Numpy data Types
Last Updated :
04 Sep, 2023
NumPy is a powerful Python library that can manage different types of data. Here we will explore the Datatypes in NumPy and How we can check and create datatypes of the NumPy array.
DataTypes in NumPy
A data type in NumPy is used to specify the type of data stored in a variable. Here is the list of characters to represent data types available in NumPy.
b
|
Boolean
|
f
|
Float
|
m
|
Time Delta
|
O
|
Object
|
U
|
Unicode String
|
i
|
Integer
|
u
|
Unsigned Integer
|
c
|
Complex Float
|
M
|
DateTime
|
S
|
String
|
V
|
A fixed chunk of memory for other types (void)
|
The list of various types of data types provided by NumPy are given below:
bool_
|
Boolean
|
int_
|
Default integer type (int64 or int32)
|
intc
|
Identical to the integer in C (int32 or int64)
|
intp
|
Integer value used for indexing
|
int8
|
8-bit integer value (-128 to 127)
|
int16
|
16-bit integer value (-32768 to 32767)
|
int32
|
32-bit integer value (-2147483648 to 2147483647)
|
int64
|
64-bit integer value (-9223372036854775808 to 9223372036854775807)
|
uint8
|
Unsigned 8-bit integer value (0 to 255)
|
uint16
|
Unsigned 16-bit integer value (0 to 65535)
|
uint32
|
Unsigned 32-bit integer value (0 to 4294967295)
|
uint64
|
Unsigned 64-bit integer value (0 to 18446744073709551615)
|
float_
|
Float values
|
float16
|
Half precision float values
|
float32
|
Single-precision float values
|
float64
|
Double-precision float values
|
complex_
|
Complex values
|
complex64
|
Represent two 32-bit float complex values (real and imaginary)
|
complex128
|
Represent two 64-bit float complex values (real and imaginary)
|
Checking the Data Type of NumPy Array
We can check the datatype of Numpy array by using dtype. Then it returns the data type all the elements in the array. In the given example below we import NumPy library and craete an array using “array()” method with integer value. Then we store the data type of the array in a variable named “data_type” using the ‘dtype’ attribute, and after then we can finally, we print the data type.
Python3
import numpy as np
arr = np.array([ 1 , 2 , 3 , 4 , 5 ])
data_type = arr.dtype
print ( "Data type:" , data_type)
|
Output:
Data type: int64
Create Arrays With a Defined Data Type
We can create an array with a defined data type by specifying “dtype” attribute in numpy.array() method while initializing an array. In the below code we have created various types of defined arrays such as ‘float64’, ‘int32’, ‘complex128’, and ‘bool’.
Python3
import numpy as np
arr1 = np.array([ 1 , 2 , 3 , 4 ], dtype = np.float64)
arr2 = np.zeros(( 3 , 3 ), dtype = np.int32)
arr3 = np.ones(( 2 , 2 ), dtype = np.complex128)
arr4 = np.empty(( 4 ,), dtype = np.bool_)
print ( "arr1:\n" , arr1)
print ( "Data type of arr1:" , arr1.dtype)
print ( "\narr2:\n" , arr2)
print ( "Data type of arr2:" , arr2.dtype)
print ( "\narr3:\n" , arr3)
print ( "Data type of arr3:" , arr3.dtype)
print ( "\narr4:\n" , arr4)
print ( "Data type of arr4:" , arr4.dtype)
|
Output:
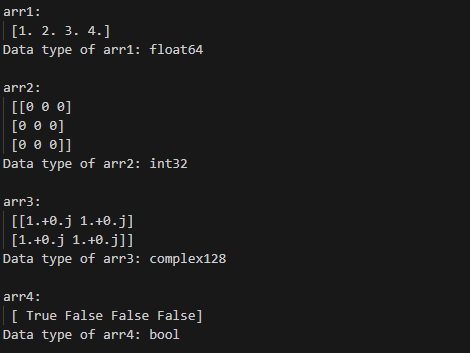
Convert Data Type of NumPy Arrays
We can convert data type of an arrays from one type to another using astype() function. In the below code we have initialize an array with float type values. After that we have convert that float64 type array to int32 type using astype() function. Finally, print the array and their types of original array and new array.
Python3
import numpy as np
arr_original = np.array([ 1.2 , 2.5 , 3.7 ])
arr_new = arr_original.astype(np.int32)
print ( "Original array:" , arr_original)
print ( "Data type of original array:" , arr_original.dtype)
print ( "\nNew array:" , arr_new)
print ( "Data type of new array:" , arr_new.dtype)
|
Output:

Share your thoughts in the comments
Please Login to comment...