Insert a node in Linked List before a given node
Last Updated :
28 Feb, 2023
Given a node of Linked List N and a value K, the task is to insert the node with value K in the linked list before the given node N.
Structure of the Node:
C++
struct Node {
int data;
Node* next;
Node( int val, Node* link = 0)
: data(val), next(link)
{
}
};
|
Java
public class Node
{
public int data;
public Node next;
public Node( int val, Node link = null )
{
this .data = val;
this .next = link;
}
}
|
Python3
class Node:
def __init__( self , val, link = None ):
self .data = val
self . next = link
|
C#
public class Node
{
public int data;
public Node next;
public Node( int val, Node link = null )
{
this .data = val;
this .next = link;
}
};
|
Javascript
<script>
class Node {
constructor(val, link = null ) {
this .data = val;
this .next = link;
}
}
</script>
|
In the given problem there might be two cases:
- Given node is the head node.
- Given node is any valid node except the head.
When given Node is the Head Node:
The idea is to create a new node with the given value K. Then the next part of the new node will be updated with the pointer head. And finally, the head will be updated with the new node’s address. Below is the image of the same:
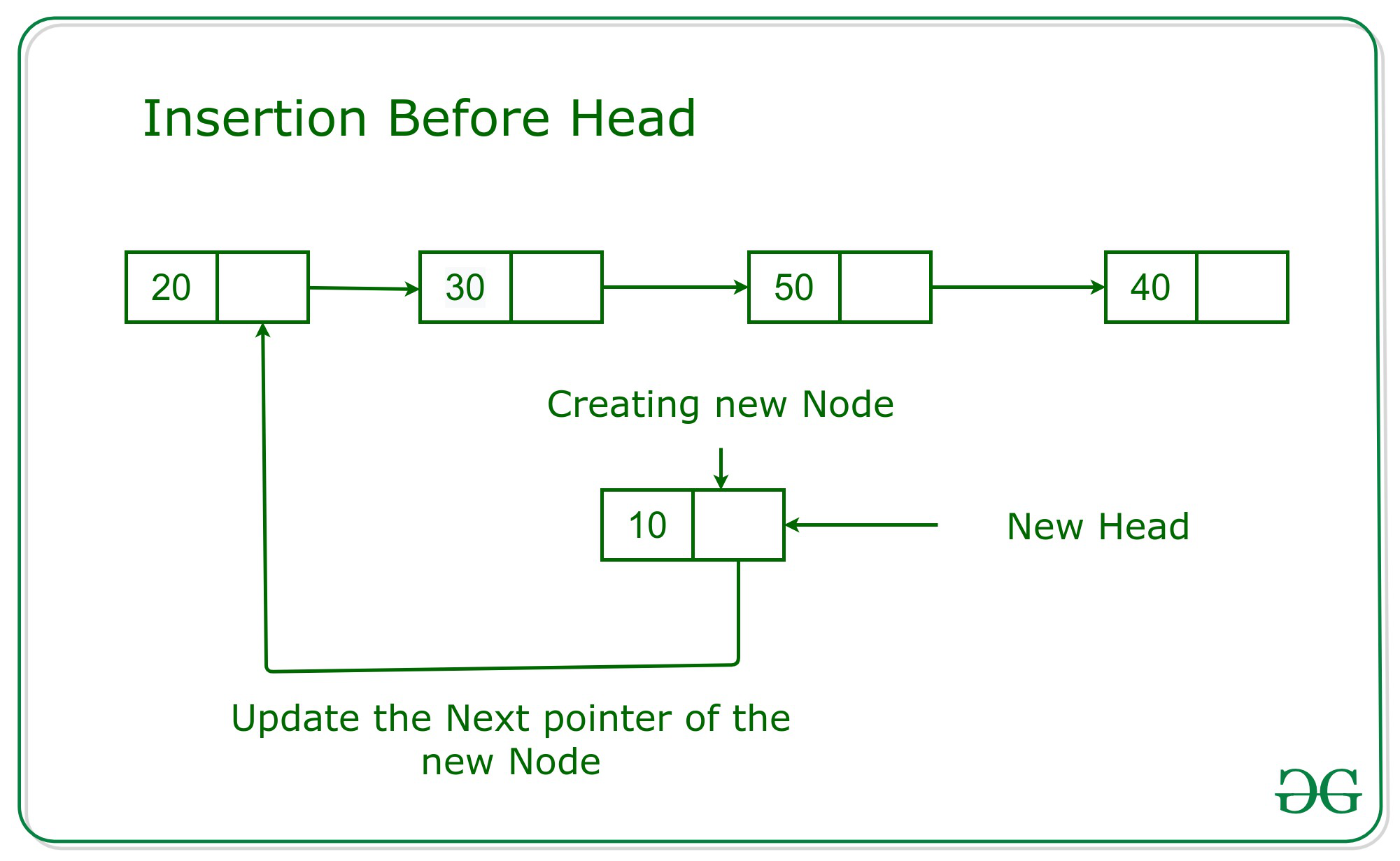
When given Node is any valid node except head node:
The simplest approach is to traverse the given linked list to search the previous node of the given node. Then, create the new node with the given value K.Now, update the next part of the new node with the address of the given node and the next part of the previous node with the address of the new node. Below is an illustration of the approach with the help of image:
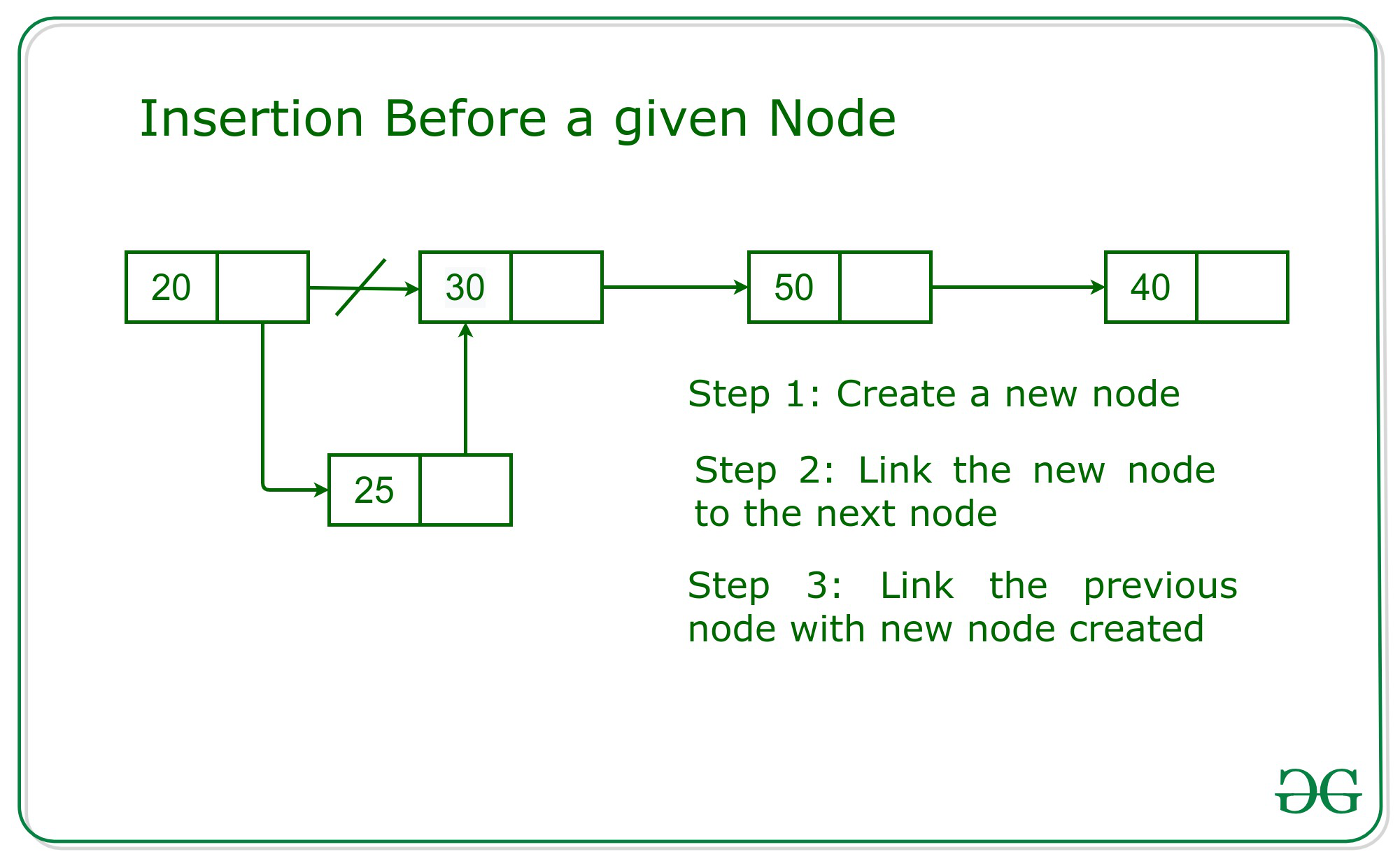
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* next;
Node( int val, Node* link = 0)
: data(val), next(link)
{
}
};
Node* head = new Node(5);
void printList(Node* n)
{
while (n != NULL) {
cout << n->data << " " ;
n = n->next;
}
}
void addBefore(Node* given_ptr, int val)
{
if (head == given_ptr) {
Node* n = new Node(val);
n->next = head;
head = n;
return ;
}
else {
Node *p, *n = head;
for (n, p; n != given_ptr;
p = n, n = n->next)
;
Node* m = new Node(val);
m->next = p->next;
p->next = m;
}
}
int main()
{
head->next = new Node(6);
addBefore(head->next, 8);
printList(head);
}
|
Java
import java.io.*;
public class GFG {
static class Node {
public int data;
public Node next;
public Node( int val) {
this .data = val;
this .next = null ;
}
}
static Node head = new Node( 5 );
static void printList(Node n) {
while (n != null ) {
System.out.print(n.data + " " );
n = n.next;
}
}
static void addBefore(Node given_ptr, int val) {
if (head == given_ptr) {
Node n = new Node(val);
n.next = head;
head = n;
return ;
}
else {
Node p = null ;
for (Node n = head; n != given_ptr; p = n, n = n.next);
Node m = new Node(val);
m.next = p.next;
p.next = m;
}
}
public static void main(String[] args) {
head.next = new Node( 6 );
addBefore(head.next, 8 );
printList(head);
}
}
|
Python3
class Node:
def __init__( self , val, link = None ):
self .data = val
self . next = link
head = Node( 5 );
def printList(n):
while (n ! = None ):
print (n.data, end = ' ' )
n = n. next ;
def addBefore(given_ptr, val):
global head
if (head = = given_ptr):
n = Node(val);
n. next = head;
head = n;
return ;
else :
p = None
n = head;
while (n ! = given_ptr):
p = n
n = n. next
m = Node(val);
m. next = p. next ;
p. next = m;
if __name__ = = '__main__' :
head. next = Node( 6 );
addBefore(head. next , 8 );
printList(head);
|
C#
using System;
class GFG{
class Node
{
public int data;
public Node next;
public Node( int val)
{
this .data = val;
this .next = null ;
}
}
static Node head = new Node(5);
static void printList(Node n)
{
while (n != null )
{
Console.Write(n.data + " " );
n = n.next;
}
}
static void addBefore(Node given_ptr,
int val)
{
if (head == given_ptr)
{
Node n = new Node(val);
n.next = head;
head = n;
return ;
}
else
{
Node p = null ;
for (Node n = head; n != given_ptr;
p = n, n = n.next);
Node m = new Node(val);
m.next = p.next;
p.next = m;
}
}
public static void Main(String[] args)
{
head.next = new Node(6);
addBefore(head.next, 8);
printList(head);
}
}
|
Javascript
<script>
class Node {
constructor(val) {
this .data = val;
this .next = null ;
}
}
var head = new Node(5);
function printList(n) {
while (n != null ) {
document.write(n.data + " " );
n = n.next;
}
}
function addBefore(given_ptr , val) {
if (head == given_ptr) {
var n = new Node(val);
n.next = head;
head = n;
return ;
}
else {
var p = null ;
for (n = head; n != given_ptr; p = n, n = n.next)
;
var m = new Node(val);
m.next = p.next;
p.next = m;
}
}
head.next = new Node(6);
addBefore(head.next, 8);
printList(head);
</script>
|
Time Complexity: O(N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...