R
main_dir <- "/Users/mallikagupta/Desktop/gfg"
print ( "list of directories" )
dir_list <- list.dirs (main_dir)[- 1]
print (dir_list)
|
Output
“list of directories”
[1] “/Users/mallikagupta/Desktop/gfg/Interviews” “/Users/mallikagupta/Desktop/gfg/Placements”
[3] “/Users/mallikagupta/Desktop/gfg/WFH”
Getting folder names in R
It is possible to retrieve only the folder or directory names within the main directory. In this case, we set the parameter full.names = FALSE in the method call. The names are alphabetically sorted.
Example:
R
main_dir <- "/Users/mallikagupta/Desktop/gfg"
print ( "list of directory names" )
dir_list <- list.dirs (main_dir,full.names = FALSE ,
recursive = FALSE )
print (dir_list)
|
Output
“list of directory names”
[1] “Interviews” “Placements” “WFH”
gsub() function is used to replace all the matches of a pattern from a string. The pattern we specify is equivalent to a regex (“\\./”) which is used to remove all the previous segments from the complete pathname and return only the last part of the path, that is the folder name.
Syntax:
gsub(pattern, replacement, string)
Parameter:
pattern – The pattern to locate in the string
replacement – The string to replace the matches with.
Example:
R
main_dir <- "/Users/mallikagupta/Desktop/gfg"
setwd (main_dir)
print ( "list of directories" )
dir_list <- list.dirs ()[- 1]
folder_list <- gsub ( "\\./" , "" , dir_list)
print (folder_list)
|
Output
“list of directories”
[1] “Interviews” “Placements” “WFH”
Share your thoughts in the comments
Please Login to comment...