Label in C#
Last Updated :
23 Jul, 2019
In Windows Forms, Label control is used to display text on the form and it does not take part in user input or in mouse or keyboard events. The Label is a class and it is defined under System.Windows.Forms namespace. In windows form, you can create Label in two different ways:
1. Design-Time: It is the easiest method to create a Label control using the following steps:
2. Run-Time: It is a little bit trickier than the above method. In this method, you can set create your own Label control using the Label class. Steps to create a dynamic label:
- Step 1: Create a label using the Label() constructor is provided by the Label class.
// Creating label using Label class
Label mylab = new Label();
- Step 2: After creating Label, set the properties of the Label provided by the Label class.
// Set the text in Label
mylab.Text = "GeeksforGeeks";
// Set the location of the Label
mylab.Location = new Point(222, 90);
// Set the AutoSize property of the Label control
mylab.AutoSize = true;
// Set the font of the content present in the Label Control
mylab.Font = new Font("Calibri", 18);
// Set the foreground color of the Label control
mylab.ForeColor = Color.Green;
// Set the padding in the Label control
mylab.Padding = new Padding(6);
- Step 3: And last add this Label control to form using Add() method.
// Add this label to the form
this.Controls.Add(mylab);
Example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp18 {
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e)
{
Label mylab = new Label();
mylab.Text = "GeeksforGeeks" ;
mylab.Location = new Point(222, 90);
mylab.AutoSize = true ;
mylab.Font = new Font( "Calibri" , 18);
mylab.ForeColor = Color.Green;
mylab.Padding = new Padding(6);
this .Controls.Add(mylab);
}
}
}
|
Output:
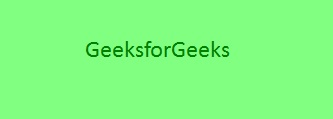
Important Properties of the Label Control
Property |
Description |
AutoSize |
This property is used to set a value indicating whether the Label control is automatically resized to display its entire contents. |
BackColor |
This property is used to set the background color for the Label control. |
BackgroundImage |
This property is used to set the background image for the Label control. |
BorderStyle |
This property is used to set the border style for the Label control. |
FlatStyle |
This property is used to set the flat style appearance of the label control. |
Font |
This property is used to set the font of the text displayed by the Label control. |
FontHeight |
This property is used to set the height of the font of the Label control. |
ForeColor |
This property is used to set the foreground color of the Label control. |
Height |
This property is used to set the height of the Label control. |
Image |
This property is used to set the image that is displayed on a Label. |
Location |
This property is used to set the coordinates of the upper-left corner of the Label control relative to the upper-left corner of its form. |
Name |
This property is used to set the name of the Label control. |
Padding |
This property is used to set padding within the Label control. |
Size |
This property is used to set the height and width of the Label control. |
Text |
This property is used to set the text associated with this Label control. |
TextAlign |
This property is used to set the alignment of text in the label. |
Visible |
This property is used to set a value indicating whether the control and all its child controls are displayed. |
Width |
This property is used to set the width of the Label control. |
Share your thoughts in the comments
Please Login to comment...