Java Program for Phone Mnemonics
Last Updated :
03 Apr, 2024
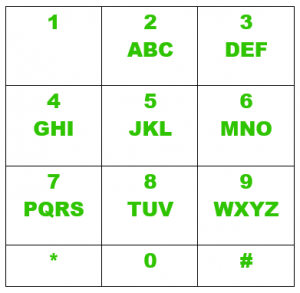
In our daily lives, we have to remember a lot of phone numbers. Most people find it quite difficult to remember such huge 10-digit phone numbers. A simple solution is to relate that phone number to some known word. For example, we can relate the phone number 32627287 with DAMASCUS based on the characters in our dial pad. (We can replace a DIGIT with any one of the corresponding CHARACTER it represents on the dial pad. For example, we have ABC mentioned under 2 in our dial pad. So we can represent 2 with either A or B or C.
Similarly we can replace 3 with D or E or F.
Write a program that will take in a phone number and print all possible such words which are in our dictionary. Let us consider the sample input 23. Here,
2 can be replaced using A or B or C.
3 can be replaced using D or E or F.
So one valid combination would be AD which corresponds to 623.
Another can be CD and so on.
We are generating all the possible permutations starting with {A or B or C} and then {D or E or F}.However, only those permutations will be printed which are in our dictionary. These selected words will represent our sample output.
However, DIGITS like 1 and 0 will be replaced by a null string as they don’t represent any character on the dial pad i.e 1 and 0 will represent “”.
Examples:
Input : 23
Output : [BE]
Input : 623
Output : [MAD, OCD]
Input : 726
Output : [PAN, PCM, PCO, RAM, SAM]
Input : 2
Output : [A, B, C]
Input : 123
Output : [BE]
Naive Approach
This program uses backtracking to generate all possible words .Click here to know more. The concept of backtracking has been explained there.
Java
import java.util.*;
public class PhoneMnemonics {
static ArrayList<String> dictionary = new ArrayList<String>();
/* List all the phone mnemonics for the given digit sequence.
* @param input a digit sequence, like "23432" */
public static ArrayList<String> listMnemonics(String input)
{
ArrayList<String> list = new ArrayList<String>();
String[] dic = { "A", "B", "C", "D", "E", "F", "G",
"H", "I", "J", "K", "L", "M", "N",
"O", "P", "Q", "R", "S", "T", "U",
"V", "W", "X", "Y", "Z", "MAD",
"OCD", "PAN", "PCM", "PCO", "RAM", "SAM", "BE" };
for (String i : dic)
dictionary.add(i);
listMnemonics("", input, list);
return list;
}
/* Helper recursive method to list phone mnemonics. This works by finding
* all possibilities for the first digit, then recursing on the rest of the
* string. For example, if prefix is "AB" and remaining is "2", then the
* mnemonics "ABA", "ABB", and "ABC" would be printed.
*
* @param prefix : the part of the mnemonic that has already been built
* @param remaining : the remaining digits to include in the mnemonic */
private static void listMnemonics(String prefix, String remaining,
ArrayList<String> list)
{
// Base case: when there are no more characters to process,
// just print out the mnemonic that we've already built.
if (remaining.isEmpty()) {
if (!list.contains(prefix) && dictionary.contains(prefix))
list.add(prefix);
return;
}
// Recursive case.
if (remaining.charAt(0) == '1' || remaining.charAt(0) == '0') {
listMnemonics(prefix, remaining.substring(1), list);
}
String digits = digitLetters(remaining.charAt(0));
for (int i = 0; i < digits.length(); i++) {
String newPrefix = prefix + digits.charAt(i);
String newRemaining = remaining.substring(1);
listMnemonics(newPrefix, newRemaining, list);
}
}
/**
* Get the letters appearing on a given key of a standard phone keypad.
* @param ch the character representation of a digit on the phone keypad
* (like '2')
* @return a string containing the letters on the given key, or the empty
* string if the key is not recognized
*/
private static String digitLetters(char ch)
{
switch (ch) {
case '2':
return "ABC";
case '3':
return "DEF";
case '4':
return "GHI";
case '5':
return "JKL";
case '6':
return "MNO";
case '7':
return "PQRS";
case '8':
return "TUV";
case '9':
return "WXYZ";
}
return "";
}
public static void main(String[] args)
{
String str = "123";
ArrayList<String> list = listMnemonics(str);
System.out.println(list);
}
}
Note: This program will become more convenient for the users once we add a dictionary of words along with their frequency of use.Using a dictionary, the following output could have been optimized to print MAD, OCD, … so on based on their frequency of use by the users. Check out this link:
Related Article with C++ implementation :
Print all possible words from phone digits
Reference :
Thinking Recursively by Eric Roberts.
Share your thoughts in the comments
Please Login to comment...