Laravel | Routing Basics
Last Updated :
28 Apr, 2023
Once you have installed Laravel and your basic Web App is up and running. Let’s just look more deeply into the framework and see how we can work with routes.
Routes: Routes are actually the web URLs that you can visit in your web application. For example /home, /profile, /dashboard etc are all different routes that one can create in a Laravel Application. Keep in mind that, routes are case sensitive thus /profile is different than /Profile.
Creating Routes: In Laravel, all of our routes are going to be written in routes/web.php file as this directory is made standard for all our web-related routes. Open this file and let’s create our first route with Laravel, write to the end of this file.
Syntax: To write route is as given below:
// Syntax of a route
Route::request_type('/url', 'function()');
Program:
php
Route::get( '/sayhello' , function () {
return 'Hey ! Hello' ;
})
|
Output:
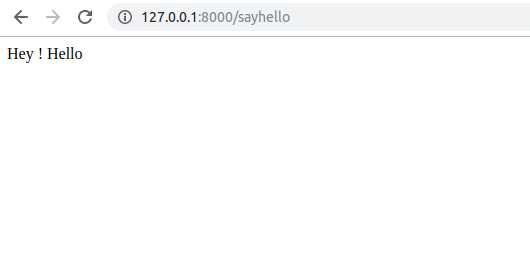
Breaking down the code given above Route::get means this is a route that will expect a GET request. The /sayhello is the name of the route and you can create a route with any name. Further, we have to specify what to do when we visit that route in the browser and we do so in the form of a callback function that returns a string saying Hey ! Hello.
Returning Web-page: Instead of just returning strings, we are going to return webpages when someone visits a route. Let’s see how we can do that. First of all create a file called index.blade.php in resources/views. In Laravel we have a built-in templating engine called Blade thus we write all of our webpages in *.blade.php not *.html.
Program 1:
php
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Hello! World.</h1>
</body>
</html>
|
Program 2: Add the following code to your web.php now.
php
Route::get( '/viewhello' , function () {
return view( 'index' );
});
|
Output:
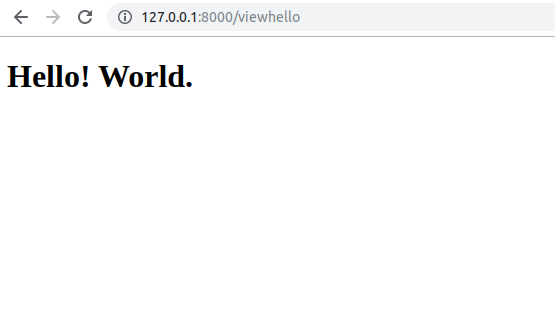
In the code given above, we have used /viewhello as the name of the route and in the callback function we have used a view() method which is an inbuilt method provided by Laravel to serve webpage and it automatically picks the file matching from resources/views folder. For example, passing ‘index’ will serve index.blade.php.
Routes with controllers: Laravel provides us with much more power than just writing a direct callback function. We can actually make our routes point to a function inside the controllers. To do so let’s manually create our controller first and call it mycontroller. Just go to app/Http/Controllers and create a file called mycontroller.php. Write the following code in this file:
Program 1: The code written below is a basic controller code where we are just using the Controllers namespace to add the capability to use it, it’s just like importing the libraries. Let’s add the function now:
php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class mycontroller extends Controller {
}
|
Program 2: Here we have created a function called index() and inside it we are using view method to serve index2.blade.php. Now let’s make such file in resources/views and add the following code to it:
php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class mycontroller extends Controller {
public function index() {
return view( 'index2' );
}
}
|
Program 3: We have written the frontend file, and written controller, and now the last thing is registering the route.
php
<!DOCTYPE html>
<html lang="en">
<body>
<h1>This is index 2.</h1>
</body>
</html>
|
Syntax: For registering the routes
Route::request_type('/url', 'ControllerName@functionName');
Note: Here ControllerName is the name of the controller and functionName is the name of the function to be used when a user visits that URL. Let’s follow this syntax and write our route in routes/web.php at the end of the file:
Program 4: Here you can see that I have written mycontroller as my controller and index as the name of the function to be attached to this url. Now let’s visit the /viewindex2 and see the output.
php
Route::get( '/viewindex2' , 'mycontroller@index' );
|
Output:
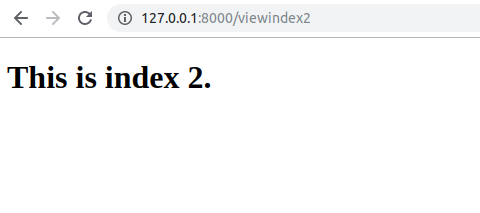
Share your thoughts in the comments
Please Login to comment...