JavaScript Program to Find Maximum Number of Pieces in N Cuts
Last Updated :
31 Oct, 2023
We have given a square piece and the total number of cuts available (n), we need to write a JavaScript program to print the maximum number of pieces of equal size that can be obtained with the n cuts. Horizontal and Vertical cuts are allowed. We have given the example for better understanding.
Example:
Input : n = 7
Output : 20
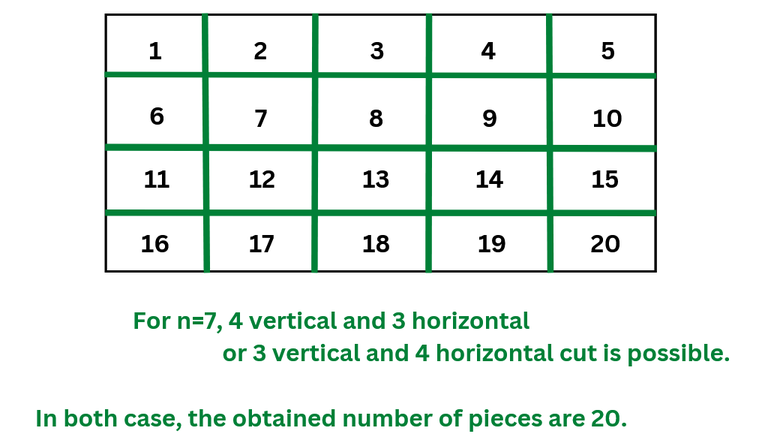
So, let’s see each of the approaches with its implementation.
Approach 1: Using parseInt() Method
In this approach, we are using the parseInt() method. Here the input is divided by 2 and using the parseInt() method it is assured that it is been treated as an Integer value, then we apply the the formula to get the maximum number of pieces.
Syntax:
parseInt(string, radix);
Example: In this example, we will be printing the Maximum number of pieces in N cuts using the parseInt() method.
Javascript
let input = 7;
let temp = parseInt(input / 2);
let result = ((temp + 1) * (input - temp + 1));
console.log(
"Max pieces for n = " + input + " is " + result);
|
Output
Max pieces for n = 7 is 20
In this approach, we are using the bitwise operation of the right shift. Here, we have to use the bitwise right shift (>>) and simple arithmetic operations to find the maximum number of pieces in N cuts.
Syntax:
operand1 >> operand2
Example: In this example, we will be printing the Maximum number of pieces in N cuts using bitwise operations.
Javascript
let input = 7;
let hCuts = input >> 1;
let vCuts = input - hCuts;
let result = (hCuts + 1) * (vCuts + 1);
console.log(
"Max pieces for n = " + input + " is " + result);
|
Output
Max pieces for n = 7 is 20
Approach 3: Using Looping
In this approach, looping is used to iterate over the input value and make alternating horizontal and vertical cuts by updating the values and calculating the maximum number of pieces.
Syntax:
for (statement 1; statement 2; statement 3) {
code here...
}
Example: In this example, we will be printing the Maximum number of pieces in N cuts using looping.
Javascript
let input = 7;
let hCuts = 0;
let vCuts = 0;
let result = 1;
for (let i = 0; i < input; i++) {
if (i % 2 === 0) {
hCuts++;
} else {
vCuts++;
}
result = (hCuts + 1) * (vCuts + 1);
}
console.log(
"Max pieces for n = " + input + " is " + result);
|
Output
Max pieces for n = 7 is 20
In this approach, we are using the recursion concept where the recursive function alternates between making the the horizontal and vertical cuts by having the track of the remaining cuts. this continues till the input reaches 0, at which point it returns the total number of pieces.
Syntax:
function functionName(parameters) {
// code here.
return functionName(modifiedParameters);
}
Example: In this example, we will be printing the Maximum number of pieces in N cuts using a recursive function.
Javascript
function recursiveFunction(input, hCuts = true ) {
if (input === 0) {
return 1;
}
if (hCuts) {
return recursiveFunction(input - 1, false ) + input;
} else {
return recursiveFunction(input - 1, true ) + 1;
}
}
let input = 7;
let result = recursiveFunction(input, true );
console.log(
"Max pieces for n = " + input + " is " + result);
|
Output
Max pieces for n = 7 is 20
Share your thoughts in the comments
Please Login to comment...