Insert and delete at the beginning in Doubly Linked List in Python
Last Updated :
10 Dec, 2023
A doubly linked list is a collection of nodes. It is an advanced version of a singly linked list. In a doubly linked list, a node has three elements its data, a link to the next node, and a link to its previous node. In this article, we will learn how to insert a node at the beginning and delete a node from the beginning of the doubly linked list.
Creating a Node class for a Linked List in Python
To implement the Node class in Python we create a class “Node” in which we have implemented an init function to initialize the node of a doubly linked list. The “init” function takes data as an argument and if we do not pass any argument while creating an instance of this class it initializes all the variables as “None”. Here, next stores the reference to the next node, prev stores the reference to the previous node, and data stores the data of the current node.
Python3
class Node:
def __init__( self , data = None ):
self .data = data
self . next = None
self .prev = None
|
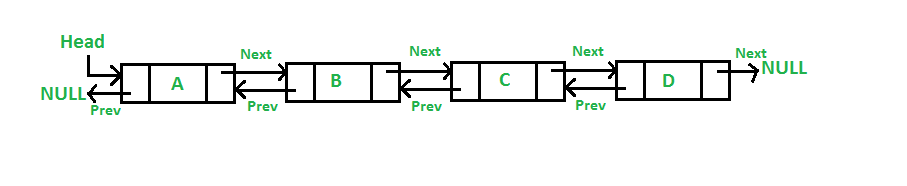
Python Program to Insert a new Node at the Beginning of DLL
To insert the a node at beginning of doubly linked list firstly, we take data as an argument from a function and then we create a new node and passed data in the new node. Now, we have two cases.
Case 1: If the head of the doubly linked list is “None”. In this case, we simply make new node as head.
Case 2: In this case we have some node in doubly linked list. Here, we make the reference to the previous node of head to new node and make head as the reference to the next of the new node and finally make the new node as head.
Python3
def insert_at_begin( self , data):
new_node = Node(data)
if self .head is None :
self .head = new_node
else :
self .head.prev = new_node
new_node. next = self .head
self .head = new_node
|
Python Program to Delete a new Node at the Beginning of DDL
When we are deleting the node there may be three cases which are when the list is empty, when there is only one element in list, and when there are more than one element in a list. Let’s see all three cases one by one.
Case 1: If the list is empty, we simply print the message that “List is empty”.
Case 2: If there is only one element in the list, it means the next of the head is “None” then we make head equal to None.
Case 3: If there are more than one nodes present in the list then to delete the node from beginning of linked list we just make second node of linked list as head and make the previous reference of that node “None”.
Python3
def delete_at_begin( self ):
if self .head is None :
print ( "List is empty" )
elif self .head. next is None :
self .head = None
else :
self .head = self .head. next
self .head.prev = None
|
Function to Print Doubly Linked List
In this function we print all the nodes of doubly linked list. To print the list firstly we check whether head is None or not. If the head is None we simply print “List is empty” and if there are nodes present in the doubly linked list first we create a new node “curr” and store the reference to the head node in it. Now, running a while loop util “curr” is not equal to “None”. Inside the while loop we print data of a node and change the reference of the “curr” to the next node.
Python3
def print_list( self ):
if self .head is None :
print ( "List is empty" )
return
curr = Node()
curr = self .head
while (curr ! = None ):
print (curr.data)
curr = curr. next
print ()
|
Complete Implementation of Doubly linked list
Below is the complete implementation of above approached explained above. In the output we can see that we are able to to insert the node at beginning and delete the node from begining.
Python3
class Node:
def __init__( self , data = None ):
self .data = data
self . next = None
self .prev = None
class DoublyLInkedList:
def __init__( self ):
self .head = None
def insert_at_begin( self , data):
new_node = Node(data)
if self .head is None :
self .head = new_node
else :
self .head.prev = new_node
new_node. next = self .head
self .head = new_node
def delete_at_begin( self ):
if self .head is None :
print ( "List is empty" )
return
elif self .head. next is None :
self .head = None
else :
self .head = self .head. next
self .head.prev = None
def print_list( self ):
if self .head is None :
print ( "List is empty" )
return
curr = Node()
curr = self .head
while (curr ! = None ):
print (curr.data)
curr = curr. next
print ()
head = DoublyLInkedList()
head.insert_at_begin( 10 )
head.insert_at_begin( 20 )
head.insert_at_begin( 30 )
print ( "List after inserting node at begining:" )
head.print_list()
head.delete_at_begin()
print ( "List after deleting node from begining:" )
head.print_list()
head.delete_at_begin()
head.delete_at_begin()
head.delete_at_begin()
|
Output:
List after inserting node at begining:
30
20
10
List after deleting node from begining:
20
10
List is empty
Advantages of Doubly Linked List
- Bidirectional traversal: In a doubly linked list we can traverse from both directions, unlike the singly linked list which is unidirectional. It is possible because a node in a linked list stores the reference of its previous and next nodes.
- Insertion and Deletion: Insertion and deletion of nodes at both the beginning and end of the list are efficient operations. We can also insert and delete nodes at any position in the list with O(1) time complexity if we have a reference to the node to be inserted/deleted.
- Reverse Linked List: We can reverse the doubly linked list very efficiently just by making the last node of the linked list the head node.
- No Need to Traverse from the Beginning: Unlike the single linked list, we do not need to traverse the doubly linked list from the beginning instead we can traverse the double linked list from any node as it has reference to its previous and next node.
Disadvantages of Doubly Linked List
- Extra Memory Usage: Doubly linked lists use more memory compared to singly linked lists because they require two pointers (next and previous) for each node instead of just one (next). This extra memory overhead can be significant in applications with limited memory resources.
- Complexity: The presence of two pointers (next and previous) in each node makes the implementation and maintenance of doubly linked lists more complex than singly linked lists. This complexity can lead to more opportunities for bugs and errors.
- Additional Overhead: Each node in a doubly linked list has extra overhead due to the two pointers. This can impact memory usage and cache performance, especially in scenarios where memory is a concern.
Share your thoughts in the comments
Please Login to comment...