JS 2018 – ECMAScript 2018
Last Updated :
24 Aug, 2023
JavaScript 2018 (ES9) or ECMAScript 2018 is a modified version of ES8, in which ES9 introduced new features like asynchronous iteration, rest/spread properties, and enhancements to regular expressions, further improving asynchronous programming, object manipulation, and string handling capabilities.
JavaScript 2018 (ES9) or ECMAScript 2018 new features are:
- Asynchronous Iteration
- Promise Finally
- Object Rest Properties
- New RegExp Features
- SharedArrayBuffer
We will explore all the above methods along with their basic implementation with the help of examples.
Method 1: Asynchronous Iteration
Asynchronous iteration allows looping over asynchronous data streams using for-await-of, we can use await keyword in for/of loop.
Syntax:
for await (variable of asynchronousIterable) {
// Code to be executed for each iteration
};
Example: In this example, we use async/await to iterate through myArray, calling doSomethingAsync() for each item. It waits for asynchronous tasks to complete before moving to the next iteration
Javascript
const myArray = [1, 2, 3, 4, 5];
async function doSomethingAsync(item) {
return new Promise(resolve => {
setTimeout(() => {
console.log(item);
resolve();
}, Math.random() * 1000);
});
}
async function main() {
for (const item of myArray) {
await doSomethingAsync(item);
}
}
main();
|
Output:
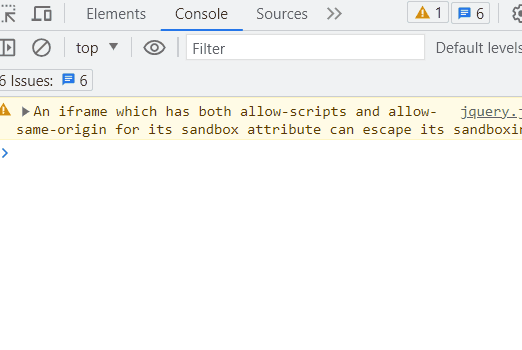
Promise.finally() executes a callback when a promise settles, either resolved or rejected, allowing cleanup operations regardless of the promise outcome.
Syntax:
task.finally(function() {
// Task to be performed when
// the promise is settled
});
Example: In this example we are using the above-explained approach.
Javascript
let task = new Promise((resolve, reject) => {
setTimeout(() => {
reject( "Promise has been rejected!" );
}, 2000);
});
task
.then(
(data) => {
console.log(data);
},
(error) => {
console.log( "Error:" , error);
}
)
.finally(() => {
console.log(
"This is finally() block that is " +
"executed after Promise is settled"
);
});
|
Output:
Error: Promise has been rejected!
This is finally() block that is executed after Promise is settled
Method 3: Object Rest Properties
Object Rest Properties allow creating new objects with selected properties, simplifying object destructuring and recombination in JavaScript.
Syntax:
{ var1, var2, ...rest } = person;
Example: In this example we are restructuring our object.
Javascript
const person = {
firstName: 'Rohan' ,
lastName: 'Nanda' ,
age: 23,
city: 'Noida'
};
let { firstName, lastName, ...rest } = person;
console.log(firstName);
console.log(lastName);
console.log(rest);
|
Output
Rohan
Nanda
{ age: 23, city: 'Noida' }
Method 4: New RegExp Features
- Unicode Property Escapes (\p{…}) : matches characters based on Unicode properties or categories in regular expressions.
- Lookbehind Assertions (?<= ) and (?<! ): matches if preceded by pattern, (?<!…) matches if not preceded, enhancing regex flexibility.
- Named Capture Groups: Named Capture Groups assign names to regex captures for organized data extraction and manipulation.
- s (dotAll) Flag: to match newline characters, improving regex pattern matching across lines.
Syntax:
const regex = /\p{Property}/gu; // Unicode Property Escapes
const regex = /(?<=prefix)pattern/; //Lookbehind Assertions
const regex = /(?<groupName>pattern)/; //Named Capture Groups
const regex = /pattern/s; //s (dotAll) Flag
Example: In this example we are using the above-explained methods.
Javascript
const text = 'GeeksforGeeks' ;
const letterRegex = /\p{Letter}+/gu;
console.log(text.match(letterRegex));
const positiveLookbehind = /(?<=Geeks) for /;
console.log(text.match(positiveLookbehind));
const negativeLookbehind = /(?<!Geeks) for /;
console.log(text.match(negativeLookbehind));
const dotAllRegex = /G.+s/s;
console.log(text.match(dotAllRegex));
const regex = /(?<year>\d{4})-(?<month>\d{2})-(?<day>\d{2})/;
const match = regex.exec( '2023-08-14' );
console.log(match.groups.year);
console.log(match.groups.month);
console.log(match.groups.day);
|
Output
[ 'GeeksforGeeks' ]
[ 'for', index: 5, input: 'GeeksforGeeks', groups: undefined ]
null
[
'GeeksforGeeks',
index: 0,
input: 'GeeksforGeeks',
groups: undefined
]
2023
08
14
Method 5: SharedArrayBuffer
SharedArrayBuffer is a JavaScript feature that enables multiple threads to access and manipulate shared memory, aiding concurrent operations, but it requires synchronization to prevent race conditions.
Syntax:
const sharedBuffer = new SharedArrayBuffer(byteLength);
Example: In this example, a SharedArrayBuffer is created with a size of 4 bytes, and an Int32Array view is established over it. The value 42 is assigned to the shared array.
Javascript
const sharedBuffer = new SharedArrayBuffer(4);
const sharedArray = new Int32Array(sharedBuffer);
sharedArray[0] = 42;
console.log(sharedArray[0]);
|
Supported browser:
- Chrome 1
- Edge 12
- Firefox 1
- Safari 1
- Opera 3
Share your thoughts in the comments
Please Login to comment...