Loss when two items are sold at same price and same percentage profit/loss
Last Updated :
09 Jun, 2022
Given the Selling price i.e ‘SP’ of the two items each. One item is sold at ‘P%’ Profit and other at ‘P%’ Loss. The task is to find out the overall Loss.
Examples:
Input: SP = 2400, P = 30%
Output: Loss = 474.725
Input: SP = 5000, P = 10%
Output: Loss = 101.01
Approach:
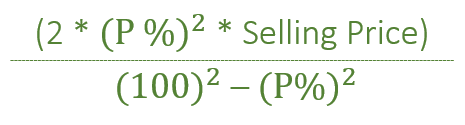
How does the above formula work?
For profit making item :
With selling price (100 + P), we get P profit.
With selling price SP, we get SP * (P/(100 + P)) profit
For loss making item :
With selling price (100 – P), we get P loss.
With selling price SP, we get SP * (P/(100 – P)) loss
Net Loss = Total Loss – Total Profit
= SP * (P/(100 – P)) – SP * (P/(100 + P))
= (SP * P * P * 2) / (100*100 – P*P)
Note: The above formula is applicable only when the Cost price of both the items are different. If CP of both the items are same then, in that case, there is ‘No profit No loss’.
Below is the implementation of the above approach
C++
#include <bits/stdc++.h>
using namespace std;
void Loss( int SP, int P)
{
float loss = 0;
loss = (2 * P * P * SP) / float (100 * 100 - P * P);
cout << "Loss = " << loss;
}
int main()
{
int SP = 2400, P = 30;
Loss(SP, P);
return 0;
}
|
Java
class GFG
{
static void Loss( int SP, int P)
{
float loss = 0 ;
loss = ( float )( 2 * P * P * SP) / ( 100 * 100 - P * P);
System.out.println( "Loss = " + loss);
}
public static void main(String[] args)
{
int SP = 2400 , P = 30 ;
Loss(SP, P);
}
}
|
Python3
def Loss(SP, P):
loss = 0
loss = (( 2 * P * P * SP) /
( 100 * 100 - P * P))
print ( "Loss =" , round (loss, 3 ))
if __name__ = = "__main__" :
SP, P = 2400 , 30
Loss(SP, P)
|
C#
class GFG
{
static void Loss( int SP, int P)
{
double loss = 0;
loss = ( double )(2 * P * P * SP) / (100 * 100 - P * P);
System.Console.WriteLine( "Loss = " +
System.Math.Round(loss,3));
}
static void Main()
{
int SP = 2400, P = 30;
Loss(SP, P);
}
}
|
PHP
<?php
function Loss( $SP , $P )
{
$loss = 0;
$loss = ((2 * $P * $P * $SP ) /
(100 * 100 - $P * $P ));
print ( "Loss = " . round ( $loss , 3));
}
$SP = 2400;
$P = 30;
Loss( $SP , $P );
?>
|
Javascript
<script>
function Loss(SP , P) {
var loss = 0;
loss = (2 * P * P * SP) / (100 * 100 - P * P);
document.write( "Loss = " + loss.toFixed(3));
}
var SP = 2400, P = 30;
Loss(SP, P);
</script>
|
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...