Maximum number of edges in Bipartite graph
Last Updated :
31 May, 2022
Given an integer N which represents the number of Vertices. The Task is to find the maximum number of edges possible in a Bipartite graph of N vertices.
Bipartite Graph:
- A Bipartite graph is one which is having 2 sets of vertices.
- The set are such that the vertices in the same set will never share an edge between them.
Examples:
Input: N = 10
Output: 25
Both the sets will contain 5 vertices and every vertex of first set
will have an edge to every other vertex of the second set
i.e. total edges = 5 * 5 = 25
Input: N = 9
Output: 20
Approach: The number of edges will be maximum when every vertex of a given set has an edge to every other vertex of the other set i.e. edges = m * n where m and n are the number of edges in both the sets. in order to maximize the number of edges, m must be equal to or as close to n as possible. Hence, the maximum number of edges can be calculated with the formula,
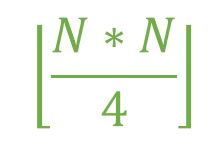
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int maxEdges( int N)
{
int edges = 0;
edges = floor ((N * N) / 4);
return edges;
}
int main()
{
int N = 5;
cout << maxEdges(N);
return 0;
}
|
Java
class GFG {
public static double maxEdges( double N)
{
double edges = 0 ;
edges = Math.floor((N * N) / 4 );
return edges;
}
public static void main(String[] args)
{
double N = 5 ;
System.out.println(maxEdges(N));
}
}
|
Python3
def maxEdges(N) :
edges = 0 ;
edges = (N * N) / / 4 ;
return edges;
if __name__ = = "__main__" :
N = 5 ;
print (maxEdges(N));
|
C#
using System;
class GFG {
static double maxEdges( double N)
{
double edges = 0;
edges = Math.Floor((N * N) / 4);
return edges;
}
static public void Main()
{
double N = 5;
Console.WriteLine(maxEdges(N));
}
}
|
PHP
<?php
function maxEdges( $N )
{
$edges = 0;
$edges = floor (( $N * $N ) / 4);
return $edges ;
}
$N = 5;
echo maxEdges( $N );
?>
|
Javascript
<script>
function maxEdges(N)
{
var edges = 0;
edges = Math.floor((N * N) / 4);
return edges;
}
var N = 5;
document.write( maxEdges(N));
</script>
|
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...