How to Play Audio File (.WAV) Using Java Applet?
Last Updated :
23 Oct, 2023
In this article, we will learn how to read and play audio (.wav) files using Java Applet.
Approach for Playing Audio File(.wav) using Applet
MusicPlayerApplet class inherits the property of Applet in Java. The interface consists of the input text field to enter a filename with an extension of the audio (.wav) file, a button to play the audio, and another button to stop the audio.
The AudioClip interface is used to play and stop the audio. The AudioClip interface consists of two important to play and stop the audio.
On clicking the Play button or Stop button, the action listener will call a method that contains logic to play and stop the audio. If the play button is pressed, play() method is called to play the audio. If the stop button is pressed, stop() method is called to stop the audio.
Notes:
Latest java version doesn’t support applet. so, it needs older version of java which supports applet to be installed.
AudioClip interface supports only WAV (Waveform Audio File Format) file with .wav extension. Other formats are not supported.
Java version for Applet viewer
Applet viewer is no longer available from Java version 9. So, it requires Java version 8 or older to run applet viewer. Refer to the following article to install the applet-supported Java version.How to Install Java Applet Viewer in Windows?
Program to play audio file (.WAV) using Applet
Below is the implementation of the above method:
Java
import java.applet.Applet;
import java.awt.*;
import java.awt.event.*;
import java.net.URL;
import java.applet.AudioClip;
public class MusicPlayerApplet extends Applet implements ActionListener {
private Button playButton;
private Button stopButton;
private TextField filenameField;
private Label filenameLabel;
private AudioClip audioClip;
public void init() {
filenameField = new TextField( 20 );
filenameLabel = new Label( "Enter Filename: " );
playButton = new Button( "Play" );
stopButton = new Button( "Stop" );
playButton.addActionListener( this );
stopButton.addActionListener( this );
add(filenameLabel);
add(filenameField);
add(playButton);
add(stopButton);
audioClip = null ;
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == playButton) {
String filename = filenameField.getText();
if (!filename.isEmpty()) {
URL audioURL = getClass().getResource(filename);
audioClip = getAudioClip(audioURL);
audioClip.play();
}
} else if (e.getSource() == stopButton) {
if (audioClip != null ) {
audioClip.stop();
}
}
}
}
|
Executing the Program
1. Run the following command to compile the program.
javac MusicPlayerApplet.java
2. Run the following command to run the program.
appletviewer MusicPlayerApplet.java

Execute the program
Steps to Run the Program
There are certain Steps mentioned below to run the Applet Program:
- Enter the filename with .wav extension.
- Click play button to play the audio.
- Click stop button to stop the audio.
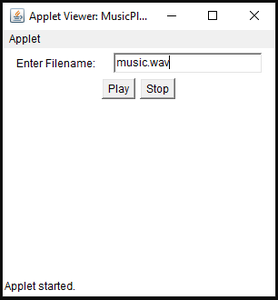
Share your thoughts in the comments
Please Login to comment...