jQuery Events
Last Updated :
16 Oct, 2023
jQuery events are actions or occurrences that happen on a web page, such as clicks, hover, or keypress. jQuery provides methods to handle and respond to these events with ease. jQuery events are used to create dynamic web pages.
Syntax:
$(selector).method(function)
Here We will explore some basic events along with their basic implementation of examples.
jQuery click() Event
jQuery click is a mouse event that triggers when an element is clicked by the mouse pointer.
Syntax:
$(selector).click(function);
Example: In this example, we are using the click() event.
HTML
<!DOCTYPE html>
< html >
< head >
< script src =
</ script >
< script >
$(document).ready(function () {
$("p").click();
});
</ script >
< style >
p {
display: block;
width: 300px;
padding: 20px;
font-size: 30px;
border: 2px solid green;
}
</ style >
</ head >
< body >
< center >
< p onclick = "alert('paragraph was clicked')" >
Geeksforgeeks.
</ p >
</ center >
</ body >
</ html >
|
Output
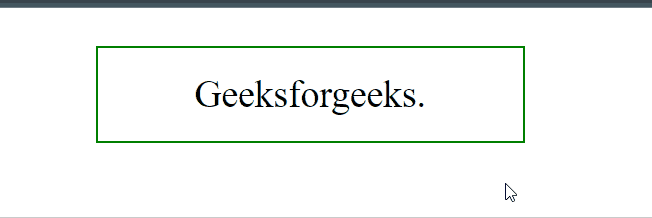
jQuery dblclick() Event
jQuery dblclick is a mouse event that triggers when an element is double-clicked by the mouse pointer.
Syntax:
$(selector).dblclick(args);
Example: In this example, we are using dblclick() event.
HTML
<!DOCTYPE html>
< html >
< head >
< script src =
</ script >
< script >
$(document).ready(function () {
$("p").dblclick();
});
</ script >
< style >
p {
display: block;
width: 300px;
padding: 20px;
font-size: 30px;
border: 2px solid green;
}
</ style >
</ head >
< body >
< center >
< p onclick = "alert('dblclick event has trigged')" >
Geeksforgeeks.
</ p >
</ center >
</ body >
</ html >
|
Output
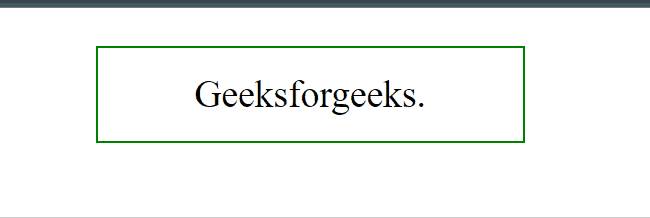
jQuery mouseenter() and mouseleave() Events
jQuery mouseenter is a mouse event that triggers when the mouse pointer enters an element, and mouseleave is a mouse event that triggers when the mouse pointer leaves an element.
Syntax:
$(selector).mouseenter(function)
and
$(selector).mouseleave(function)
Example: In this example, we have a div with a class “box.” It changes color to white on mouseenter and black on mouseleave using jQuery.
HTML
<!DOCTYPE html>
< html >
< head >
< script src =
</ script >
< style >
.box {
width: 200px;
height: 100px;
background-color: lightblue;
}
.box:hover {
background-color: gray;
}
</ style >
</ head >
< body >
< h2 >
Mouse Enter and Mouse Leave Events Example
</ h2 >
< div class = "box" >
Hover over the div
</ div >
< script >
$(document).ready(function () {
// Using the mouseenter event
$(".box").mouseenter(function () {
$(this).css("color", "white");
});
// Using the mouseleave event
$(".box").mouseleave(function () {
$(this).css("color", "black");
});
});
</ script >
</ body >
</ html >
|
Output
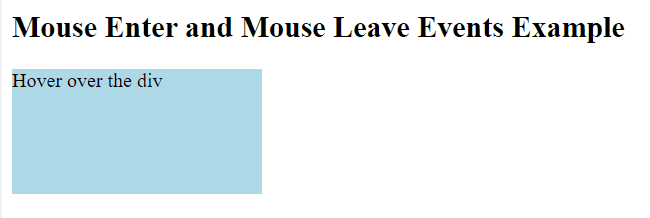
The Complete List of jQuery events are listed below:
jQuery Mouse events
jQuery Mouse events handle interactions with the mouse, like click, hover, dblclick. Use on() method to bind event handlers.
click() |
The jQuery click() is an inbuilt method that starts the click event or attaches a function to run when a click event occurs. |
dblclick() |
The jQuery dblclick() is an inbuilt method that is used to trigger the double-click event to occur. |
moueenter() |
This is an inbuilt method that works when the mouse pointer moves over the selected element. |
mouseleave() |
This is an inbuilt method that works when the mouse pointer leaves the selected element. |
mouseup() |
Occurs when any mouse button is released on an element. |
contextmenu() |
Occurs when the right mouse button is clicked on an element, opening the context menu. |
mouseover() |
The event occurs when the pointer is moved onto an element, or onto one of its children. |
mouseout() |
The event occurs when a user moves the mouse pointer out of an element, or out of one of its children. |
jQuery Keyboard Events
jQuery Keyboard events handle interactions with the keyboard, like keypress, keyup, keydown. Use on() method to bind event handlers.
keypress() |
The jQuery keypress() method triggers the keypress event whenever the browser registers a keyboard input. |
keydown() |
This is used to trigger the key-down event whenever the User presses a key on the keyboard. |
keyup() |
This is used to trigger the keyup event whenever the User releases a key from the keyboard. |
jQuery Document/ Window Events
jQuery Document/Window events handle interactions with the document or window.
load() |
load is a document event that triggers when the entire page and its resources have loaded. |
resize() |
resize is a window event that triggers when the window is resized. |
scroll() |
The jQuery scroll() is an inbuilt method that is used to user scroll in the specified element. |
unload() |
The unload is a window event that triggers when the page unloads. |
jQuery Form Event
jQuery Form event handles form-related interactions like submit etc. Use on() method to bind event handlers to form elements.
Form submission event triggered when a user submits a form on a web page. |
Event-triggered when an element’s value changes (e.g., input, select). |
Event fired when an element gains focus (e.g., input, textarea). |
The event is fired when an element loses focus. |
Share your thoughts in the comments
Please Login to comment...