Job Selection Problem – Loss Minimization Strategy | Set 2
Last Updated :
20 Dec, 2022
We have discussed one loss minimization strategy before: Job Sequencing Problem – Loss Minimization. In this article, we will look at another strategy that applies to a slightly different problem.
We are given a sequence of N goods of production numbered from 1 to N. Each good has a volume denoted by (Vi). The constraint is that once a good has been completed its volume starts decaying at a fixed percentage (P) per day. All goods decay at the same rate and further each good takes one day to complete.
We are required to find the order in which the goods should be produced so that the overall volume of goods is maximized.
Example 1:
Input: 4, 2, 151, 15, 1, 52, 12 and P = 10%
Output: 222.503
Solution: In the optimum sequence of jobs, the total volume of goods left at the end of all jobs is 222.503
Example 2:
Input: 3, 1, 41, 52, 15, 4, 1, 63, 12 and P = 20%
Output: 145.742
Solution: In the optimum sequence of jobs the total volume of goods left at the end of all jobs is 145.72
Explanation:
Since this is an optimization problem, we can try to solve this problem by using a greedy algorithm. On each day we make a selection from among the goods that are yet to be produced. Thus, all we need is a local selection criterion or heuristic, which when applied to select the jobs will give us the optimum result.
Instead of trying to maximize the volume, we can also try to minimize the losses. Since the total volume that can be obtained from all goods is also constant, if we minimize the losses we are guaranteed to get the optimum answer.
Now consider any good having volume V
Loss after Day 1: PV
Loss after Day 2: PV + P(1-P)V or V(2P-P^2)
Loss after Day 3: V(2P-P^2) + P(1 – 2P + P^2)V or V(3P – 3P^2 + P^3)
As the day increases the losses to increase. So the trick would be to ensure that the goods are not kept idle after production. Further, since we are required to produce at least one job per day, we should perform low-volume jobs, and then perform high-volume jobs.
This strategy works due to two factors.
- High Volume goods are not kept idle after production.
- As the volume decreases the loss per day too decreases, so for low-volume goods, the losses become negligible after a few days.
So in order to obtain the optimum solution we produce the larger volume goods later on. For the first day select the good with the least volume and produce it. Remove the produced good from the list of goods. For the next day repeat the same. Keep repeating while there are goods left to be produced.
When calculating the total volume at the end of production, keep in mind the good produced on day i, will have
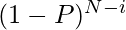
times its volume left. Evidently, the good produced on day N (last day) will have its volume intact since

Algorithm:
Step 1: Add all the goods to a min-heap
Step 2: Repeat following steps while Queue is not empty
Extract the good at the head of the heap
Print the good
Remove the good from the heap
[END OF LOOP]
Step 4: End
Below is the implementation of the solution.
C++
#include <bits/stdc++.h>
using namespace std;
void optimum_sequence_jobs(vector< int >& V, double P)
{
int j = 1, N = V.size() - 1;
double result = 0;
priority_queue< int , vector< int >, greater< int > > Queue;
for ( int i = 1; i <= N; i++)
Queue.push(V[i]);
while (!Queue.empty()) {
cout << Queue.top() << " " ;
V[j++] = Queue.top();
Queue.pop();
}
for ( int i = N; i >= 1; i--)
result += pow ((1 - P), N - i) * V[i];
cout << endl << result << endl;
}
int main()
{
vector< int > V{ -1, 3, 5, 4, 1, 2, 7, 6, 8, 9, 10 };
double P = 0.10;
optimum_sequence_jobs(V, P);
return 0;
}
|
Java
import java.util.*;
class GFG{
static void optimum_sequence_jobs( int [] V,
double P)
{
int j = 1 , N = V.length - 1 ;
double result = 0 ;
PriorityQueue<Integer> Queue =
new PriorityQueue<>();
for ( int i = 1 ; i <= N; i++)
Queue.add(V[i]);
while (!Queue.isEmpty())
{
System.out.print(Queue.peek() +
" " );
V[j++] = Queue.peek();
Queue.remove();
}
for ( int i = N; i >= 1 ; i--)
result += Math.pow(( 1 - P),
N - i) * V[i];
System.out.printf( "\n%.2f\n" ,
result );
}
public static void main(String[] args)
{
int [] V = {- 1 , 3 , 5 , 4 , 1 ,
2 , 7 , 6 , 8 , 9 , 10 };
double P = 0.10 ;
optimum_sequence_jobs(V, P);
}
}
|
Python3
from heapq import heappop, heappush, heapify
def optimum_sequence_jobs(V: list , P: float ):
N = len (V) - 1
j = 1
result = 0
Queue = []
for i in V[ 1 :]:
heappush(Queue, i)
while Queue:
top = heappop(Queue)
V[j] = top
print (top, end = " " )
j + = 1
print ()
for i in range (N, 0 , - 1 ):
result + = V[i] * pow (( 1 - P), (N - i))
print (f "{result:.4f}" )
if __name__ = = "__main__" :
V = [ - 1 , 3 , 5 , 4 , 1 , 2 , 7 , 6 , 8 , 9 , 10 ]
P = 0.10
optimum_sequence_jobs(V, P)
|
C#
using System;
using System.Collections.Generic;
public class GFG{
static void optimum_sequence_jobs( int [] V,
double P)
{
int j = 1, N = V.Length - 1;
double result = 0;
List< int > Queue =
new List< int >();
for ( int i = 1; i <= N; i++)
Queue.Add(V[i]);
Queue.Sort();
while (Queue.Count!=0)
{
Console.Write(Queue[0] +
" " );
V[j++] = Queue[0];
Queue.RemoveAt(0);
}
for ( int i = N; i >= 1; i--)
result += Math.Pow((1 - P),
N - i) * V[i];
Console.Write( "\n{0:F2}\n" ,
result );
}
public static void Main(String[] args)
{
int [] V = {-1, 3, 5, 4, 1,
2, 7, 6, 8, 9, 10};
double P = 0.10;
optimum_sequence_jobs(V, P);
}
}
|
Javascript
<script>
function sorter(a, b){
return a - b;
}
function optimum_sequence_jobs(V,P){
var j = 1, N = V.length - 1;
var result = 0;
var Queue = [];
for ( var i=1;i<=N;i++){
Queue[i]=V[i];
}
Queue.sort(sorter);
for ( var i = 0; i < Queue.length - 1; i++){
document.write(Queue[i]+ " " );
V[j]=Queue[i];
j++;
}
for ( var i = N; i >= 1; i--){
result += ((Math.pow((1 - P),(N - i))) * V[i]);
}
document.write( "\n" );
document.write(result.toFixed(4));
}
let V = [-1, 3, 5, 4, 1, 2, 7, 6, 8, 9, 10];
var P = 0.10;
optimum_sequence_jobs(V, P);
</script>
|
Output1 2 3 4 5 6 7 8 9 10
41.3811
Time Complexity: O(N log N)
Auxiliary Space: O(N)
Share your thoughts in the comments
Please Login to comment...