Introduction to JustPy | A Web Framework based on Python
Last Updated :
13 Oct, 2023
JustPy is a web framework that leverages the power of Python to create web applications effortlessly. In this article, we’ll explore JustPy, its features, and why it’s gaining attention among developers.
What is the JustPy Module of Python?
The JustPy module of Python is a web framework like Django but it is an oriented, Component-Based, and High-level framework with required zero Front End Programming. Users who don’t know Frontend technologies like JavaScript can easily build some interactive websites without any Frontend knowledge, just by using Python Code and not a single line of JavaScript.
In JustPy, the methods or classes which is used to create the webpage elements are known as Component Class. This Component Classes will hold the user-defined custom elements inside it and users can use them as many times as they want, without writing the same design twice. Using these component classes, we can differentiate between different designs, functionalities, etc. from the other designs or component classes so that they don’t overlap with each other.
JustPy also supports the SVG and HTML components alongside some more complex features like Charts and Grids with the help of the Matplotlib library of Python. It also supports other Python libraries like Pandas, and even with a Pandas extension which helps the user create interactive charts or grids using the Pandas Datastructures like Series and Dataframe.
Features of JustPy
- Uses Python Syntax – The JustPy module is purely based upon the Python language and it uses Pythonic syntax, which makes it easier for developers who know Python and can easily learn and use it.
- Real-Time Applications – JustPy supports real-time functionality, which means that it lets the developers create interactive and responsive web applications without the use of languages like JavaScript.
- Support Events – Developers can create Python functions and those functions will react based upon certain events, such as clicking a button.
- Supports Component-Based Architecture – As JustPy follows an object-oriented approach, all the classes or functions used act as reusable components, so that the developers can use them as many times as possible and it makes the code short and concise.
- No external support needed by default – Although, if the developers want they can manually use HTML or CSS to make the application more complex or add extra features, by default JustPy doesn’t need any of those to work, it depends solely upon Python
Advantages of JustPy
- Event Driven Programming – Just like modern JavaScript Frameworks, React and Vue.JS, it follows an Even Driven Architecture. Developers can create certain Python functions and make them react on specific events like clicking a button, hovering over something etc. The event driven approach makes it simple to handle user interactions with the Web Applications.
- Reactive Programming – JustPy supports Reactive programming, which updates the UI of the web application automatically if something has been changed in the code. No need to reload it manually, this reactive approach leads to simpler UI development by reducing the need of explicit and excessive DOM manipulation.
- No Front End Framework Needed – By default, JustPy doesn’t need any external front end technologies like JavaScript, HTML, CSS etc. JustPy can build complete web applications just by using Python. Developers’ can use HTML or CSS if needed, but it is not necessary to build web applications in JustPy.
- Built-in Chart Support – JustPy also provides a built-in chart support system, which lets the developers to create interactive charts without using any other modules.
- Cross Platform Support – As Python supports multiple platforms like Windows, MacOS, Linux etc, JustPy also supports cross-platform development.
- Rapid Prototyping – JustPy’s Pythonic syntax makes it well-suited for rapid prototyping, developers can quickly develop and test web applications without the need of external complex web developments setups.
Displaying a simple “Hello Geeks” message using JustPy
To install justpy use this command.
pip install justpy
This function is used to display something on the browser.
Python3
import justpy as jp
def hello_world_function():
web_page = jp.WebPage()
div = jp.Div(text = 'Hello Geeks' )
web_page.add(div)
return web_page
jp.justpy(hello_world_function)
|
Running the Program
python <filename>.py
The code will be deployed in 127.0.0.1:8080, i.e localhost and port number 8080.

Output
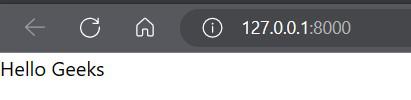
Basic Concepts related to JustPy
Components
As stated earlier, JustPy components are classes that contains specific design or code which can be resued by the user just by creating an object of it and calling it via the object. In the above example –
div = jp.Div(text='Hello Geeks')
This line is similar to the HTML version –
<div> Hello Geeks </div>
Web Pages
Web Pages are also a component of JustPy and they are instance of the WebPage class of JustPy. We need to first create an instance of it then add elements as we want.
web_page = jp.WebPage()
The variable web_page is storing the instance of the WebPage() class. Now we can add text or any other element by calling add() method.
web_page.add(div)
Finally, we need to return the object of the WebPage class, i.e in which we have instantiated the class and stored it.
Adding some Basic HTML Components
Adding a Button
We can add some responsive button in our webpage too using JustPy. The button will show some text before clicking, and that will change after we have clicked it. It is like the innerHTML concept of JavaScript DOM.
Python3
import justpy as jp
button_class = 'm-2 bg-blue-500 hover:bg-green-700 text-white font-bold py-2 px-4 rounded'
def on_clicked( self , msg):
self .text = 'Oh! Hi Geek!'
def button_event():
wp = jp.WebPage()
d = jp.Button(text = 'I don\'t know You Yet' , a = wp, classes = button_class)
d.on( 'click' , on_clicked)
return wp
jp.justpy(button_event)
|
Output
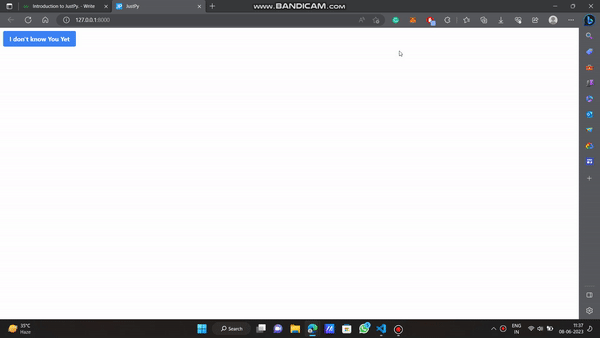
Adding Hover Reaction
Now we will use a button and add some response when the user hovers over it. We will use Three functions / Components here.
- First one will define the behaviour of the button when it is clicked.
- Second one will define the behaviour when the user is hovering over the button.
- Third one will define when the user left the button or stop hovering over it and moved other way.
As we are using three functions here with different functionalities it as another example of handling multiple events at once.
Python3
import justpy as jp
def click_fun( self , msg):
self .text = 'This button has been clicked'
self .set_class( 'bg-blue-500' )
def hover_function( self , msg):
self .text = 'The mouse pointer is hovering over'
self .set_class( 'bg-red-500' )
def hover_function_reaction( self , msg):
self .text = 'The mouse Pointer left'
self .set_class( 'bg-green-500' )
def main_hover():
wp = jp.WebPage()
d = jp.Div(text = 'Button not Clicked yet' , a = wp,
classes = 'w-64 text-2xl m-2 p-2 bg-blue-500 text-white rounded' ,
click = click_fun, mouseenter = hover_function,
mouseleave = hover_function_reaction)
return wp
jp.justpy(main_hover)
|
Output
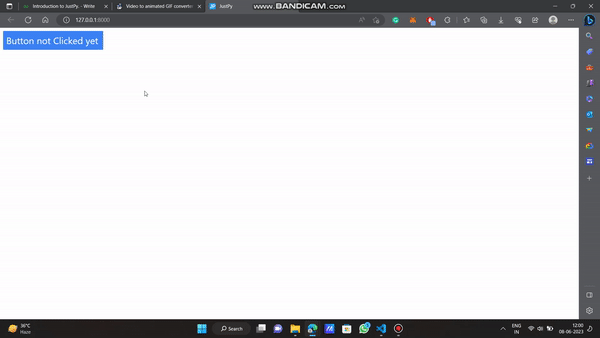
Adding Italics Text
This Python script uses the JustPy library to create a web page displaying the text “GeeksforGeeks in Italic” in an italic format. It defines a function ‘italic_text()’ that sets up the web page and adds the italic text. When executed, it runs as a JustPy web application, serving the page with the formatted text.
Python3
import justpy as jp
def italic_text():
wp = jp.WebPage()
jp.I(text = 'GeeksforGeeks in Italic' , a = wp)
return wp
jp.justpy(italic_text)
|
Output
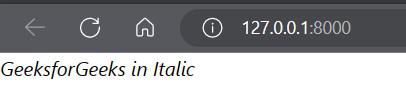
Adding a Line Break
This script uses the JustPy library to create a web page with italicized text and a line break. It imports JustPy as jp, defines a function (line_break_demo), and adds text elements to the page. It then returns the web page object. When executed, it serves this web page.
Python3
import justpy as jp
def line_break_demo():
wp = jp.WebPage()
jp.I(text = 'GeeksforGeeks in Italic' , a = wp)
jp.Br(a = wp)
jp.P(text = "Text after line Break" , a = wp)
return wp
jp.justpy(line_break_demo)
|
Output
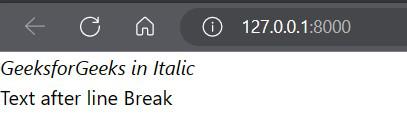
Adding a Bold Text
This script uses the JustPy library to create a web page with italicized text, a line break, and bold text. It imports JustPy as jp, defines a function (bold_text), and adds text elements to the page. It returns the web page object. When executed, it serves this web page.
Python3
import justpy as jp
def bold_text():
wp = jp.WebPage()
jp.I(text = 'GeeksforGeeks in Italic' , a = wp)
jp.Br(a = wp)
jp.Strong(text = "Bold Text after line Break" , a = wp)
return wp
jp.justpy(bold_text)
|
Output
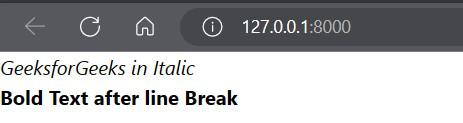
HTML Links (Anchor Tag)
This script uses the JustPy library to create a web page with italicized text, a line break, bold text, and a hyperlink. It imports JustPy as jp, defines a function (bold_text), and adds various text elements to the page, including a hyperlink to “Best Place to learn DSA” with a link to GeeksforGeeks. It returns the web page object. When executed, it serves this web page.
Python3
import justpy as jp
def bold_text():
wp = jp.WebPage()
jp.I(text = 'GeeksforGeeks in Italic' , a = wp)
jp.Br(a = wp)
jp.P(text = "Bold Text after line Break" , a = wp)
jp.Br(a = wp)
a = wp, classes = 'm-2 p-2 text-xl text-white bg-green-500 hover:bg-green-700' )
return wp
jp.justpy(bold_text)
|
Output
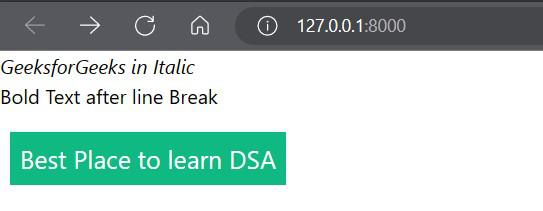
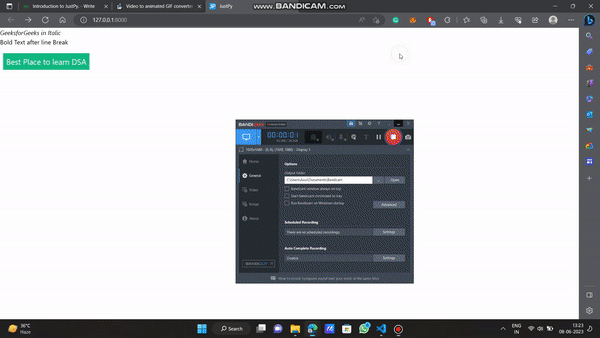
Share your thoughts in the comments
Please Login to comment...