How to Open a Link in a New Window Using Applet?
Last Updated :
13 Nov, 2023
A Java applet is a little application created in the Java programming language and run on a web browser while embedded in an HTML page. In essence, it’s a method for introducing Java’s “write once, run anywhere” feature to the world of web browsers.
Components and Organization
- Applets are subclasses of the ‘java.applet.Applet’ class.
- For their graphical user interface, they can use either the AWT (Abstract Window Toolkit) or Swing frameworks, however, AWT has a smaller environmental impact and was previously more popular.
Applet’s Life Cycle
- Once the applet has loaded, the init() method is called. used to serve as an initialization.
- When the applet is relaunched and after init(), start() is called. utilized to initiate or continue applet execution.
- To redraft the applet, call paint(Graphics g). The content of the applet is shown there.
- When the applet is no longer visible or active, the stop() method is called.
- When the applet is stopped, such as by closing the browser or leaving the page, the destruct() method is called.
Displaying and Loading
The applet> tag, which is no longer supported, was used to embed applets into HTML pages. This tag defined the class, size, and other details of the applet. Later, to accomplish the same task, the <object> and <embed> tags were introduced.
Security
A security sandbox environment keeps applets from carrying out dangerous actions on the client’s computer while they are running. The user’s filesystem, network access, and other actions are restricted in this sandboxed environment. Applets had to be digitally signed in order to perform additional functions outside of the sandbox, and users had to give express consent.
What’s the Use of Applets?
Prior to the popularity of dynamic JavaScript-driven pages, they introduced dynamic and interactive information to static web pages.
- Portability: Because Java is platform-independent, applets provide a mechanism to embed functionality in web pages that function consistently across different systems.
- Rich User Interface: Compared to the constrained HTML and early JavaScript capabilities of the time, applets may offer a richer UI by utilizing Java’s GUI capabilities.
- Real-time Updates: Before AJAX gained popularity, real-time updates could be facilitated using applets without requiring a page refresh.
- Complex Computations: Because applets are Java programs, they may carry out more complex computations on the client side.
How to open a link in a new window using Applet?
Tool Requirements for Preparation: VS Code and Java 8
Establishing the Project Workspace:
- Initiate Visual Studio Code.
- Make a new directory with the name of your choice. We’ll call it “Appletdemo” for the purposes of this example.
- Create a new directory inside “Appletdemo.” Again, you choose the name, but we’ll stick with “appletdemo” to maintain consistency.
File Creation
- Create the following files in the “appletdemo” directory: “my_policy.txt,” “OpenWebPageApplet.html,” and “OpenWebPageApplet.java.”
- Note: You are free to select different names, but make sure to modify the project’s commands before running it.
Keep in mind that naming will be important during the project’s execution phase.
Summary of the project directory
Your project hierarchy ought to look like
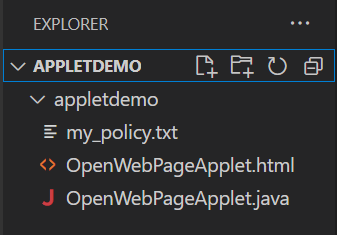
Let’s use Applet to produce the essential files needed to open a link in a new window.
- The’my_policy.txt’ file gives the Java applet particular access. Applets’ capabilities are limited by default for security reasons. We use the’my_policy.txt’ file to specifically grant an applet the required permissions if it needs to do anything that isn’t allowed by those default constraints (like accessing a web page).
The ‘my_policy.txt’ file will look like this:
HTML
grant {
permission java.security.AllPermission;
permission java.awt.AWTPermission "showWindowWithoutWarningBanner";
};
|
OpenWebPageApplet.html
The browser scans the applet> element and recognizes that it needs to launch a Java applet when you load this HTML in a browser (that supports Java applets, with the relevant configurations in place). The “OpenWebPageApplet.class” file, which contains the bytecode for the applet, will then be searched for by the browser. This bytecode will be executed by the Java Virtual Machine (JVM), and the applet will operate in the area enclosed by the width and height (in this case, 800×300 pixels). When the applet starts, the init() method is invoked, which configures the event listeners and user interface components (such as buttons and text fields).
The final OpenWebPageApplet.html file will look like this:
HTML
<!DOCTYPE html>
< html >
< head >
< title >Open a link using Applet</ title >
</ head >
< body >
< applet code = "OpenWebPageApplet.class" width = "800" height = "300" ></ applet >
</ body >
</ html >
|
OpenWebPageApplet.java
Step 1 : Initialization
- The ‘init()’ method is called when the applet loads. It initializes the user interface elements in this procedure.
- It constructs a “GO” button, a text field with a pre-filled value, and ties the “GO” button to an action listener.
Java
public void init()
{
btngo = new Button( "GO" );
add(btngo);
txturl = new TextField( "Enter domain like 'google'" , 20 );
add(txturl);
btngo.addActionListener( this );
}
|
Step 2 : Button Click Event Handling
- The ‘actionPerformed()’ method is called when the user presses the “GO” button. When the “GO” button is detected as the action event’s source, this method opens the URL.
- It retrieves the user’s input from the text field inside the ‘actionPerformed()’ method.
- If “.com” is not already present, it is appended to the input.
- Builds a ‘URL’ object from the user’s input.
- Opens the URL in the system’s default web browser after determining whether surfing is supported by the system using the ‘Desktop’ class.
Java
public void actionPerformed(ActionEvent ae) {
if (ae.getSource() == btngo) {
try {
String userInput = txturl.getText().trim();
if (!userInput.endsWith( ".com" )) {
userInput += ".com" ;
}
URL url = new URL( "http://" + userInput);
if (Desktop.isDesktopSupported() && Desktop.getDesktop().isSupported(Desktop.Action.BROWSE)) {
Desktop.getDesktop().browse(url.toURI());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
|
Step 3: Handling errors
The code is enclosed in a try-catch block to deal with potential errors, such as problems with the system’s default browser or invalid URLs.
When this applet is included in an HTML page, a straightforward user interface with a text field and a button is produced. The “GO” button attempts to launch the specified domain in the user’s default web browser when the user types in a domain name (such as “google”) and clicks it. In the absence of “.com,” the applet adds it to the domain name before attempting to open the URL. The URL is opened in the browser using the ‘Desktop’ class, and any problems are handled correctly thanks to exception handling.
The final OpenWebPageApplet.java file will look like this:
Java
import java.applet.Applet;
import java.awt.Button;
import java.awt.Desktop;
import java.awt.TextField;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.net.URL;
public class OpenWebPageApplet extends Applet implements ActionListener {
Button btngo;
TextField txturl;
public void init() {
btngo = new Button( "GO" );
add(btngo);
txturl = new TextField( "Enter domain like 'google'" , 20 );
add(txturl);
btngo.addActionListener( this );
}
public void actionPerformed(ActionEvent ae) {
if (ae.getSource() == btngo) {
try {
String userInput = txturl.getText().trim();
if (!userInput.endsWith( ".com" )) {
userInput += ".com" ;
}
URL url = new URL( "http://" + userInput);
if (Desktop.isDesktopSupported() && Desktop.getDesktop().isSupported(Desktop.Action.BROWSE)) {
Desktop.getDesktop().browse(url.toURI());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
|
Running the Applet in Visual Studio Code with Java 8
- Java 8 is required in order for our applet to function properly. Verify that Java 8 is the chosen runtime for your project in Visual Studio Code before continuing.
- In Visual Studio Code, select “Java Project”.
- Click the “ellipsis” or “more options” symbol, which is represented by three vertical dots.
- Click the drop-down menu and select “Configure Java Runtime.”
- Select ‘Configure Java Runtime’ from the following screen and select the location where Java 8 is installed. Choose the relevant folder.
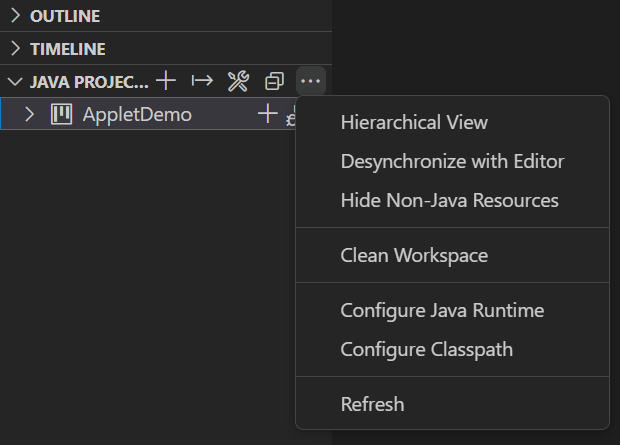
To set up the correct runtime, click on ‘Configure Java Runtime’ and then navigate to and select the directory where Java 8 is installed.
Compilation
With the correct runtime set up, the next step is compiling our Java file to generate the necessary class file. Open a terminal within Visual Studio Code.
Run the following command to compile the `OpenWebPageApplet.java` file using the Java 8 compiler:
& "C:\Program Files\Java\jdk1.8.0_202\bin\javac" appletdemo\OpenWebPageApplet.java
Once the command executes successfully, you’ll find a `OpenWebPageApplet.class` file generated in your directory.
Navigating to the Applet Directory:
Move to the `appletdemo` directory by running the following command in the terminal:
cd appletdemo
Running the Applet:
With everything set up and our Java file compiled, it’s time to run our applet and see it in action.
Use the following command to run `OpenWebPageApplet.html` using the Java applet viewer:
& "C:\Program Files\Java\jdk1.8.0_202\bin\appletviewer" -J"-Djava.security.policy=my_policy.txt" OpenWebPageApplet.html
Video Demo of the Program
Post successful execution, an interface will appear where you can enter the desired domain. Once you input the domain and trigger the action, the specified website will launch in a new browser window.
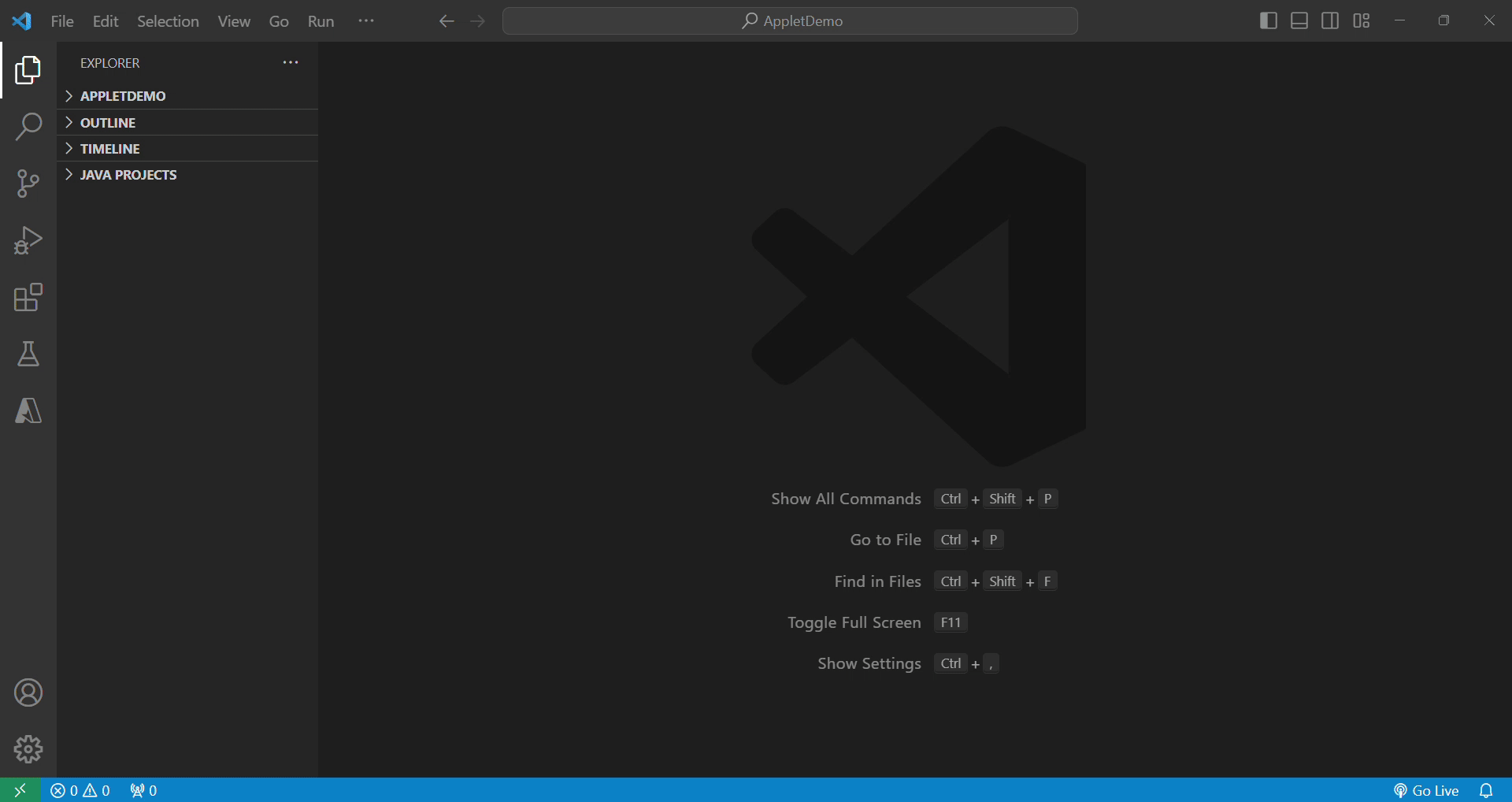
Frequently Asked Questions
1. Exactly what is a Java applet?
A Java applet is a tiny Java application that is designed to be inserted in an HTML page by a web browser. It takes advantage of Java’s promise to enable “write once, run anywhere,” ensuring interoperability with different operating systems and web browsers.
2. How are Applets Organized and Structured?
‘java.applet.Applet’ is the class from which applets are derived. Although they can use both Swing and the AWT (Abstract Window Toolkit) for their GUI, the AWT has historically been more popular because of its lower overhead.
3. How Does an Applet’s Lifecycle Operate?
Several stages make into an applet’s lifecycle:
- The ‘init()’ function is used for initialization, and it is called when the applet loads.
- Starting through the’start()’ method: Activated following the ‘init()’ method or when the applet is restarted.
- Using the ‘paint(Graphics g)’ command to display content: shows the content of the applet.
- Stopping via’stop()’: Invoked when the applet stops responding.
- Applet termination via ‘destruct()’: Represents the termination of the applet, such as when the user exits the browser.
4. How Do I Embed Applets in Web Pages and What Security Consequences Do They Pose?
The “applet” tag was once utilized for embedding, however it is no longer in use. The tags “object” and “embed” are now the preferred ones. When it comes to security, applets are contained within a sandbox environment that restricts access to the file system and the internet. Applets must be digitally signed and the user must expressly consent in order to receive further permissions.
5. Why Use Applets in the Landscape of Web Development Today?
Applets gave static web pages interactive features prior to the emergence of dynamic JavaScript pages. Their portability guarantees uniformity in functionality across many systems. Before the introduction of AJAX, they also offered real-time updates and a richer user interface than conventional HTML. Additionally, because they are Java applications, applets can do sophisticated computations on the client side.
Share your thoughts in the comments
Please Login to comment...