Java AWT Label
Last Updated :
19 Sep, 2023
Abstract Window Toolkit (AWT) was Java’s first GUI framework, and it has been part of Java since version 1.0. It contains numerous classes and methods that allow you to create windows and simple controls. Controls are components that allow a user to interact with your application in various ways.
The AWT supports the following types of controls:
- Labels
- Push buttons
- Checkboxes
- Choice lists
- Lists
- Scroll bars
- Text Editing
The easiest control to use is a label. A Label is an object of type Label (class in java.awt.Component), and it contains a string, which it displays within a container. Labels are passive controls that do not support any interaction with the user. To create a label, we need to create the object of the Label class.
What is Label in Java AWT?
Label in Java AWT is a component for placing text or images in a container. The text displayed in the Container (Display) by Java AWT Label can’t be edited directly by the user but needs the programmer to change it from his side.
Note: Java AWT Label is also called passive control as no event is created when it is accessed.
Syntax of Java AWT Label
public class Label extends Component implements Accessible
Java AWT Label Class Constructors
There are three types of Java AWT Label Class
1. Label():
Creates Empty Label.
2. Label(String str):
Constructs a Label with str as its name.
3. Label(String str, int x):
Constructs a label with the specified string and x as the specified alignment
Java AWT Label Fields
Java AWT Labels have 3 fields there can also be determined as the java.awt.Component class fields. All of them are mentioned below:
static int LEFT
|
It specifies that the label is aligned left.
|
static int RIGHT
|
It specifies that the label is aligned right.
|
static int CENTER
|
It specifies that the label is aligned center.
|
Java AWT Label Class Methods
There are a few predefined Methods associated with Java AWT Label as mentioned below:
1.
|
void setText(String text) |
Sets the text of the label. |
2.
|
String getText() |
Gets the text of the label. |
3.
|
void setAlignment(int alignment) |
Sets the alignment of the text in the label. Possible values: Label.LEFT , Label.RIGHT , Label.CENTER . |
4.
|
int getAlignment() |
Gets the alignment of the text in the label. |
5.
|
void addNotify() |
Creates the peer for the label. |
6.
|
AccessibleContext getAccessibleContext() |
Gets the AccessibleContext associated with the label. |
7.
|
void addNotify()
|
Creates the peer (native window) for the label.
|
Methods inherited
The Methods provided with AWT Label is inherited by classes mentioned below:
- java.awt.Component
- java.lang.Object
Java AWT Label Examples
There are certain cases associated with Java AWT Label so let us explore them as examples below:
Example 1: ( Basic Printing Hello World! )
Java
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
class Main {
public static void main(String[] args)
{
Frame frame = new Frame( "Basic" );
Label label = new Label( "Hello World!" );
frame.add(label);
frame.setSize( 500 , 500 );
frame.setVisible( true );
frame.addWindowListener( new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e)
{
System.exit( 0 );
}
});
}
}
|
Compiling and Executing the Program
javac Main.java
java Main
Output:
-300.webp)
Example 2: (Printing Center Aligned Text)
In this example we will check how to change the alignment of the Label we are putting into the frame.
Below is the implementation of the Example:
Java
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
class Main {
public static void main(String[] args)
{
Frame frame = new Frame( "Basic" );
Label label = new Label( "Change is Here" );
label.setAlignment(Label.CENTER);
frame.add(label);
frame.setSize( 500 , 500 );
frame.setVisible( true );
frame.addWindowListener( new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e)
{
System.exit( 0 );
}
});
}
}
|
Compiling and Executing the Program
javac Example2.java
java Example2
Output:
-(1)-300.webp)
Example 3: ( ActionListener )
In this example , we are creating objects of Label and Button classes which are added to the Frame. Using actionPerformed() method an event is generated over the button. When we click the button we will get the result if a number is odd or even.
Below is the implementation of the above method:
Java
import java.awt.*;
import java.awt.event.*;
public class Example3
extends Frame implements ActionListener {
private Frame frame;
private Label label;
private Button button;
public int count = 0 ;
public Example3()
{
frame = new Frame( "Example 3 of AWT Label" );
label
= new Label( "Check if a Number is Even or Odd" );
button = new Button( "Calculate" );
button.addActionListener( this );
frame.add(label, BorderLayout.CENTER);
frame.add(button, BorderLayout.SOUTH);
frame.setSize( 300 , 300 );
frame.setVisible( true );
frame.addWindowListener( new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e)
{
System.exit( 0 );
}
});
}
@Override public void actionPerformed(ActionEvent e)
{
if (e.getSource() == button) {
String str;
if (count % 2 == 0 )
str = count + " is Even" ;
else
str = count + " is odd" ;
label.setText(str);
count++;
}
}
public static void main(String[] args)
{
Example3 example = new Example3();
}
}
|
Compiling and Executing the Program
javac Example3.java
java Example3
Output Before Any Click:
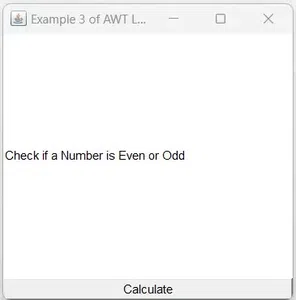
Output After First Click:
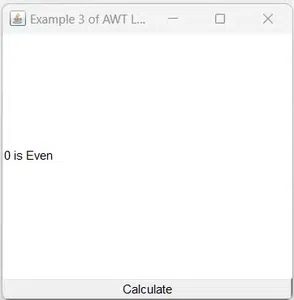
Output After Second Click:
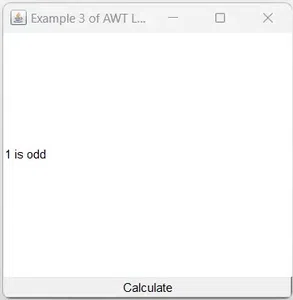
Conclusion
We hope you learned something new from this article on the Java AWT Label class. As a Java developer, you’ll find this Label class to be a very useful component. It is simple to use, but it can be customized to meet the needs of any application. Experiment with the Label class and see how you can use it to make your user interfaces more informative and engaging.
Java AWT Label – FAQs
1. What is a Java AWT Label?
A Java AWT Label is a component that is used to display a single line of read-only text. It is a passive control, which means that it does not generate any events when it is accessed.
2. What are the features of the Java AWT Label class?
The Java AWT Label class has the following features:
- It can display a single line of text.
- The text can be changed by the programmer.
- The text can be aligned to the left, right, or center.
- The text can be set to a variety of fonts and colors.
- The label can be given a border or background.
3. What are the methods of the Java AWT Label class?
The Java AWT Label class has the following methods:
- void setText(String text): This method sets the text of the label.
- String getText(): This method gets the text of the label.
- void setAlignment(int alignment): This method sets the alignment of the text in the label. The possible values for alignment are Label.LEFT, Label.RIGHT, and Label.CENTER.
- int getAlignment():This method gets the alignment of the text in the label.
- void setFont(Font font): This method sets the font of the text in the label.
- protected String paramString(): This Returns a string representing the state of this Label.
- Void addNotify(): Creates the peer (native window) for the label.
4. How to create a Java AWT Label?
To create a Java AWT Label, you can use the following code:
Label label = new Label(“This is a label”);
Share your thoughts in the comments
Please Login to comment...