Maximum area that can be saved in the given Matrix
Last Updated :
09 Dec, 2023
Consider a matrix of N rows and M columns. Initially, there is a cell at co-ordinate (X, Y), which is blue. Then the task is to place the Yellow color in an optimal cell such that the area with yellow color after ending below simulation is the maximum possible. The simulation takes place as follows (after putting a yellow color in a cell):
- The cells, that are adjacent to blue cells and not colored yellow becomes blue.
- The cells, that are adjacent to yellow cells and not colored blue becomes yellow.
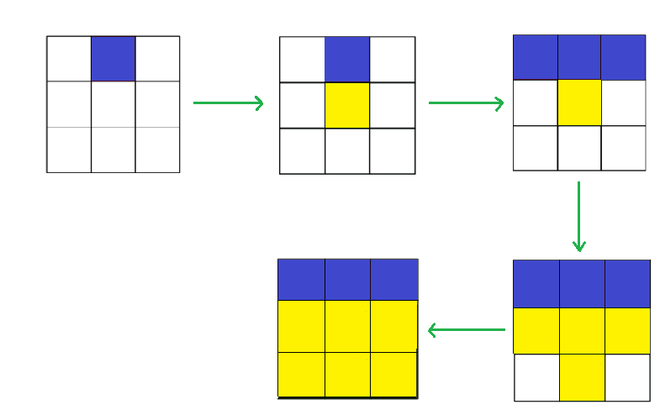
Explanation of simulation
Examples:
Input: N = 3, M = 2, X = 1, Y = 2
Output: 4
Explanation: The blue colored cell is placed at (1, 2) initially. It will be optimal to place yellow cell at (2, 2). By doing so we will get yellow colored area 4 sq. units, which is maximum possible.
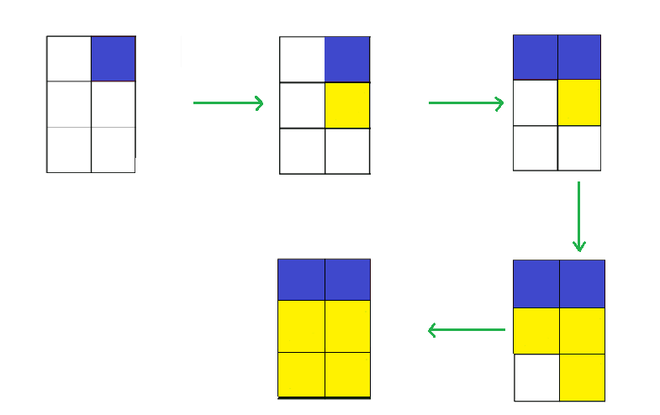
Explanation of test case 1
Input: N = 3, M = 4, X = 2, Y = 2
Output: 6
Explanation: It can be verified that placing yellow color at optimal cell will give us the maximum yellow colored area as 6 sq. units.
Approach: Implement the idea below to solve the problem
This problem is an observation based. Let us the observations:
- The optimal way to choose the starting cell of yellow color is the adjacent cell of the first blue cell. We can place the first yellow color in a cell just above, just below, just left or just right of the initial blue cell.
- Out of the above four choices, wherever you place the first yellow color cell, a part of the matrix will be blue.
- Say, If you place the yellow cell just below the blue cell, all the rows above the blue will be colored with blue with the simulation for sure.
- If we place the yellow cell above the blue cell, the lower part will get blue with the simulation.
- Similarly for right and left positions.
Steps were taken to solve the problem:
- Create a variable let say Total to store the total possible area of matrix and initialize it with N*M
- Create six variables let say, A, B, C, D, C1, C2 and C3
- Set all the above variables as follows:
- A = X*M
- B = (N-X+1)*M
- C = Y*N
- D = (M-Y+1)*N
- C1 = min (A, B)
- C2 = min (C, D)
- C3 = min (C1, C2)
- Output (Total – C3)
Code to implement the approach:
C++
#include <iostream>
void Max_Area( int N, int M, int X, int Y);
int main()
{
int N = 3, M = 2, X = 1, Y = 1;
Max_Area(N, M, X, Y);
return 0;
}
void Max_Area( int N, int M, int X, int Y)
{
int total = N * M;
int A, B, C, D, C1, C2, C3;
A = X * M;
B = (N - X + 1) * M;
C = Y * N;
D = (M - Y + 1) * N;
C1 = std::min(A, B);
C2 = std::min(C, D);
C3 = std::min(C1, C2);
std::cout << total - C3 << std::endl;
}
|
Java
public class Main {
public static void main(String[] args)
{
int N = 3 , M = 2 , X = 1 , Y = 1 ;
Max_Area(N, M, X, Y);
}
public static void Max_Area( int N, int M, int X, int Y)
{
int total = N * M;
int A, B, C, D, C1, C2, C3;
A = X * M;
B = (N - X + 1 ) * M;
C = Y * N;
D = (M - Y + 1 ) * N;
C1 = Math.min(A, B);
C2 = Math.min(C, D);
C3 = Math.min(C1, C2);
System.out.println(total - C3);
}
}
|
Python
def Max_Area(N, M, X, Y):
total = N * M
A = X * M
B = (N - X + 1 ) * M
C = Y * N
D = (M - Y + 1 ) * N
C1 = min (A, B)
C2 = min (C, D)
C3 = min (C1, C2)
print (total - C3)
N, M, X, Y = 3 , 2 , 1 , 1
Max_Area(N, M, X, Y)
|
C#
using System;
public class MainClass
{
public static void Max_Area( int N, int M, int X, int Y)
{
int total = N * M;
int A = X * M;
int B = (N - X + 1) * M;
int C = Y * N;
int D = (M - Y + 1) * N;
int C1 = Math.Min(A, B);
int C2 = Math.Min(C, D);
int C3 = Math.Min(C1, C2);
Console.WriteLine(total - C3);
}
public static void Main( string [] args)
{
int N = 3, M = 2, X = 1, Y = 1;
Max_Area(N, M, X, Y);
}
}
|
Javascript
function Max_Area(N, M, X, Y) {
const total = N * M;
const A = X * M;
const B = (N - X + 1) * M;
const C = Y * N;
const D = (M - Y + 1) * N;
const C1 = Math.min(A, B);
const C2 = Math.min(C, D);
const C3 = Math.min(C1, C2);
console.log(total - C3);
}
const N = 3, M = 2, X = 1, Y = 1;
Max_Area(N, M, X, Y);
|
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...