Movie Recommendation System with Node and Express.js
Last Updated :
07 Mar, 2024
Building a movie recommendation system with Node and Express will help you create personalized suggestions and recommendations according to the genre you selected. To generate the recommendation OpenAI API is used. In this article, you will see the step-wise guide to build a Movie recommendation system.
Output Preview: Let us have a look at how the final output will look like.
.gif)
Prerequisites :
Approach to create Movie Recommendation System:
- Input processing: Analyze user preferences and input using the OpenAI API.
- Personalized Recommendations: Utilize the OpenAI API to generate movie recommendations based on user preferences.
- Responsive Design: Ensure the application is responsive across various devices.
- User interface: Provide a user-friendly interface to interact with the system.
- Error Handling: Implement robust error handling to enhance user experience
Steps to create the project:
Step 1: Set Up Your Development Environment:
- Install Node.js: If you haven’t already, download and install Node.js from the official website (https://nodejs.org/). Follow the installation instructions for your operating system.
- Verify Installation: After installation, open a terminal (or command prompt) and run node -v and npm -v to ensure Node.js and npm (Node Package Manager) are installed correctly.
Step 2: Set Up Project Structure:
Create a new directory for your project and navigate into it:
mkdir movie-recommendation
cd movie-recommendation
Step 3: Install Dependencies:
You’ll need to install Express.js and Axios for making HTTP requests:
npm init -y
npm install express axios dotenv
Folder Structure:
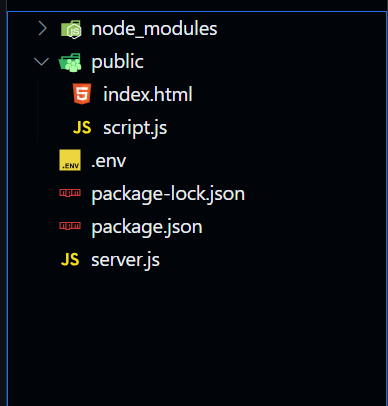
The updated Dependencies in package.json file will look like:
"dependencies": {
"axios": "^1.6.7",
"dotenv": "^16.4.5",
"express": "^4.18.3"
}
Example: Create the files as suggested in folder structure and add the following code.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" />
< title >Movie Recommendation</ title >
</ head >
< body >
< div class = "container" >
< br >
< h1 class = "text-center" >Movie Recommendation System</ h1 >
< div class = "form-group" >
< label for = "userInput" >Enter Your Preferences:</ label >
< textarea class = "form-control" id = "userInput" rows = "3" ></ textarea >
</ div >
< button type = "button" class = "btn btn-primary" id = "recommendBtn" >
Get Movie Recommendation
</ button >
< div id = "recommendation" class = "mt-3" ></ div >
< div id = "loader" class = "mt-3 text-center" style = "display: none;" >
< div class = "spinner-border text-primary" role = "status" >
< span class = "sr-only" >Loading...</ span >
</ div >
</ div >
</ div >
< script src = "script.js" defer></ script >
</ body >
</ html >
|
Javascript
document.addEventListener( "DOMContentLoaded" , () => {
const recommendBtn = document.getElementById( "recommendBtn" );
const userInput = document.getElementById( "userInput" );
const recommendationDiv = document.getElementById( "recommendation" );
const loaderDiv = document.getElementById( "loader" );
recommendBtn.addEventListener( "click" , async () => {
const inputText = userInput.value.trim();
if (inputText === "" ) {
alert( "Please enter your preferences." );
return ;
}
loaderDiv.style.display = "block" ;
try {
const response = await axios.get
(`/recommend?input=${inputText}`);
const recommendedMovie = marked.parse(response.data);
if (recommendedMovie) {
recommendationDiv.innerHTML = `
<h3>Recommended Movie:</h3>
<p>${recommendedMovie}</p>
`;
} else {
recommendationDiv.innerHTML = `
<p>No Movie recommendation available.</p>
`;
}
} catch (error) {
console.error(error);
recommendationDiv.innerHTML = `
<p>An error occurred while fetching Movie recommendation.</p>
`;
} finally {
loaderDiv.style.display = "none" ;
}
});
});
|
Javascript
const express = require( "express" );
const axios = require( "axios" );
const dotenv = require( "dotenv" );
const { OpenAI } = require( "openai" );
dotenv.config();
const app = express();
const PORT = process.env.PORT || 3000;
const OPENAI_API_KEY = process.env.OPENAI_API_KEY;
const openai = new OpenAI({ apiKey: OPENAI_API_KEY });
app.use(express.static( "public" ));
app.get( "/recommend" , async (req, res) => {
try {
const userInput = req.query.input;
const completion = await openai.chat.completions.create({
messages: [
{ role: "system" , content: "You are helpful in recommending Movies." },
{ role: "user" , content: userInput + "\n list out all Movies" },
],
model: "gpt-3.5-turbo" ,
});
const recommendedMovie = completion.choices[0].message.content;
res.send(recommendedMovie);
} catch (error) {
console.error(error);
res.status(500).send( "An error occurred while processing your request." );
}
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
|
.env file: This file will contain your environment variables, including your OpenAI API key.
PORT=3000
OPENAI_API_KEY=your_openai_api_key_here
Run the following command to start the server:
node server.js
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...