Loop Inside React JSX
Last Updated :
08 May, 2024
When working with React, you often need to render lists of items dynamically. JavaScript’s map function provides a convenient way to loop through arrays and generate JSX elements for each item. In this article, we’ll explore how to loop inside JSX in React using various techniques and best practices.
Prerequisites
Steps to Create React Application
Step 1: Create a React application using the following command:
npx create-react-app myapp
Step 2: After creating your project folder i.e. folder name, move to it using the following command:
cd myapp
Step 3: After setting up the react environment on your system, we can start by creating an App.js file.
Project Structure
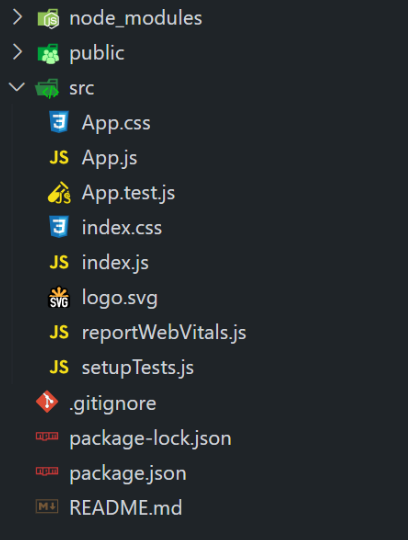
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
The approaches to loop inside the React JSX are given below:
Using Array.map( ) Method
In this approach, we are using Array.map() inside JSX to dynamically render a list of numbers from the state array numbers. Each number is displayed as an <li> element with inline styling for color, margin, and cursor. The approach1Fn function adds a random number to the list when the “Click Me” button is clicked, demonstrates dynamic rendering and user interaction within React JSX.
Syntax:
array.map((currentValue, index, array) => {
// code
}, thisArg);
Example: The below example uses Array.map() to Loop inside React JSX.
JavaScript
// App.js
import React, { useState } from 'react';
const App = () => {
const [numbers, setNumbers] =
useState([1, 2, 3, 4, 5]);
const approach1Fn = () => {
const randomNum =
Math.floor(Math.random() * 10) + 1;
setNumbers([...numbers, randomNum]);
};
return (
<div>
<h1 style={{ color: 'green' }}>
GeeksforGeeks
</h1>
<h3>Looping with Array.map()</h3>
<ul>
{numbers.map((number, index) => (
<li key={index}
style={{
marginBottom: '10px',
color: 'blue',
cursor: 'pointer'
}}>
Number {number}
</li>
))}
</ul>
<button onClick={approach1Fn}>
Click Me
</button>
</div>
);
};
export default App;
Output:
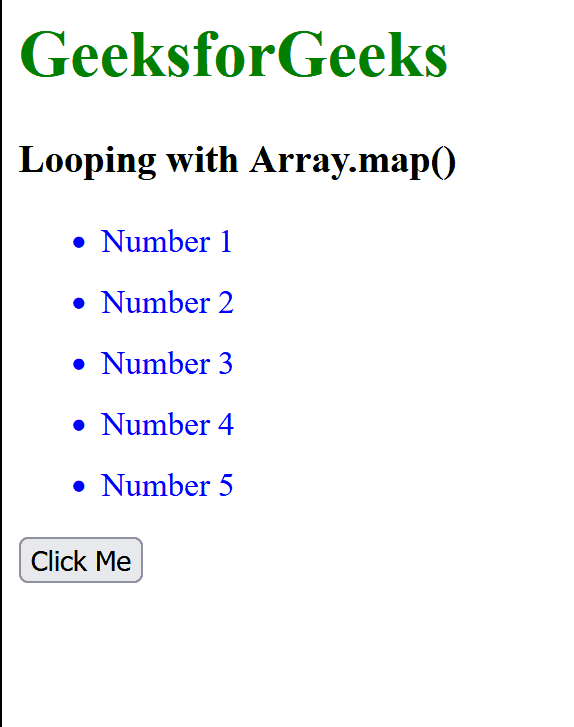
Using Traditional Loop
In this approach, a traditional for loop is used within JSX to dynamically render colored div elements based on the colors array. The loop iterates through the colors array, generating div elements with inline styling for each color. Users can add new colors to the list using the approach2Fn function, demonstrating dynamic rendering and user interaction within a React component.
Syntax:
for (initialization; condition; increment/decrement) {
// code
}
Example: The below example uses traditional Loop to Loop inside React JSX.
JavaScript
import React, { useState } from 'react';
const App = () => {
const [colors, setColors] =
useState(['red', 'green', 'blue']);
const approach2Fn = () => {
const newColor = prompt('Enter a color:');
if (newColor) {
setColors([...colors, newColor]);
}
};
const colorItems = [];
for (let i = 0; i < colors.length; i++) {
colorItems.push(
<div key={i}
style={{
backgroundColor: colors[i],
padding: '10px', margin: '5px',
borderRadius: '2px', width: '100px'
}}>
{colors[i]}
</div>
);
}
return (
<div>
<h1 style={{ color: 'green' }}>
GeeksforGeeks
</h1>
<h3>Using Traditional Loop</h3>
<div>{colorItems}</div>
<button onClick={approach2Fn}>
Add Color
</button>
</div>
);
};
export default App;
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...