Intersecting rectangle when bottom-left and top-right corners of two rectangles are given
Last Updated :
23 May, 2022
Given coordinates of 4 points, bottom-left and top-right corners of two rectangles. The task is to find the coordinates of the intersecting rectangle formed by the given two rectangles.
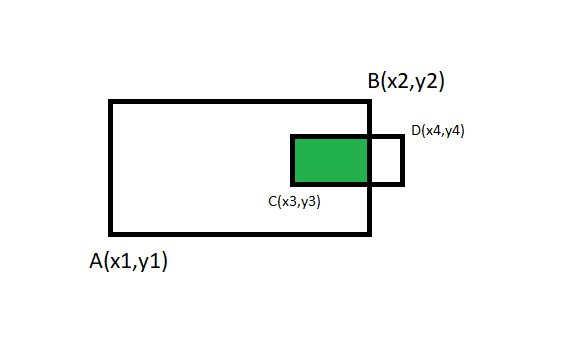
Examples:
Input:
rec1: bottom-left(0, 0), top-right(10, 8),
rec2: bottom-left(2, 3), top-right(7, 9)
Output: (2, 3) (7, 8) (2, 8) (7, 3)
Input:
rec1: bottom-left(0, 0), top-right(3, 3),
rec2: bottom-left(1, 1), top-right(2, 2)
Output: (1, 1) (2, 2) (1, 2) (2, 1)
Approach :
As two given points are diagonals of a rectangle. so, x1 < x2, y1 < y2. similarly x3 < x4, y3 < y4.
so, bottom-left and top-right points of intersection rectangle can be found by using formula.
x5 = max(x1, x3);
y5 = max(y1, y3);
x6 = min(x2, x4);
y6 = min(y2, y4);
In case of no intersection, x5 and y5 will always exceed x6 and y5 respectively. The other two points of the rectangle can be found by using simple geometry.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void FindPoints( int x1, int y1, int x2, int y2,
int x3, int y3, int x4, int y4)
{
int x5 = max(x1, x3);
int y5 = max(y1, y3);
int x6 = min(x2, x4);
int y6 = min(y2, y4);
if (x5 > x6 || y5 > y6) {
cout << "No intersection" ;
return ;
}
cout << "(" << x5 << ", " << y5 << ") " ;
cout << "(" << x6 << ", " << y6 << ") " ;
int x7 = x5;
int y7 = y6;
cout << "(" << x7 << ", " << y7 << ") " ;
int x8 = x6;
int y8 = y5;
cout << "(" << x8 << ", " << y8 << ") " ;
}
int main()
{
int x1 = 0, y1 = 0, x2 = 10, y2 = 8;
int x3 = 2, y3 = 3, x4 = 7, y4 = 9;
FindPoints(x1, y1, x2, y2, x3, y3, x4, y4);
return 0;
}
|
C
#include <stdio.h>
int min( int a, int b)
{
int min = a;
if (min > b)
min = b;
return min;
}
int max( int a, int b)
{
int max = a;
if (max < b)
max = b;
return max;
}
void FindPoints( int x1, int y1, int x2, int y2, int x3, int y3, int x4, int y4)
{
int x5 = max(x1, x3);
int y5 = max(y1, y3);
int x6 = min(x2, x4);
int y6 = min(y2, y4);
if (x5 > x6 || y5 > y6) {
printf ( "No intersection" );
return ;
}
printf ( "(%d, %d) " ,x5,y5);
printf ( "(%d, %d) " ,x6,y6);
int x7 = x5;
int y7 = y6;
printf ( "(%d, %d) " ,x7,y7);
int x8 = x6;
int y8 = y5;
printf ( "(%d, %d) " ,x8,y8);
}
int main()
{
int x1 = 0, y1 = 0, x2 = 10, y2 = 8;
int x3 = 2, y3 = 3, x4 = 7, y4 = 9;
FindPoints(x1, y1, x2, y2, x3, y3, x4, y4);
return 0;
}
|
Java
class GFG
{
static void FindPoints( int x1, int y1,
int x2, int y2,
int x3, int y3,
int x4, int y4)
{
int x5 = Math.max(x1, x3);
int y5 = Math.max(y1, y3);
int x6 = Math.min(x2, x4);
int y6 = Math.min(y2, y4);
if (x5 > x6 || y5 > y6)
{
System.out.println( "No intersection" );
return ;
}
System.out.print( "(" + x5 + ", " +
y5 + ") " );
System.out.print( "(" + x6 + ", " +
y6 + ") " );
int x7 = x5;
int y7 = y6;
System.out.print( "(" + x7 + ", " +
y7 + ") " );
int x8 = x6;
int y8 = y5;
System.out.print( "(" + x8 + ", " +
y8 + ") " );
}
public static void main(String args[])
{
int x1 = 0 , y1 = 0 ,
x2 = 10 , y2 = 8 ;
int x3 = 2 , y3 = 3 ,
x4 = 7 , y4 = 9 ;
FindPoints(x1, y1, x2, y2,
x3, y3, x4, y4);
}
}
|
Python3
def FindPoints(x1, y1, x2, y2,
x3, y3, x4, y4):
x5 = max (x1, x3)
y5 = max (y1, y3)
x6 = min (x2, x4)
y6 = min (y2, y4)
if (x5 > x6 or y5 > y6) :
print ( "No intersection" )
return
print ( "(" , x5, ", " , y5, ") " , end = " " )
print ( "(" , x6, ", " , y6, ") " , end = " " )
x7 = x5
y7 = y6
print ( "(" , x7, ", " , y7, ") " , end = " " )
x8 = x6
y8 = y5
print ( "(" , x8, ", " , y8, ") " )
if __name__ = = "__main__" :
x1 = 0
y1 = 0
x2 = 10
y2 = 8
x3 = 2
y3 = 3
x4 = 7
y4 = 9
FindPoints(x1, y1, x2, y2, x3, y3, x4, y4)
|
C#
using System;
class GFG
{
static void FindPoints( int x1, int y1,
int x2, int y2,
int x3, int y3,
int x4, int y4)
{
int x5 = Math.Max(x1, x3);
int y5 = Math.Max(y1, y3);
int x6 = Math.Min(x2, x4);
int y6 = Math.Min(y2, y4);
if (x5 > x6 || y5 > y6)
{
Console.WriteLine( "No intersection" );
return ;
}
Console.Write( "(" + x5 + ", " +
y5 + ") " );
Console.Write( "(" + x6 + ", " +
y6 + ") " );
int x7 = x5;
int y7 = y6;
Console.Write( "(" + x7 + ", " +
y7 + ") " );
int x8 = x6;
int y8 = y5;
Console.Write( "(" + x8 + ", " +
y8 + ") " );
}
public static void Main()
{
int x1 = 0, y1 = 0,
x2 = 10, y2 = 8;
int x3 = 2, y3 = 3,
x4 = 7, y4 = 9;
FindPoints(x1, y1, x2, y2,
x3, y3, x4, y4);
}
}
|
PHP
<?php
function FindPoints( $x1 , $y1 , $x2 , $y2 ,
$x3 , $y3 , $x4 , $y4 )
{
$x5 = max( $x1 , $x3 );
$y5 = max( $y1 , $y3 );
$x6 = min( $x2 , $x4 );
$y6 = min( $y2 , $y4 );
if ( $x5 > $x6 || $y5 > $y6 )
{
echo "No intersection" ;
return ;
}
echo "(" . $x5 . ", " . $y5 . ") " ;
echo "(" . $x6 . ", " . $y6 . ") " ;
$x7 = $x5 ;
$y7 = $y6 ;
echo "(" . $x7 . ", " . $y7 . ") " ;
$x8 = $x6 ;
$y8 = $y5 ;
echo "(" . $x8 . ", " . $y8 . ") " ;
}
$x1 = 0; $y1 = 0; $x2 = 10; $y2 = 8;
$x3 = 2; $y3 = 3; $x4 = 7; $y4 = 9;
FindPoints( $x1 , $y1 , $x2 , $y2 ,
$x3 , $y3 , $x4 , $y4 );
?>
|
Javascript
<script>
function FindPoints(x1, y1, x2, y2,
x3, y3, x4, y4)
{
var x5 = Math.max(x1, x3);
var y5 = Math.max(y1, y3);
var x6 = Math.min(x2, x4);
var y6 = Math.min(y2, y4);
if (x5 > x6 || y5 > y6)
{
document.write( "No intersection" );
return ;
}
document.write( "(" + x5 + ", " +
y5 + ") " );
document.write( "(" + x6 + ", " +
y6 + ") " );
var x7 = x5;
var y7 = y6;
document.write( "(" + x7 + ", " +
y7 + ") " );
var x8 = x6;
var y8 = y5;
document.write( "(" + x8 + ", " +
y8 + ") " );
}
var x1 = 0, y1 = 0,
x2 = 10, y2 = 8;
var x3 = 2, y3 = 3,
x4 = 7, y4 = 9;
FindPoints(x1, y1, x2, y2,
x3, y3, x4, y4);
</script>
|
Output:
(2, 3) (7, 8) (2, 8) (7, 3)
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...